
eBook - ePub
GraphQL in Action
Samer Buna
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
GraphQL in Action
Samer Buna
Book details
Book preview
Table of contents
Citations
About This Book
Reduce bandwidth demands on your APIs by getting only the results you needâall in a single request! The GraphQL query language simplifies interactions with web servers, enabling smarter API queries that can hugely improve the efficiency of data requests. In GraphQL in Action, you'll learn how to bring those benefits to your own APIs, giving your clients the power to ask for exactly what they need from your server, no more, no less. Practical and example-driven, this book teaches everything you need to get started with GraphQLâfrom design principles and syntax right through to performance optimization.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is GraphQL in Action an online PDF/ePUB?
Yes, you can access GraphQL in Action by Samer Buna in PDF and/or ePUB format, as well as other popular books in Informatik & Webservices & APIs. We have over one million books available in our catalogue for you to explore.
Information
Topic
InformatikSubtopic
Webservices & APIsPart 1. Exploring GraphQL
Do you use Yelp, Shopify, Coursera, or GitHub? If so, you have consumed a GraphQL API! These are just a few of the companies that adopted GraphQL as their data communication solution.
The first part of this book answers the why, what, and how questions about GraphQL. The word GraphQL can mean different things to different people, but it is fundamentally a âlanguageâ that API consumers can use to ask for data. This part will get you comfortable with the fundamentals of that language.
In chapter 1, youâll learn what exactly GraphQL is, what problems it solves, and what problems it introduces. Youâll explore the design concepts behind it and how it is different from the alternatives, like REST APIs.
Chapter 2 explores GraphQLâs feature-rich interactive playground. This playground takes advantage of GraphQLâs introspective power, which you can use to explore what you can do with GraphQL and to write and test GraphQL requests. Youâll use this playground to explore examples of GraphQL queries and mutations. Youâll learn about the fundamental parts of a GraphQL request, and youâll test practical examples from the GitHub GraphQL API.
Chapter 3 introduces you to the many built-in features of the GraphQL language that let you customize and organize data requests and responses. Youâll learn about fields and arguments, aliases, directives, fragments, interfaces, and unions.
1 Introduction to GraphQL
This chapter covers
- Understanding GraphQL and the design concepts behind it
- How GraphQL differs from alternatives like REST APIs
- Understanding the language used by GraphQL clients and services
- Understanding the advantages and disadvantages of GraphQL
Necessity is the mother of invention. The product that inspired the creation of GraphQL was invented at Facebook because the company needed to solve many technical issues with its mobile application. However, I think GraphQL became so popular so fast not because it solves technical problems but rather because it solves communication problems.
Communication is hard. Improving our communication skills makes our lives better on many levels. Similarly, improving the communication between the different parts of a software application makes that application easier to understand, develop, maintain, and scale.
Thatâs why I think GraphQL is a game changer. It changes the game of how the different âendsâ of a software application (frontend and backend) communicate with each other. It gives them equal power, makes them independent of each other, decouples their communication process from its underlying technical transport channel, and introduces a rich new language in a place where the common previously spoken language was limited to a few words.
GraphQL powers many applications at Facebook today, including the main web application at facebook.com, the Facebook mobile application, and Instagram. Developersâ interest in GraphQL is very clear, and GraphQLâs adoption is growing fast. Besides Facebook, GraphQL is used in many other major web and mobile applications like GitHub, Airbnb, Yelp, Pinterest, Twitter, New York Times, Coursera, and Shopify. Given that GraphQL is a young technology, this is an impressive list.
In this first chapter, letâs learn what GraphQL is, what problems it solves, and what problems it introduces.
1.1 What is GraphQL?
The word graph in GraphQL comes from the fact that the best way to represent data in the real world is with a graph-like data structure. If you analyze any data model, big or small, youâll always find it to be a graph of objects with many relations between them.
That was the first âAha!â moment for me when I started learning about GraphQL. Why think of data in terms of resources (in URLs) or tables when you can think of it naturally as a graph?
