
eBook - ePub
Practical Robotics in C++
Build and Program Real Autonomous Robots Using Raspberry Pi (English Edition)
Lloyd Brombach
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Practical Robotics in C++
Build and Program Real Autonomous Robots Using Raspberry Pi (English Edition)
Lloyd Brombach
Book details
Book preview
Table of contents
Citations
About This Book
Learn how to build and program real autonomous robots
Key Features
- Simplified coverage on fundamentals of building a robot platform.
- Learn to program Raspberry Pi for interacting with hardware.
- Cutting-edge coverage on autonomous motion, mapping, and path planning algorithms for advanced robotics. Description
Practical Robotics in C++ teaches the complete spectrum of Robotics, right from the setting up a computer for a robot controller to putting power to the wheel motors. The book brings you the workshop knowledge of the electronics, hardware, and software for building a mobile robot platform. You will learn how to use sensors to detect obstacles, how to train your robot to build itself a map and plan an obstacle-avoiding path, and how to structure your code for modularity and interchangeability with other robot projects. Throughout the book, you can experience the demonstrations of complete coding of robotics with the use of simple and clear C++ programming.In addition, you will explore how to leverage the Raspberry Pi GPIO hardware interface pins and existing libraries to make an incredibly capable machine on the most affordable computer platform ever. What You Will Learn
- Write code for the motor drive controller.
- Build a Map from Lidar Data.
- Write and implement your own autonomous path-planning algorithm.
- Write code to send path waypoints to the motor drive controller autonomously.
- Get to know more about robot mapping and navigation. Who this book is for This book is most suitable for C++ programmers who have keen interest in robotics and hardware programming. All you need is just a good understanding of C++ programming to get the most out of this book. Table of Contents
1. Choose and Set Up a Robot Computer
2. GPIO Hardware Interface Pins Overview and Use
3. The Robot Platform
4. Types of Robot Motors and Motor Control
5. Communication with Sensors and other Devices
6. Additional Helpful Hardware
7. Adding the Computer to Control your Robot
8. Robot Control Strategy
9. Coordinating the Parts
10. Maps for Robot Navigation
11. Robot Tracking and Localization
12. Autonomous Motion
13. Autonomous Path Planning
14. Wheel Encoders for Odometry
15. Ultrasonic Range Detectors
16. IMUs: Accelerometers, Gyroscopes, and Magnetometers
17. GPS and External Beacon Systems
18. LIDAR Devices and Data
19. Real Vision with Cameras
20. Sensor Fusion
21. Building and Programming an Autonomous Robot About the Author
Lloyd Brombach is a controls engineer, programmer, and long-time electronics and robotics enthusiast. He has competed at robotics events such as the NASA-funded 2007 Lunar Regolith Excavation Challenge and recently the 27th Intelligent Ground Vehicle Challenge. He is committed to making contributions to the robotics field that future roboticists and robot-owners will benefit from for years to come. Facebook Profile: www.facebook.com/practicalrobotics
Youtube: www.youtube.com/practicalrobotics
LinkedIn Profile: https://www.linkedin.com/in/lbrombach
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Practical Robotics in C++ an online PDF/ePUB?
Yes, you can access Practical Robotics in C++ by Lloyd Brombach in PDF and/or ePUB format, as well as other popular books in Technik & Maschinenbau & Robotertechnik. We have over one million books available in our catalogue for you to explore.
Information
Subtopic
RobotertechnikCHAPTER 1
Choose and Set Up a Robot Computer
In this chapter, we will be covering the following topics:
- What is a Raspberry Pi?
- Raspberry Pi models and why not all are suitable for our purposes
- Operating system choices
- Operating system installation and setup
- Programming environment (IDE) installation and setup
What is a Raspberry Pi?
