
eBook - ePub
Parallel Programming with C# and .NET Core
Developing Multithreaded Applications Using C# and .NET Core 3.1 from Scratch
Rishabh Verma
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Parallel Programming with C# and .NET Core
Developing Multithreaded Applications Using C# and .NET Core 3.1 from Scratch
Rishabh Verma
Book details
Book preview
Table of contents
Citations
About This Book
Learn, understand, and code parallel programs with confidence using C# 8 and.NET Core 3.0 Key Features
- Explore and work with the new features and enhancements in.NET Core 3.0 & C# 8
- Understand the fundamentals of parallel programming
- Learn various threading patterns and synchronization constructs
- Build concurrent applications using C# and.Net Core 3.0 from the ground up
- Understand the principles of unit testing and debugging in concurrent applications
-
Description
Application development has evolved over the last decade, and with the advent of the latest technologies like Angular, React on client-side, and ASP.NET Core, Spring on the server-side, the consumer expectations have risen like never before. The primary objective of this book is to help readers understand the importance of asynchronous programming and various ways it can be achieved using.NET Core 3.1 and C# 8 to successfully build concurrent applications. Along the way reader will learn the fundamentals of threading, asynchronous programming, various asynchronous patterns, synchronisation constructs, unit testing parallel methods, debugging enterprise applications, and cool tips and tricks.
There are samples based on practical examples that will help the reader effectively use parallel programming. By the end of this book, you will be equipped with all the knowledge needed to understand, code, and debug multithreaded, concurrent and parallel programs with confidence. What You Will Learn
- Understand the internals of async/await.
- Learn how to build applications using async/await.
- Write unit tests for asynchronous methods.
- Explore various debugging techniques for enterprise applications.
-
Who this book is for
Beginners and intermediate developers who build enterprise applications using.NET Core platform and tools. Advanced users can also use this book for brushing up fundamentals and for learning debugging tools, techniques, tips, and tricks. Table of Contents
1. Getting Started
2. What's new in C# 8?
3..NET Core 3.1
4. Demystifying Threading
5. Parallel Programming
6. The Threading Patterns
7. Synchronization Constructs
8. Unit Testing Parallel and Asynchronous Programs
9. Debugging and Troubleshooting ( Its spelling is incorrect in pdf)
10. Tips and Tricks About the Authors
Rishabh Verma is a Microsoft certified professional and works at Microsoft as a senior development consultant, helping the customers to design, develop, and deploy enterprise-level applications. An electronic engineer by education, he has 12+ years of hardcore development experience on the.NET technology stack. He is passionate about creating tools, Visual Studio extensions, and utilities to increase developer productivity. His interests are.NET Compiler Platform (Roslyn), Visual Studio Extensibility, code generation, and.NET Core. Neha Shrivastava is a Microsoft certified professional and works as a software engineer for the Cloud & AI group at Microsoft India Development Center. She has about 10 years' development experience and has expertise in the financial, healthcare, and e-commerce domains. Neha did her bachelor's in electronics engineering. Ravindra Akella works as a Senior Consultant at Microsoft with more than 13 years of software development experience. Specializing in.NET and web-related technologies, his current role involves end to end ownership of products right from architecture to delivery.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlego’s features. The only differences are the price and subscription period: With the annual plan you’ll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, we’ve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Parallel Programming with C# and .NET Core an online PDF/ePUB?
Yes, you can access Parallel Programming with C# and .NET Core by Rishabh Verma in PDF and/or ePUB format, as well as other popular books in Informatique & Programmation parallèle. We have over one million books available in our catalogue for you to explore.
Information
Topic
InformatiqueSubtopic
Programmation parallèleCHAPTER 1
Getting Started
“The secret to getting ahead is getting started!”
- Anonymous
As the name of chapter states, we will set up the required tools and get started with our journey of parallel programming with .NET Core 3.1 and C# 8. There are essential framework and tools to be downloaded and installed to start our learning and practical implementation on .NET Core 3.1 using windows operating system. We will begin the journey with the installation of Visual Studio 2019 and the latest version of .NET Core 3.1. We will create our first .NET Core 3.1 application. Though .NET Core 3.1 is cross-platform, we will focus the discussion on Windows as that’s the most popular and widely used operating system platform on the planet. So, let’s get started.
Structure
We will cover the following topics:
- Download essential tools for Windows
- Installing Visual Studio 2019 with .NET Core 3.1
- PerfMon
- ProcMon
- Proc exe
- PerfView
- JustDecompile
- DebugDiag
- WinDbg
- Create your first .NET Core 3.1 application using Visual Studio 2019
- Summary
- Exercise
Objective
By the end of this chapter, the reader would:
- Learn to download and install all the required tools for .NET Core 3.1 and C# 8
- Create a “Hello World” application using .NET Core 3.1 template using Visual Studio 2019
- Learn to set up the development, debugging, troubleshooting and monitoring tools
Download essential tools for Windows
In this section, we will discuss the prerequisites. To have a seamless experience in learning .NET Core 3.1, we need to download and install a few developer tools. Microsoft recommends the Visual Studio Integrated Development Environment (IDE) to develop programs for Android, iOS, Windows applications, mobile applications, web applications, websites, web services, and cloud.
