
Reactive Patterns with RxJS for Angular
Lamis Chebbi
- 224 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Reactive Patterns with RxJS for Angular
Lamis Chebbi
About This Book
Discover how you can leverage the reactive paradigm with RxJS in your Angular applications using some common patterns and techniques that can be implemented in real-world use cases
Key Features
- Learn how to write clean, maintainable, performant, and optimized Angular web applications using reactive patterns
- Explore various RxJS operators and techniques in detail to improve the testing and performance of your code
- Switch from an imperative mindset to reactive by comparing both
Book Description
RxJS is a fast, reliable, and compact library for handling asynchronous and event-based programs. It is a first-class citizen in Angular and enables web developers to enhance application performance, code quality, and user experience, so using reactive patterns in your Angular web development projects can improve user interaction on your apps, which will significantly improve the ROI of your applications.This book is a step-by-step guide to learning everything about RxJS and reactivity. You'll begin by understanding the importance of the reactive paradigm and the new features of RxJS 7. Next, you'll discover various reactive patterns, based on real-world use cases, for managing your application's data efficiently and implementing common features using the fewest lines of code.As you build a complete application progressively throughout the book, you'll learn how to handle your app data reactively and explore different patterns that enhance the user experience and code quality, while also improving the maintainability of Angular apps and the developer's productivity. Finally, you'll test your asynchronous streams and enhance the performance and quality of your applications by following best practices.By the end of this RxJS Angular book, you'll be able to develop Angular applications by implementing reactive patterns.
What you will learn
- Understand how to use the marble diagram and read it for designing reactive applications
- Work with the latest features of RxJS 7
- Build a complete Angular app reactively, from requirement gathering to deploying it
- Become well-versed with the concepts of streams, including transforming, combining, and composing them
- Explore the different testing strategies for RxJS apps, their advantages, and drawbacks
- Understand memory leak problems in web apps and techniques to avoid them
- Discover multicasting in RxJS and how it can resolve complex problems
Who this book is for
If you're an Angular developer who wants to leverage RxJS for building reactive web applications, this is the book for you. Beginner-level experience with Angular and TypeScript and knowledge of functional programming concepts is assumed.
]]>
Frequently asked questions
Information
Part 1 â Introduction
- Chapter 1, The Power of the Reactive Paradigm
- Chapter 2, RxJS 7 â The Major Features
- Chapter 3, A Walkthrough of the Application
Chapter 1: The Power of the Reactive Paradigm
- Exploring the pillars of reactive programming
- Using RxJS in Angular and its advantages
- Learning about the marble diagram â our secret weapon
Technical requirements
Exploring the pillars of reactive programming
Data streams
Observer patterns
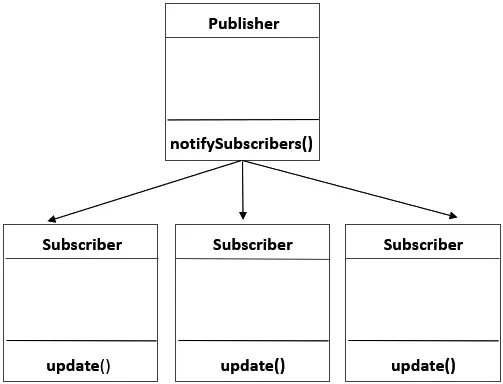