
Mastering Spring Boot 2.0
Build modern, cloud-native, and distributed systems using Spring Boot
Dinesh Rajput
- 390 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mastering Spring Boot 2.0
Build modern, cloud-native, and distributed systems using Spring Boot
Dinesh Rajput
About This Book
Learn to develop, test, and deploy your Spring Boot distributed application and explore various best practices.
Key Features
- Build and deploy your microservices architecture in the cloud
- Build event-driven resilient systems using Hystrix and Turbine
- Explore API management tools such as KONG and API documentation tools such as Swagger
Book Description
Spring is one of the best frameworks on the market for developing web, enterprise, and cloud ready software. Spring Boot simplifies the building of complex software dramatically by reducing the amount of boilerplate code, and by providing production-ready features and a simple deployment model.
This book will address the challenges related to power that come with Spring Boot's great configurability and flexibility. You will understand how Spring Boot configuration works under the hood, how to overwrite default configurations, and how to use advanced techniques to prepare Spring Boot applications to work in production. This book will also introduce readers to a relatively new topic in the Spring ecosystem – cloud native patterns, reactive programming, and applications. Get up to speed with microservices with Spring Boot and Spring Cloud. Each chapter aims to solve a specific problem or teach you a useful skillset. By the end of this book, you will be proficient in building and deploying your Spring Boot application.
What you will learn
- Build logically structured and highly maintainable Spring Boot applications
- Configure RESTful microservices using Spring Boot
- Make the application production and operation-friendly with Spring Actuator
- Build modern, high-performance distributed applications using cloud patterns
- Manage and deploy your Spring Boot application to the cloud (AWS)
- Monitor distributed applications using log aggregation and ELK
Who this book is for
The book is targeted at experienced Spring and Java developers who have a basic knowledge of working with Spring Boot. The reader should be familiar with Spring Boot basics, and aware of its benefits over traditional Spring Framework-based applications.
Frequently asked questions
Information
Containerizing Microservice
- Introducing containers in the microservice architecture
- Getting started with Docker
- Dockerizing any Spring Boot application
- Writing Dockerfile
- Using docker-compose
- Writing the docker-compose file
- Orchestration using docker-compose
- Introducing Kubernetes
- Orchestration using Kubernetes
Introducing containers to the microservice architecture
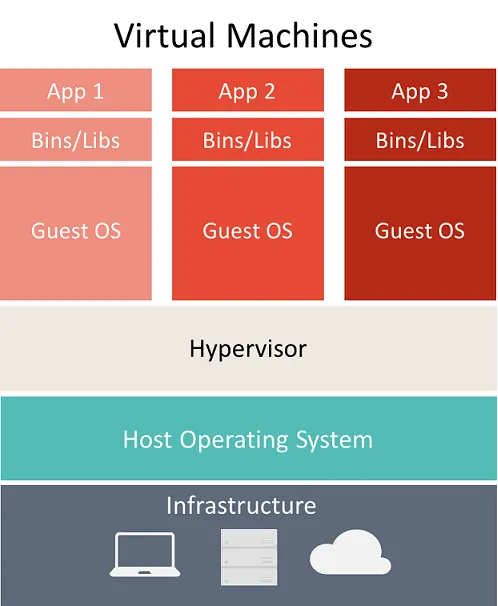
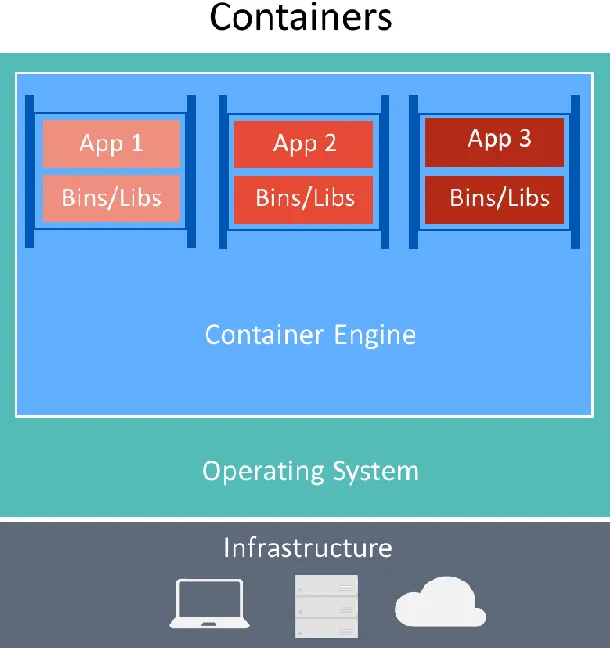
Virtual machines versus containers
Virtual machines | Containers |
Virtual machines include the applications, the required dependencies, and a full guest operating system | Containers include the applications and the required dependencies, and share operating systems and underlying infrastructure |
Each virtual machine has its own guest operating system; because of this, it requires more resources | Because containers share resources, they require fewer resource, the minimal kernel of the operating system present for each container |
The hypervisor manages VMs, environments | The container engine manages containers |
You have to add specific resources for scaling | You can scale out containers by creating another container of an image |
Fewer virtual machines can be created for the same hardware and resources | More containers can be created for the same hardware and resources |
Virtual machines are virtualizing the underlying hardware | Containers are virtualizing the underlying operating system |
A VM can take up several GB depending on guest OS | Containers don't require that many GB, because they share resources, they merely use dozens of MB |
Virtual machines are generally more suitable for monolithic applications with high-security concerns | Containers are generally more suitable for microservice-based applications, or other cloud-native applications, where security is not the major concern |
Benefits of a container-oriented approach
- A container-oriented approach eliminates the challenges that arise from inconsistent environment setups.
- You can do fast application scale-up by instancing new containers as required.
- Requires minimal usage of kernels on the operating system.
- You can create the number of containers for a microservice, depending on application requirements.
- You can easily...