The Raspberry Pi is a small,
single-board computer
(SBC
). All that means that unlike your home desktop PC that might have a motherboard with slots or connectors for a processor, memory, video processor, and other components that you can swap and customize at will, an SBC is manufactured with these all permanently fixed to a single board. There is no changing or upgrading them without replacing the whole board. They donât have or run from a hard drive - the operating system runs from a micro SD card. There are several SBCs available today, but the Raspberry Pi is hands-down the most popular because of both itâs very low-cost and massive community support - if you have a problem, there are many thousands of Raspberry Pi enthusiasts eager to help you on social media groups or the official Raspberry Pi foundation-user forums at www.raspberrypi.org.Despite their minimal size (the biggest is about the size of a deck of cards), Raspberry Pis are fully functioning computers running a complete operating system (usually Linux) and capable of doing much of what your desktop PC can do such as web browsing, playing videos, connecting to printers and cameras, emailing, etc., as well as several things your desktop PC canât do without adding extra hardware like directly reading buttons, sensors or signalling the most common motor-controllers directly thanks to the
General Purpose Input/Output
(GPIO
) header that weâll spend all of Chapter 2, GPIO Hardware Interface Pins Overview and Use getting used to. Also, thanks to its GPIO header, small size, and simple projects Raspberry Pis get used for, they sometimes get confused for a type microcontroller such as an Arduino. Still, the fact is these are in two entirely different classes.Whatâs the difference then?
Among the big differences is the ability to write, compile, and execute programs directly on a Raspberry Pi. At the same time, a microcontroller requires you to write your programs and compile them on a computer, then download them to the microcontroller - which requires at least a USB cable and sometimes even a whole programming circuit. Once this is done, that program (which is limited to a much smaller amount of available memory, by the way) is the only thing your microcontroller can do until you repeat the whole process. A Raspberry Pi can run multiple programs at once - one of which can even be your microcontroller programming software. Since it is typical to be running multiple programs at once for all but the simplest of robots, we are going to exclude Arduinos and other microcontrollers from the list of suitable robot brains for our purposes.
So the Raspberry Pi is the only choice for a robot controller?
Not at all. Although it is a fantastic choice, most of the robot code we will be writing throughout this book will work just fine on any other computer without any modifications. The exceptions being anything to do with the GPIO. On other computers, we have to use external hardware to do their job, and that requires different code. Itâs often not cost-effective to dedicate a regular laptop and the extra hardware, cost, and space requirements for a relatively simple robotics project, but sometimes itâs worth the trade-offs. As weâll see later, computer vision takes a lot of computational power. This can go a lot faster if the computer has something called a
Graphics Processing Unit
(GPU
). These specialized processors are designed to handle operations on image data in large chunks at a time, making them magnitudes faster than a regular CPU. Nvidia
, one of the most prominent manufacturers of GPUs, released its low-cost single-board computer in 2019 called the Jetson Nano
. At a price point of $100 US
and including a legitimate, 128 core GPU and 40 pin GPIO header compatible with the Raspberry Pis, itâs bound to become a common name in the robotics world. Between the even lower price (making it available to more people) and huge community support network that the Jetson just doesnât have yet (although itâs rapidly growing!), I still have to give the title of Ideal learning computer for robotics to the Raspberry Pi. Thatâs why weâve chosen to do our example projects with a Raspberry Pi for a controller.Isnât the Raspberry Pi for schools, hobbyists, and toys? I wanted to learn about real robotics.
You can indeed find a Raspberry Pi in the hands of all manner of toys and hobbyists, but that just speaks for its high power at a remarkably affordable price. Universities love for them how much teaching can be done on them for so little money, and I recently traveled to an international robotics summit and was surprised at the number of commercial robot companies that deploy their autonomous robot products with a Raspberry Pi as a controller. Following figure 1.1 shows just one example.
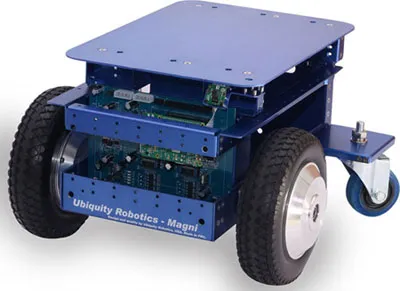
Figure 1.1: Ubiquity Roboticâs Magni is just one of a number of commercially available robots powered by a Raspberry Pi.
