
Java EE 8 Development with Eclipse
Develop, test, and troubleshoot Java Enterprise applications rapidly with Eclipse, 3rd Edition
Ram Kulkarni
- 596 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Java EE 8 Development with Eclipse
Develop, test, and troubleshoot Java Enterprise applications rapidly with Eclipse, 3rd Edition
Ram Kulkarni
About This Book
Develop and deploy fully functional applications and microservices utilising Tomcat, Glassfish servers, Cloud and docker in Java EE 8About This Book• Explore the complete workflow of developing enterprise Java applications• Develop microservices with Docker Container and deploy it in cloud• Simplify Java EE application developmentWho This Book Is ForIf you are a Java developer with little or no experience in Java EE application development, or if you have experience in Java EE technology but are looking for tips to simplify and accelerate your development process, then this book is for you.What You Will Learn• Set up Eclipse, Tomcat, and Glassfish servers for Java EE application development• Use JSP, Servlet, JSF, and EJBs to create a user interface and write business logic• Create Java EE database applications using JDBC and JPA• Handle asynchronous messages using MDBs for better scalability• Deploy and debug Java EE applications and create SOAP and REST web services• Write unit tests and calculate code coverage• Use Eclipse MAT (Memory Analysis Tool) to debug memory issues• Create and deploy microservicesIn DetailJava EE is one of the most popular tools for enterprise application design and development. With recent changes to Java EE 8 specifications, Java EE application development has become a lot simpler with the new specifications, some of which compete with the existing specifications. This guide provides a complete overview of developing highly performant, robust and secure enterprise applications with Java EE with Eclipse.The book begins by exploring different Java EE technologies and how to use them (JSP, JSF, JPA, JDBC, EJB, and more), along with suitable technologies for different scenarios. You will learn how to set up the development environment for Java EE applications and understand Java EE specifications in detail, with an emphasis on examples. The book