
C++ Data Structures and Algorithms
Wisnu Anggoro
- 322 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
C++ Data Structures and Algorithms
Wisnu Anggoro
About This Book
Learn how to build efficient, secure and robust code in C++ by using data structures and algorithms - the building blocks of C++About This Book⢠Use data structures such as arrays, stacks, trees, lists, and graphs with real-world examples⢠Learn the functional and reactive implementations of the traditional data structures⢠Explore illustrations to present data structures and algorithms, as well as their analysis, in a clear, visual mannerWho This Book Is ForThis book is for developers who would like to learn the Data Structures and Algorithms in C++. Basic C++ programming knowledge is expected.What You Will Learn⢠Know how to use arrays and lists to get better results in complex scenarios⢠Build enhanced applications by using hashtables, dictionaries, and sets⢠Implement searching algorithms such as linear search, binary search, jump search, exponential search, and more⢠Have a positive impact on the efficiency of applications with tree traversal⢠Explore the design used in sorting algorithms like Heap sort, Quick sort, Merge sort and Radix sort⢠Implement various common algorithms in string data types⢠Find out how to design an algorithm for a specific task using the common algorithm paradigmsIn DetailC++ is a general-purpose programming language which has evolved over the years and is used to develop software for many different sectors. This book will be your companion as it takes you through implementing classic data structures and algorithms to help you get up and running as a confident C++ programmer.We begin with an introduction to C++ data structures and algorithms while also covering essential language constructs. Next, we will see how to store data using linked lists, arrays, stacks, and queues. Then, we will learn how to implement different sorting algorithms, such as quick sort and heap sort. Along with these, we will dive into searching algorithms such as linear search, binary search and more. Our next mission will be to attain high performance by implementing algorithms to string datatypes and implementing hash structures in algorithm design. We'll also analyze Brute Force algorithms, Greedy algorithms, and more.By the end of the book, you'll know how to build components that are easy to understand, debug, and use in different applications.Style and approachReaders will be taken through an indispensable list of data structures and algorithms so they can confidently begin coding in C++.
Frequently asked questions
Information
Storing Data in Lists and Linked Lists
- Understanding the array data type and how to use it
- Building the list data structure using the array data type
- Understanding the concept of node and node chaining
- Building SinglyLinkedList and DoublyLinkedList using node chaining
- Applying the Standard Template Library to implement the list and linked list
Technical requirements
- A desktop PC or Notebook with Windows, Linux, or macOS
- GNU GCC v5.4.0 or above
- Code::Block IDE v17.12 (for Windows and Linux OS) or Code::Block IDE v13.12 (for macOS)
- You will find the code files on GitHub at https://github.com/PacktPublishing/CPP-Data-Structures-and-Algorithms
Getting closer to an array
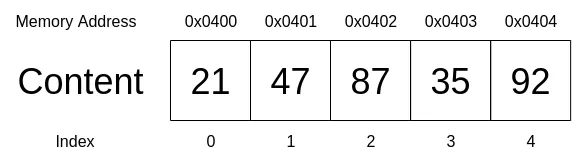
// Project: Array.cbp
// File : Array.cpp
#include <iostream>
using namespace std;
int main()
{
// Initialize an array
int arr[] = { 21, 47, 87, 35, 92 };
// Access each element
cout << "Array elements: ";
for(int i = 0; i < sizeof(arr)/sizeof(*arr); ++i)
cout << arr[i] << " ";
cout << endl;
// Manipulate several elements
arr[2] = 30;
arr[3] = 64;
// Access each element again
cout << "Array elements: ";
for(int i = 0; i < sizeof(arr)/sizeof(*arr); ++i)
cout << arr[i] << " ";
cout << endl;
return 0;
}

int * ptr = new int[5] { 21, 47, 87, 35, 92 };
cout << *ptr << endl;
cout << ptr << endl;
cout << ptr[i] << endl;
cout << &ptr[i] << endl;
// Project: Array_As_Pointer.cbp
// File : Array_As_Pointer.cpp
#include <iostream>
using namespace std;
int main()
{
// Initialize the array length
int arrLength = 5;
// Initialize a pointer
// to hold an array
int * ptr = new int[arrLength] { 21, 47, 87, 35, 92 };
// Display each element value
// by incrementing the pointer (ptr++)
cout << "Using pointer increment" << endl;
cout << "Value\tAddress" << endl;
while(*ptr)
{
cout << *ptr << "\t";
cout << ptr << endl;
ptr++;
}
// Since we have moved forward the pointer five tim...