
Professional JavaScript
Fast-track your web development career using the powerful features of advanced JavaScript
Hugo Di Francesco, Siyuan Gao, Vinicius Isola, Philip Kirkbride
- 664 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Professional JavaScript
Fast-track your web development career using the powerful features of advanced JavaScript
Hugo Di Francesco, Siyuan Gao, Vinicius Isola, Philip Kirkbride
About This Book
Develop your JavaScript programming skills by learning strategies and techniques commonly used in modern full-stack application development
Key Features
- Write and deploy full-stack applications efficiently with JavaScript
- Delve into JavaScript's multiple programming paradigms
- Get up to speed with core concepts such as modularity and functional programming to write efficient code
Book Description
In depth knowledge of JavaScript makes it easier to learn a variety of other frameworks, including React, Angular, and related tools and libraries. This book is designed to help you cover the core JavaScript concepts you need to build modern applications.
You'll start by learning how to represent an HTML document in the Document Object Model (DOM). Then, you'll combine your knowledge of the DOM and Node.js to create a web scraper for practical situations. As you read through further lessons, you'll create a Node.js-based RESTful API using the Express library for Node.js. You'll also understand how modular designs can be used for better reusability and collaboration with multiple developers on a single project. Later lessons will guide you through building unit tests, which ensure that the core functionality of your program is not affected over time. The book will also demonstrate how constructors, async/await, and events can load your applications quickly and efficiently. Finally, you'll gain useful insights into functional programming concepts such as immutability, pure functions, and higher-order functions.
By the end of this book, you'll have the skills you need to tackle any real-world JavaScript development problem using a modern JavaScript approach, both for the client and server sides.
What you will learn
- Apply the core concepts of functional programming
- Build a Node.js project that uses the Express.js library to host an API
- Create unit tests for a Node.js project to validate it
- Use the Cheerio library with Node.js to create a basic web scraper
- Develop a React interface to build processing flows
- Use callbacks as a basic way to bring control back
Who this book is for
If you want to advance from being a frontend developer to a full-stack developer and learn how Node.js can be used for hosting full-stack applications, this is an ideal book for you. After reading this book, you'll be able to write better JavaScript code and learn about the latest trends in the language. To easily grasp the concepts explained here, you should know the basic syntax of JavaScript and should've worked with popular frontend libraries such as jQuery. You should have also used JavaScript with HTML and CSS but not necessarily Node.js.
Frequently asked questions
Information
Chapter 1
JavaScript, HTML, and the DOM
Learning Objectives
- Describe the HTML Document Object Model (DOM)
- Use the Chrome DevTools source tab to explore the DOM of a web page
- Implement JavaScript to query and manipulate the DOM
- Build custom components using Shadow DOM
Introduction
HTML and the DOM
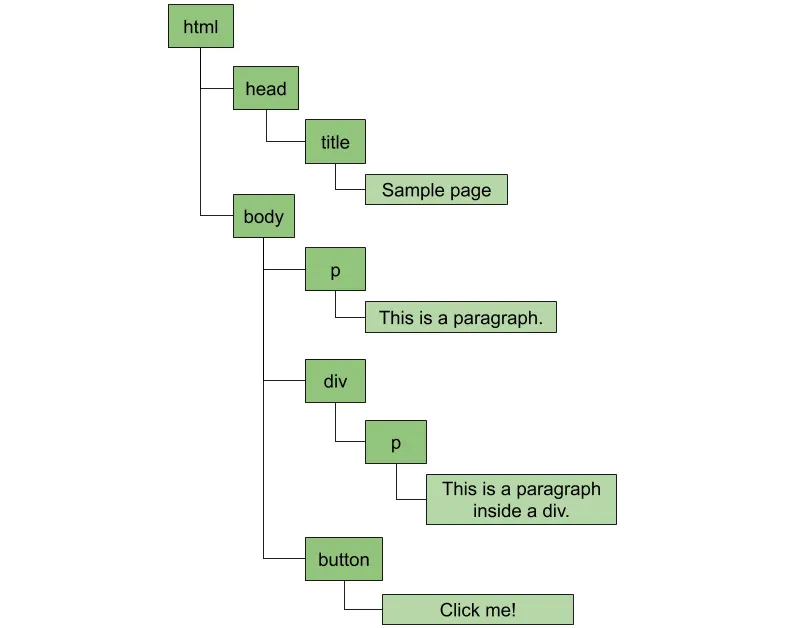
Figure 1.1: A paragraph node contains a text node
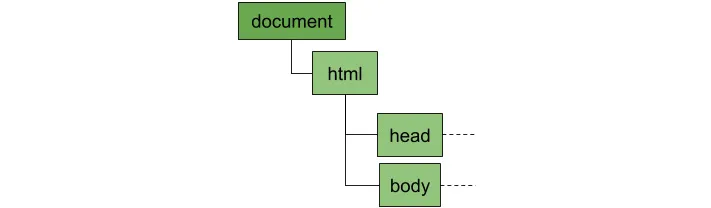
Figure 1.2: All DOM trees have a document element at the root
Exercise 1: Iterating over Nodes in a Document
- Open the text editor of your preference and create a new file called alert_paragraphs.html containing the sample HTML from the previous section (which can be found on GitHub: https://bit.ly/2maW0Sx):<html><head><title>Sample Page</title></head><body><p>This is a paragraph.</p><div><p>This is a paragraph inside a div.</p></div><button>Click me!</button></body></html>
- At the end of the body element, add a script tag such that the last few lines look like the following:</div><button>Click me!</button><script></script></body></html>
- Inside the script tag, add an event listener for the click event of the button. To do that, you query the document object for all elements with the button tag, get the first one (there's only one button on the page), then call addEventListener:document.getElementsByTagName('button')[0].addEventListener('click', () => {});
- Inside the event listener, query the document again to find all paragraph elements:const allParagraphs = document.getElementsByTagName('p');
- After that, create two variables inside the event listener to store how many paragraph elements you found and another to store their content:let allContent = "";let count = 0;
- Iterate over all paragraph elements, count them, and store their content:for (let i = 0; i < allParagraphs.length; i++) { const node = allParagraphs[i];count++;allContent += `${count} - ${node.textContent}\n`;}
- After the loop, show an alert that contains the number of paragraphs that were found and a list with all their content:alert(`Found ${count} paragraphs. Their content:\n${allContent}`);You can see how the final code should look here: https://github.com/TrainingByPackt/Professional-JavaScript/blob/master/Lesson01/Exercise01/alert_paragraphs.html.Opening the HTML document in the browser and clicking the button, you should see t...