Navigate to the URL https://visualstudio.microsoft.com/%20downloads/ in your preferred browser. Microsoft gives us options to select from 4 Visual Studio variations:
- Community:Powerful IDE, free for students, open-source contributors, and individuals, open-source contributors, and individuals
- Professional: Professional IDE best suited to small teams
- Enterprise:Scalable, end-to-end solution for teams of any size
- Code: The fast, free and open-source code editor that adapts to your needs
We can download one of these depending on our choice and description stated above. These descriptions are taken as-is from the download site. However, depending upon the selected option, you may or may not have features described in this book, like time travel debugging, and so on. For pure development purposes and following the code snippets and samples of this book, Visual Studio 2019 Community version would suffice. The great thing is that this version is free. However, if it is possible for the reader, I would recommend Visual Studio 2019 Enterprise as it has a great set of tools and features for developing an enterprise-grade application. The authors of this book use Visual Studio 2019 Enterprise for code development and demonstration of tools.
We can go through Release Notes of each of the variants available to us to know more about them. Every variant and its small description are available on the above site:
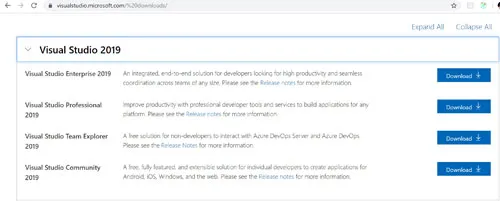
Figure 1.1: Download Visual Studio 2019
Visual Studio Code (VS Code): Apart from Community, Professional, and Enterprise variants of Visual Studio 2019, Microsoft provides a free open source code editor Visual Studio Code. It is a cross-platform code editor, and apart from Windows, VS code works with Linux and Mac OS. It’s a cross-platform editor that can be extended with extensions available based on our requirements, for example, C# extension. It includes provision for embedded Git control, debugging, syntax highlighting, snippets, intelligent code completion, extensions support, and code refactoring.
Note: Visual Studio Code, like notepad, is an editor, and Visual Studio is an IDE. So Visual Studio Code is very lightweight, fast, with great support for debugging and has embedded Git control. It is a file and folders-based editor and doesn’t need to know the project context, unlike an IDE. There is no
File | New Project
support in Visual Studio Code as we have in Visual Studio IDE. Instead, Visual Studio Code has a terminal, through which we can run .NET commands.Apart from Visual Studio 2019, we are going to use few more tools in upcoming chapters like PerfMon for performance monitoring and troubleshooting, ProcMon tool for process monitoring, PerfView for performance analysis, and many more. We will see them in action in Chapter 9, Debugging and Troubleshooting.
Installing Visual Studio 2019 with .NET Core 3.1
For coding in Windows, as we discussed above, we can use:
- Visual Studio 2019 IDE
- Visual Studio Code editor
If we choose Visual Studio 2019, we just need to download Visual Studio 2019 version 16.4 or higher from https://visualstudio.microsoft.com/vs/. Visual Studio 2019 version 16.4 is the latest at the time of writing this chapter. It may change by the time; this book gets published. It comes with .NET Core 3.1 SDK and its project templates. So, we will be ready for development immediately after installing it.
Here I am installing Visual Studio Enterprise 2019. In
workloads
section, select .NET Core cross-platform development
: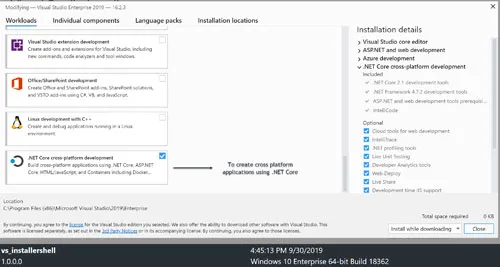
Figure 1.2: Select .NET Core cross-platform development workload
You can select additional workloads based on your needs. For this book, we need .
NET
Core cross-platform development
, so we selected it and performed the installation. With this workload selected, the rest of the steps are straight forward, and the installation can be done without any issues.Next, we will investigate other tools that we shall be using during this book. Let’s start with the tool that comes installed by default in Windows Enterprise or Pro version of the operating system.
Perfmon
- Perfmon, which is an acronym for performance monitoring, is the Windows reliability and performance monitoring tool. It helps us to troubleshoot the issues, including application level to the hardware level. To open perfmon, go to
Run
(press Windows key + R), type perfmon, and then click theOK
button:Figure 1.3: Open perfmon through Run or by ...