Note that the graph in GraphQL does not mean that GraphQL can only be used with a âgraph database.â You can have a document database (like MongoDB) or a relational database (like PostgreSQL) and use GraphQL to represent your API data in a graph-like structure.
The QL in GraphQL might be a bit confusing, though. Yes, GraphQL is a query language for data APIs, but thatâs only from the perspective of the frontend consumer of those data APIs. GraphQL is also a runtime layer that needs to be implemented on the backend, and that layer is what makes the frontend consumer able to use the new language.
The GraphQL language is designed to be declarative, flexible, and efficient. Developers of data API consumers (like mobile and web applications) can use that language to request the data they need in a language close to how they think about data in their heads instead of a language related to how the data is stored or how data relations are implemented.
On the backend, a GraphQL-based stack needs a runtime. That runtime provides a structure for servers to describe the data to be exposed in their APIs. This structure is what we call a schema in the GraphQL world. An API consumer can then use the GraphQL language to construct a text request representing their exact data needs. The client sends that text request to the API service through a transport channel (for example, HTTPS). The GraphQL runtime layer accepts the text request, communicates with other services in the backend stack to put together a suitable data response, and then sends that data back to the consumer in a format like JSON. Figure 1.1 summarizes the dynamics of this communication.
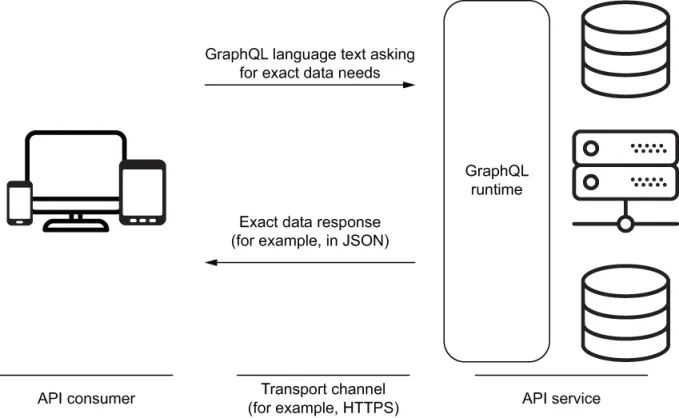
Figure 1.1 GraphQL is a language and a runtime.
1.1.1 The big picture
In general, an API is an interface that enables communication between multiple components in an application. For example, an API can enable the communication that needs to happen between a web client and a database server. The client tells the server what data it needs, and the server fulfills the clientâs requirement with objects representing the data the client asked for (figure 1.2).
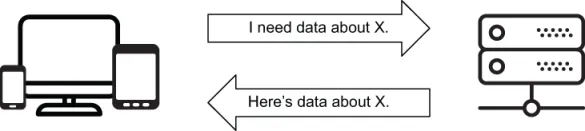
Figure 1.2 The big picture of data APIs
There are different types of APIs, and every big application needs them. For GraphQL, we are specifically talking about the API type used to read and modify data, which is usually referred to as a data API.
GraphQL is one option out of many that can be used to provide applications with programmable interfaces to read and modify the data the applications need from data services. Other options include REST, SOAP, XML, and even SQL itself.
SQL (Structured Query Language) might be directly compared to GraphQL because QL is in both names, after all. Both SQL and GraphQL provide a language to query data schemas. They can both be used to read and modify data. For example, if we have a table of data about a companyâs employees, the following is an example SQL statement to read data about the employees in one department.
Listing 1.1 SQL statement for querying
SELECT id, first_name, last_name, email, birth_date, hire_date FROM employees WHERE department = 'ENGINEERING'
Here is another example SQL statement that inserts data for a new employee.
Listing 1.2 SQL statement for mutating
INSERT INTO employees (first_name, last_name, email, birth_date, hire_date) VALUES ('Jane', 'Doe', '[email protected]', '01/01/1990', '01/01/2020')
You can use SQL to communicate data operations as we did i...