
Mastering Concurrency Programming with Java 9 - Second Edition
Javier Fernandez Gonzalez
- 516 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mastering Concurrency Programming with Java 9 - Second Edition
Javier Fernandez Gonzalez
About This Book
Master the principles to make applications robust, scalable and responsiveAbout This Book⢠Implement concurrent applications using the Java 9 Concurrency API and its new components⢠Improve the performance of your applications and process more data at the same time, taking advantage of all of your resources⢠Construct real-world examples related to machine learning, data mining, natural language processing, and moreWho This Book Is ForThis book is for competent Java developers who have basic understanding of concurrency, but knowledge of effective implementation of concurrent programs or usage of streams for making processes more efficient is not requiredWhat You Will Learn⢠Master the principles that every concurrent application must follow⢠See how to parallelize a sequential algorithm to obtain better performance without data inconsistencies and deadlocks⢠Get the most from the Java Concurrency API components⢠Separate the thread management from the rest of the application with the Executor component⢠Execute phased-based tasks in an efficient way with the Phaser components⢠Solve problems using a parallelized version of the divide and conquer paradigm with the Fork / Join framework⢠Find out how to use parallel Streams and Reactive Streams⢠Implement the "map and reduce" and "map and collect" programming models⢠Control the concurrent data structures and synchronization mechanisms provided by the Java Concurrency API⢠Implement efficient solutions for some actual problems such as data mining, machine learning, and moreIn DetailConcurrency programming allows several large tasks to be divided into smaller sub-tasks, which are further processed as individual tasks that run in parallel. Java 9 includes a comprehensive API with lots of ready-to-use components for easily implementing powerful concurrency applications, but with high flexibility so you can adapt these components to your needs.The book starts with a full description of the design principles of concurrent applications and explains how to parallelize a sequential algorithm. You will then be introduced to Threads and Runnables, which are an integral part of Java 9's concurrency API. You will see how to use all the components of the Java concurrency API, from the basics to the most advanced techniques, and will implement them in powerful real-world concurrency applications.The book ends with a detailed description of the tools and techniques you can use to test a concurrent Java application, along with a brief insight into other concurrency mechanisms in JVM.Style and approachThis is a complete guide that implements real-world examples of algorithms related to machine learning, data mining, and natural language processing in client/server environments. All the examples are explained using a step-by-step approach.
Frequently asked questions
Information
Concurrency in JVM - Clojure and Groovy with the Gpars Library and Scala
- Clojure: Provides reference types such as Atom and Agent and other elements such as Future and Promise
- Groovy: With the GPars library, provides elements for data parallelization, its own actor model, agents, and dataflow
- Scala: Provides two elements, futures and promises
Concurrency in Clojure
- Clojure data structures are immutable, so they can be shared between threads without any problem. This does not mean that you can't have mutable values on concurrent applications as you'll see later.
- Clojure separates the concepts of identity and value, almost deleting the need for explicit locks.
Using Java elements
(ns example.example1) (defn example1 ( [number] (println (format "%s : %d"(Thread/currentThread) number)) )) (dotimes [i 10] (.start (Thread. (fn[] (example1 I)))))
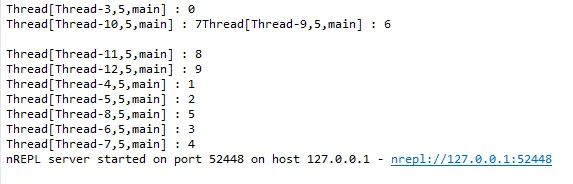
Reference types
- Coordinated: When two or more operations cooperate with each other
- Uncoordinated: When the operation doesn't affect other operations
- Synchronous: When the caller waits for the finalization of the operation
- Asynchronous: When the caller doesn't wait for the finalization of the operation
- Atoms
- Agents
- Refs
Atoms
- atom: To define a new atom object.
- swap!: Atomically changes the value of the atom to a new one based on the result of a function. It follows the format (swap! atom function) where atom is the name of the atom object, and function is the function that returns the new value of the atom.
- reset!: Establish the value of the atom to a new value. It follows the format (reset! atom value) where atom is the name of the atom object and value the new value.
- compare-and-set!: Atomically changes the value of the atom if the actual value is the same as the value passed as a parameter. It follows the format (compare-and-set! atom old-value new-value) where atom is the name of the atom object, old-value is the expected actual value of the atom, and new value is the new value we want to assign to the atom.
(ns example.example2) (defn company ( [account salary] (swap! account + salary) (println (format "%s : %d"(Thread/currentThread) @account)) ))
(defn user ( [account money] (swap! account - money) (println (format "%s : %d"(Thread/currentThread) @account)) ))
(defn myTask ( [account] (dotimes [i 1000] (company account 100) (user account 100) (Thread/sleep 100) )))
(def myAccount (atom 0)) (dotimes [i 10] (.start (Thread. (fn[] (myTask myAccount)))))
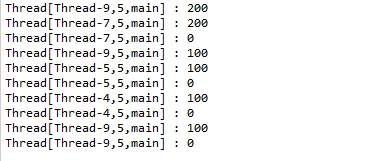
Agents
- agent: To create a new Agent object.
- send: To establish the new value of the agent. It follows the syntax (send agent function value) where agent is the name of the agent we want to modify, function is a function to be executed to calculate the new value of the agent, and value is the value that will be passed to the function with the actual value of the agent to calculate the new one.
- send-of: You can use this function when you want to use a function to update the value that is a blocking function (for example, reading a file). The send-of function will return immediately and the function that updates the value of the agent continues its execution in another thread. It follows the same syntax as the send function.
- await: Waits (blocking the current thread) until all the pending operations with the agent have finished. If follows the syntax (await agent) where agent is the name of the agent we want to wait for.
- await-for: You can use this function to wait the number of milliseconds specif...