
Mobile Test Automation with Appium
Nishant Verma
- 256 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mobile Test Automation with Appium
Nishant Verma
About This Book
Automate your mobile app testingAbout This Book• How to automate testing with Appium• Apply techniques for creating comprehensive tests• How to test on physical devices or emulatorsWho This Book Is ForAre you a mobile developer or a software tester who wishes to use Appium for your test automation? If so, then this is the right book for you.You must have basic Java programming knowledge. You don't need to have prior knowledge of Appium.What You Will Learn• Discover Appium and how to set up an automation framework for mobile testing• Understand desired capabilities and learn to find element locators• Learn to automate gestures and synchronize tests using Appium• Take an incremental approach to implement page object pattern• Learn to run Appium tests on emulators or physical devices• Set up Jenkins to run mobile automation tests by easy to learn steps• Discover tips and tricks to record video of test execution, inter app automation concepts• Learn to run Appium tests in parallel on multiple devices simultaneouslyIn DetailAppium is an open source test automation framework for mobile applications. It allows you to test all three types of mobile applications: native, hybrid, and mobile web. It allows you to run the automated tests on actual devices, emulators, and simulators. Today, when every mobile app is made on at least two platforms, iOS and Android, you need a tool that allows you to test across platforms.Having two different frameworks for the same app increases the cost of the product and time to maintain it as well. Appium helps save this cost.With mobile app growth exploding, mobile app automation is mainstream now. In this book, author Nishant Verma provides you with a firm grounding in the concepts of Appium while diving into how to set up appium & Cucumber-jvm test automation framework, implement page object design pattern, automate gestures, test execution on emulators and physical devices, and implement continuous integration with Jenkins. The mobile app we have referenced in this book is Quikr because of its relatively lower learning curve to understand the application. It's a local classifieds shopping app.Style and approachThis book takes a practical, step-by-step approach to testing and automating individual apps such as native, hybrid, and mobile web apps using different examples.
Frequently asked questions
Information
Writing Your First Appium Test
- Create a sample Java project
- Add Appium (automation tool) as a dependency
- Add Cucumber-JVM as a dependency
- Write a small test for a mobile web
Creating an Appium Java project (using gradle)
- Launch IntelliJ and click on Create New Project on the welcome screen.
- On the New Project screen, select Gradle from the left pane. Project SDK should get populated with the Java version.
- Click on Next, enter the GroupId as com.test and ArtifactId as HelloAppium. The version will already be populated; click on Next.
- Check the Use auto-import option and ensure that Gradle JVM is populated. Click on Next. In case the Gradle JVM is not populated, please follow the below steps:

-
- Click on Configure > Project Defaults > Project Structure:

-
- Choose Project under Project Settings as shown below:

-
- Click on New... button.
- Point it to the JDK installed on your machine.
-
- Click on OK to close the pop up and go to the new Project creation screen.
- The Project name field will be auto-populated with what you gave as ArtifactId. Choose a Project location and click on Finish. IntelliJ will be running the background task (Gradle build), which can be seen in the status bar.
- This should create a project with the following structure:
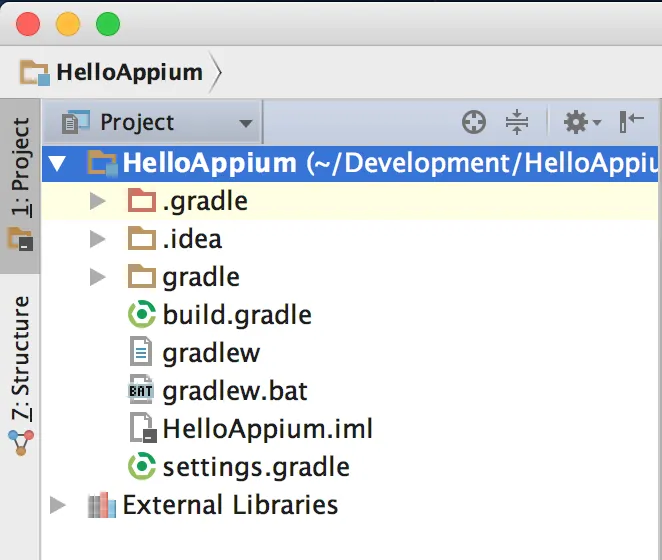
- Open the build.gradle file. You will see a message, as shown; click on Ok, apply suggestion!:

- Enter the following two lines in build.gradle. This adds Appium and cucumber-jvm under dependencies:
compile group: 'info.cukes', name: 'cucumber-java',
version: '1.2.5'
compile group: 'io.appium', name: 'java-client',
version: '5.0.0-BETA6'
- Here's how the gradle file should look:

- Once done, navigate to View > Tools Windows > Gradle and click on the Refresh all gradle projects icon. This will pull all the dependencies in External Libraries:

- Navigate to Preferences > Plugins, search for Cucumber for Java, and click on Install (if it's not previously installed).
- Repeat the preceding step for Gherkin and install the same. Once done, restart IntelliJ if it prompts.
Introduction to Cucumber
Given I launch the app
And I click on Register
Then I should see register with Facebook and Google