source: Ubiquity Robotics
source: Ubiquity Robotics
Magni and other commercial, Raspberry Pi-based robots can do things like security patrol, work in warehouses, office or baggage delivery, serve as cocktail waiters, and plenty more. I think youâll find a Raspberry Pi far more capable than
just a toy
and highly recommend starting your robotics-learning career with one.Raspberry Pi models and why not all are suitable for our purposes
Take a look at the comparison chart on the following figure 1.2. These are the most popular and readily available models of Raspberry Pi. I have omitted the less-common (and older) A and B models and place them in the
not suitable for our purposes
category. This is because of factors such as slow speeds, lack of memory, and a lot of software packages we might benefit from just wonât run on them. They certainly could be made to work, but even in the cases where I might use one, I would generally use a Zero
or ZeroW
models instead. Itâs just a better deal for the money.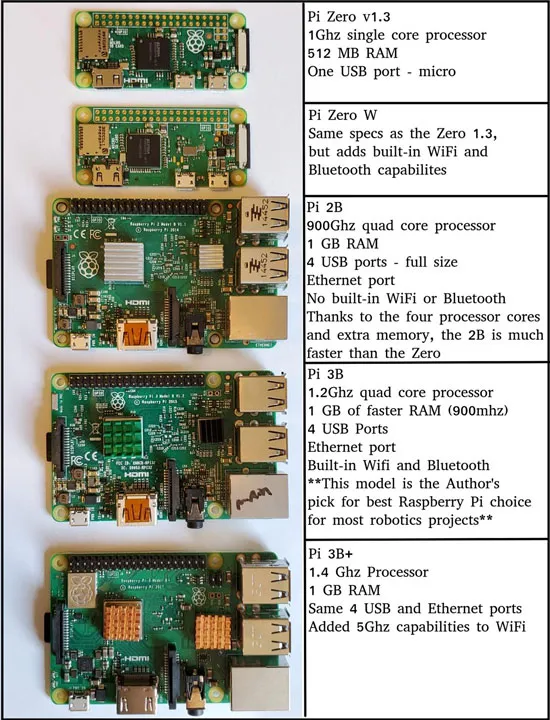
Figure 1.2: A comparison of the most popular Raspberry Pi models.
Before you run off and buy the most powerful Raspberry Pi listed (or the new Raspberry Pi 4 â not pictured), please read the rest of this chapter as there are reasons the 3B+ or 4 may not be the best choice.
Raspberry Pi Zero and Raspberry Pi ZeroW
The Raspberry Pi
Zero
and Zero W
models are great little machines for a meagre price, but Iâm afraid they just havenât got what it takes to be real robot controllers, except perhaps the most basic of obstacle avoidance bots. (The Zero W
is a regular Zero
with built-in Wi-Fi and Bluetooth adapters). I tend to use them where a microcontroller would probably do the job. Still, I might want to remotely reprogram it or perform functions that it makes easier than a microcontroller such as display or web access functions, or where I want the batteries to last a long time - the Zeros have no close competition among the other Pis as far as being low-power goes.The problem arises from both its lack of memory and its single-core processor. This is less of a problem if you are an old Linux pro and are happy without a GUI, but if you like to use an
Integrated Development Environment
(IDE
), the little Zeros are going to struggle. Try running your IDE over Virtual Network Computing
(VNC
) for remote access, and you might as well forget it. For these reasons, I tend to limit my use of Zeros to things with smaller programs that I donât mind editing from a plain text editor and compiling from the command line.Also, be aware that the
Zero
models require an adapter to output to a standard HDMI monitor, and another to plug in a standard USB device like a keyboard. In the case of the less-expensive Zero 1.3
, you will need a separate Wi-Fi adapter as well, which means you need a USB hub to have both Wi-Fi and keyboard, plus you have to buy and solder in your header pins for the GPIO. All this makes it less affordable than it seems at first glance.Verdict:
Not for a fully autonomous machine.
![]() | We love the Raspberry Pi Zeros and it can be tempting to buy one for your project to save money. Still, plenty of folks new to the little computers have been disappointed when they discover that they have to buy extra adapters or how painfully slow they are â especially when using the graphical user environment. |
The Raspberry Pi 2B
The
Pi 2B
is the first model I find genuinely suitable for a real, autonomous robotics project. The full gigabyte of memory and quad-core processor makes it start to feel like a real computer and less like Iâm trying to surf the Internet with a slow dialup connection back in 1995. You will have to buy a USB adapter for Wi-Fi if you donât have one lying about, but thatâs about it. Unlike the Zeros, it comes with header pins already soldered on so you can start plugging devices straight away.Notice: I said
start
to feel like a real computer - The Pi 2B is still on the sluggish side at some things like web browsing or when running remotely over VNC.
This is a way of remotely controlling one computer from another, and it can be done from across the room or the planet. There are other ways to accomplish remote control of a computer, but VNC allows the sharing of the graphical environment so you can do everything with a remote machine as if you were plugged right into it. This can be very useful when developing robots.
Verdict:
Acceptable choice, but unless you already have a USB Wi-Fi adapter lying around, you can get the even faster 3B model for about the sam...