
React 17 Design Patterns and Best Practices
Design, build, and deploy production-ready web applications using industry-standard practices, 3rd Edition
Carlos Santana Roldán
- 394 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
React 17 Design Patterns and Best Practices
Design, build, and deploy production-ready web applications using industry-standard practices, 3rd Edition
Carlos Santana Roldán
About This Book
Build scalable, maintainable, and powerful React web apps with design patterns and insightful best practices
Key Features
- Make the most of design patterns in React – including render props and controlled and uncontrolled inputs
- Master React Hooks with the help of this updated third edition
- Work through examples that can be used to create reusable code and extensible designs
Book Description
Filled with useful React patterns that you can use in your projects straight away, this book will help you save time and build better web applications with ease.React 17 Design Patterns and Best Practices is a hands-on guide for those who want to take their coding skills to a new level. You'll spend most of your time working your way through the principles of writing maintainable and clean code, but you'll also gain a deeper insight into the inner workings of React.As you progress through the chapters, you'll learn how to build components that are reusable across the application, how to structure applications, and create forms that actually work. Then you'll build on your knowledge by exploring how to style React components and optimize them to make applications faster and more responsive.Once you've mastered the rest, you'll learn how to write tests effectively and how to contribute to React and its ecosystem.By the end of this book, you'll be able to avoid the process of trial and error and developmental headaches. Instead, you'll be able to use your new skills to efficiently build and deploy real-world React web applications you can be proud of.
What you will learn
- Get to grips with the techniques of styling and optimizing React components
- Create components using the new React Hooks
- Use server-side rendering to make applications load faster
- Get up to speed with the new React Suspense technique and using GraphQL in your projects
- Write a comprehensive set of tests to create robust and maintainable code
- Build high-performing applications by optimizing components
Who this book is for
This book is for web developers who want to understand React better and apply it to real-life app development. You'll need an intermediate-level experience with React and JavaScript before you get started.
]]>
Frequently asked questions
Information
- Installing PostgreSQL
- Creating environment variables with a .env file
- Configuring Apollo Server
- Defining GraphQL queries and mutations
- Working with resolvers
- Creating Sequelize models
- Implementing JWTs
- Using GraphQL Playground
- Performing authentication
Technical requirements
- Node.js 12+
- Visual Studio Code
- PostgreSQL
- Homebrew (https://brew.sh)
- pgAdmin 4 (https://www.pgadmin.org/download/)
- OmniDB (https://omnidb.org)
Installing PostgreSQL
brew install postgres
ln -sfv /usr/local/opt/postgresql/*.plist ~/Library/LaunchAgents
alias pg_start="launchctl load ~/Library/LaunchAgents"
alias pg_stop="launchctl unload ~/Library/LaunchAgents"
createdb `whoami`
createuser -s postgres
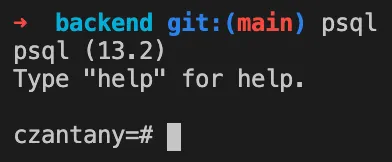
Best tools for PostgreSQL database management
FATAL: lock file "postmaster.pid" already exists.
If you get this error, you can easily fix it by running the rm /usr/local/var/postgres/postmaster.pid command. Then, you will be able to start your PostgreSQL server.
Creating our .env file and configuration files
npm init --yes
npm install @contentpi/lib @graphql-tools/load-files @graphql-tools/merge apollo-server dotenv express jso...