
Microservices with Spring Boot and Spring Cloud
Build resilient and scalable microservices using Spring Cloud, Istio, and Kubernetes, 2nd Edition
Magnus Larsson
- 774 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Microservices with Spring Boot and Spring Cloud
Build resilient and scalable microservices using Spring Cloud, Istio, and Kubernetes, 2nd Edition
Magnus Larsson
About This Book
Create and deploy production-quality microservices-based applications â New edition updated for the smooth running of Spring, Java, Kubernetes, and Istio, with an introduction to Helm 3 and support for Mac and Windows with WSL2
Key Features
- Build cloud-native production-ready microservices with this comprehensively updated guide
- Understand the challenges of building large-scale microservice architectures
- Learn how to get the best out of Spring Cloud, Kubernetes, and Istio in combination
Book Description
Want to build and deploy microservices, but don't know where to start? Welcome to Microservices with Spring Boot and Spring Cloud.
This edition features the most recent versions of Spring, Java, Kubernetes, and Istio, demonstrating faster and simpler handling of Spring Boot, local Kubernetes clusters, and Istio installation. The expanded scope includes native compilation of Spring-based microservices, support for Mac and Windows with WSL2, and an introduction to Helm 3 for packaging and deployment. A revamped security chapter now follows the OAuth 2.1 specification and makes use of the newly launched Spring Authorization Server from the Spring team.
You'll start with a set of simple cooperating microservices, then add persistence and resilience, make your microservices reactive, and document their APIs using OpenAPI.
Next, you'll learn how fundamental design patterns are applied to add important functionality, such as service discovery with Netflix Eureka and edge servers with Spring Cloud Gateway. You'll deploy your microservices using Kubernetes and adopt Istio, then explore centralized log management using the Elasticsearch, Fluentd, and Kibana (EFK) stack, and then monitor microservices using Prometheus and Grafana.
By the end of this book, you'll be building scalable and robust microservices using Spring Boot and Spring Cloud.
What you will learn
- Build reactive microservices using Spring Boot
- Develop resilient and scalable microservices using Spring Cloud
- Use OAuth 2.1/OIDC and Spring Security to protect public APIs
- Implement Docker to bridge the gap between development, testing, and production
- Deploy and manage microservices with Kubernetes
- Apply Istio for improved security, observability, and traffic management
- Write and run automated microservice tests with JUnit, testcontainers, Gradle, and bash
Who this book is for
If you're a Java or Spring Boot developer learning how to build microservice landscapes from scratch, then this book is for you. You don't need any prior knowledge about microservices architecture to get started, but a solid grasp and enough experience in Java and Spring Boot to build apps autonomously is a must.
Frequently asked questions
Information
18
Using a Service Mesh to Improve Observability and Management
- An introduction to the service mesh concept and Istio, a popular open source implementation
- Deploying Istio in Kubernetes
- Creating, observing, and securing a service mesh
- Ensuring that a service mesh is resilient
- Performing zero-downtime updates
- Testing the microservice landscape using Docker Compose to ensure that the source code in the microservices is not locked into either Kubernetes or Istio
Technical requirements
- Chapter 21 for macOS
- Chapter 22 for Windows
$BOOK_HOME/Chapter18
.diff
tool and compare the two folders, $BOOK_HOME/Chapter17
and $BOOK_HOME/Chapter18
.Introducing service meshes using Istio
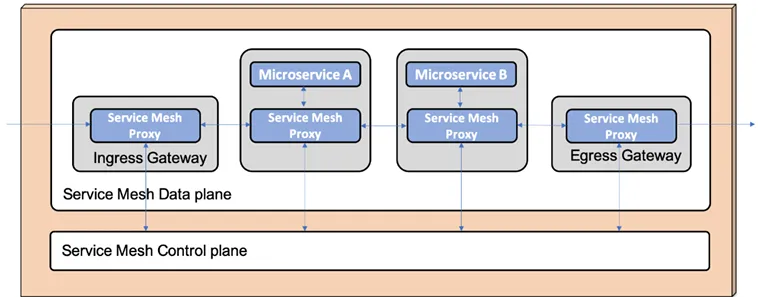
Introducing Istio
istioctl
, to install Istio in our Minikube-based, single-node Kubernetes cluster.