
eBook - ePub
Data Structures and Algorithms Implementation Through C
Let's Learn and Apply
Brijesh Bakariya
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Data Structures and Algorithms Implementation Through C
Let's Learn and Apply
Brijesh Bakariya
Book details
Book preview
Table of contents
Citations
About This Book
Book with a practical approach for understanding the basics and concepts of Data Structure Key Features
- This book is especially designed for beginners, explains all basics and concepts about data structure.
- Source code of all data structures are given in C language.
- Important data structures like Stack, Queue, Linked List, Tree and Graph are well explained.
- Solved example, frequently asked in the examinations are given which will serve as a useful reference source.
- Effective description of sorting algorithm (Quick Sort, Heap Sort, Merge Sort etc.)
Description
Book gives a full understanding of the theoretical topic and easy implementation of data structures through C. The book is going to help students in self-learning of data structures and in understanding how these concepts are implemented in programs.
Algorithms are included to clear the concept of data structure. Each algorithm is explained with figures to make student clearer about the concept. Sample data set is taken and step by step execution of the algorithm is provided in the book to ensure the in-depth knowledge of students about the concept discussed. What You Will Learn
- New features and essential of Algorithms and Arrays.
- Linked List, its type and implementation.
- Stacks and Queues
- Trees and Graphs
- Searching and Sorting
- Greedy method
- Beauty of Blockchain
-
Who this book is for
This book is specially designed to serve as textbook for the students of various streams such as PGDCA, B.Tech. /B.E., BCA, BSc M.Tech. /M.E., MCA, MS and cover all the topics of Data Structure. The subject data structure is of prime importance for the students of Computer Science and IT. It is practical approach for understanding the basics and concepts of data structure. All the concepts are implemented in C language in an easy manner. To make clarity on the topic, diagrams, examples and programs are given throughout the book Table of Contents
1. Algorithm and Flowcharts
2. Algorithm Analysi
3. Introduction to Data structure
4. Functions and Recursion
5. Arrays and Pointers
6. String
7. Stack
8. Queues
9. Linked Lists
10. Trees
11. Graphs
12. Searching
13. Sorting
14. Hashing About the Author
Dr. Brijesh Bakariya, working as an Assistant Professor in Department of Computer Science and Engineering, I.K. Gujral Punjab Technical University (IKGPTU) Jalandhar (Punjab) has his Ph. D. from Maulana Azad National Institute of Technology (NIT- Bhopal), Madhya Pradesh and MCA Degree from Devi Ahilya Vishwavidyalaya, Indore (Madhya Pradesh) in Computer Applications. He has been teaching since 2009 and guiding M.Tech/ Ph.D students. He has also published many research papers in the area of Data Mining and Image Processing.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Data Structures and Algorithms Implementation Through C an online PDF/ePUB?
Yes, you can access Data Structures and Algorithms Implementation Through C by Brijesh Bakariya in PDF and/or ePUB format, as well as other popular books in Computer Science & Programming Algorithms. We have over one million books available in our catalogue for you to explore.
Information
CHAPTER 1
Algorithms and Flowcharts
1.1 Introduction of Algorithm
First of all, before making any program or software, we have to make the documentation of it. This documentation provides the information about that entire software. This program or software can be deployed in any programming language, but if it is necessary to change something later in that program or software then its algorithm or documentation is very important.
By studying the documentation, you get complete information about the software and make some changes in it too easily. So, we can say that the understanding of the software increases through algorithms.
Let us take an example, if the software is being created for a shopping complex and its documentation is being prepared. Now, if we have to make some changes to the software according to the needs of the customer, it is very important to be aware of the outline. If the software needs to extend and we have proper documentation of it, then the software developer will not depend on the developer who develop that software.
1.2 What is an algorithm?
Let's take the problem for making a tea. For making a tea, there are following steps:
- Set a metallic saucepan on the stove top or hob.
- Add the piece of freshly crushed ginger.
- Add tea leaves in it.
- Add milk according to choice.
- Filter a solution with the help tea strainer.
- Put the filtered solution into cups.
- Serve it.
What we are doing for making a tea which is our problem. We are writing step by step procedure for solving it. Therefore, the definition of an algorithm is âIt is a step by step procedure to solve a given problem. Moreover, an algorithm accepts an input list of data and creates an output list of data.â Fig. 1.1 shows the basis concept of an algorithm.
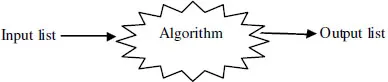
Fig 1.1: Basic structure of algorithm
We can also analysis an algorithm in terms of time and space complexity. The analysis of an algorithm has discussed in next chapter.
1.3 Flowcharts
Sometime an algorithm can be a difficult to understand. For improving an understandability of an algorithm, the developer makes a flowchart.
Suppose, if you visit a university and you have to go to a specific department then you go through a flowchart. Moreover, via map a person can easily reach to destination place. A flowchart is a pictorial of graphical representation of an algorithm.
1.3.1 Reason to Use Flowcharts
There are various reasons to use a flow chart.
- Documentation and training: Flowcharts are ideal for documentation and training purposes. Flowcharts are also very beneficial for training materials because they're visually stimulating while also being easy to understand. Ultimately, this keeps the reader's attention, so they learn faster.
- Workflow management: Flow chart is used for continuous improvement of business process all times. This decides when we meet to customer improvement.
- Programming: In the world of algorithms, you will find complexity of algorithm. Information Technology (IT) demands flowcharts for programming, and makes the process faster and smoother. Flowcharts will lead to faster, more efficient coding with fewer errors along the way.
- Troubleshooting: Multiple problems and solutions can be presented, thus makes algorithms large in size. When created properly, a flowchart for troubleshooting of the module takes less time.
- Quality assurance: Modules mapped with flowcharts ensure that regulatory requirements are followed all times. It is an essential process, and is made easier with the use of flowcharts.
- Process flow management services: It increases business efficiency through flowchart.
1.4 Advantages
- Communication: Flow Charts are better way of communicating the logic of a system.
- Effective Analysis: Problem can be effectively analysed with the help of flowchart
- Proper Documentation: Flow charts serve as a good program documentation, which is needed for various purposes.
- Efficient Coding: The flow charts act as a guide or bluep...