takes you through deployment of an application in Tomcat, GlassFish Servers, and also in the cloud. It goes beyond the basics and covers topics like debugging, testing, deployment, and securing your Java EE applications. You'll also get to know techniques to develop cloud-ready microservices in Java EE.Style and approachThis guide takes a step-by-step approach to developing, testing, debugging, and troubleshooting Java EE applications, complete with examples and tips.
Frequently asked questions
Information
Creating JEE Database Applications
- Core JDBC concepts
- Using JDBC to access the database
- Using JDBC connection pool
- Core JPA concepts
- Using JPA to map entities (classes) to tables in the database
- Configuring relationships between JPA entities
Creating database schema
- You can use data description language (DDL) statements directly at MySQL Command Prompt from the Terminal
- You can use MySQL Workbench and create tables directly
- You can create an entity-relationship diagram in MySQL Workbench, export it to create a DDL script, and then run this script to create tables and relationships
- Open MySQL Workbench. Select the File | New Model menu. A blank model will be created with the option to create ER diagrams:
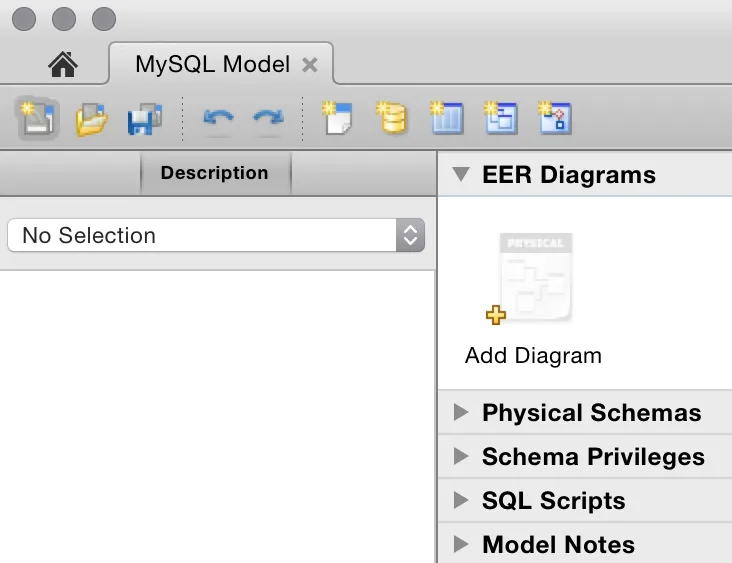
- Double-click the Add Diagram icon; a blank ER diagram will be opened:
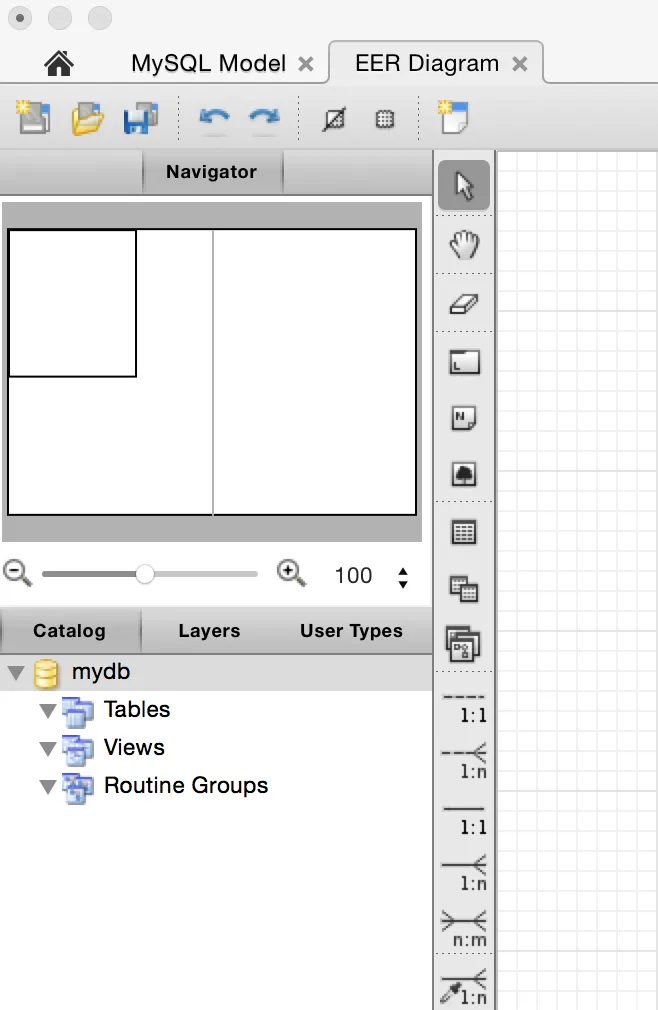
- By default, the new schema is named mydb. Double-click on it to open properties of the schema. Rename the schemacourse_management:

- Hover over the toolbar buttons on the left-hand side of the page, and you will see tool tips about their functions. Click on the button for a new table and then click on the blank page. This will insert a new table with the name table1. Double-click the table icon to open the Properties page of the table. In the Properties page, change the name of the table to Course:

- We will now create columns of the table. Double-click on the first column and name it id. Check the PK (primary key), NN (not null), and AI (auto increment) checkboxes. Add other columns as shown in the following screenshot:
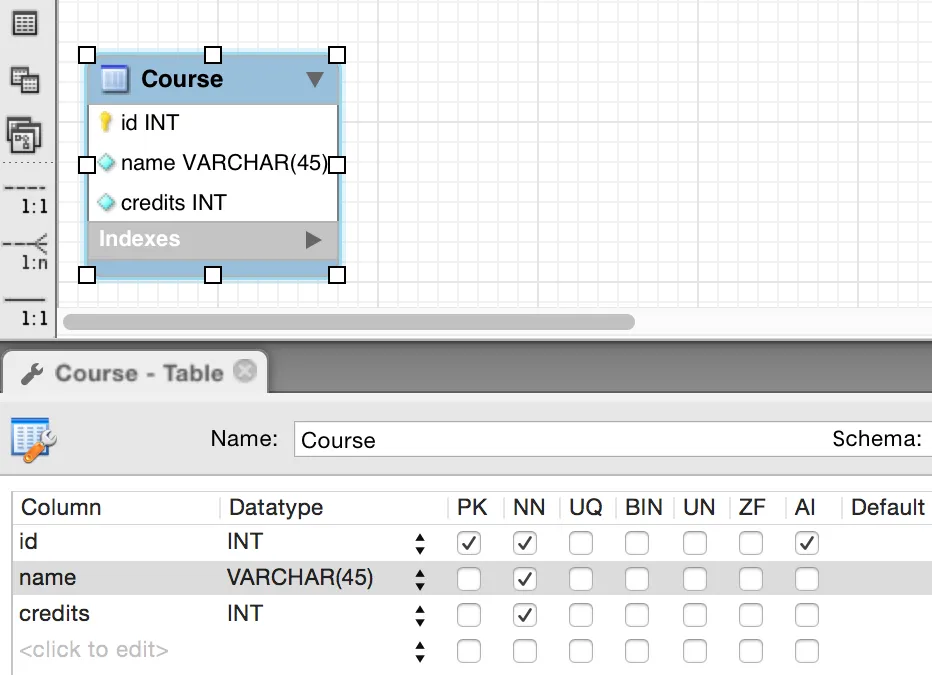
- Create other tables, namely Student and Teacher, as shown in the following screenshot:
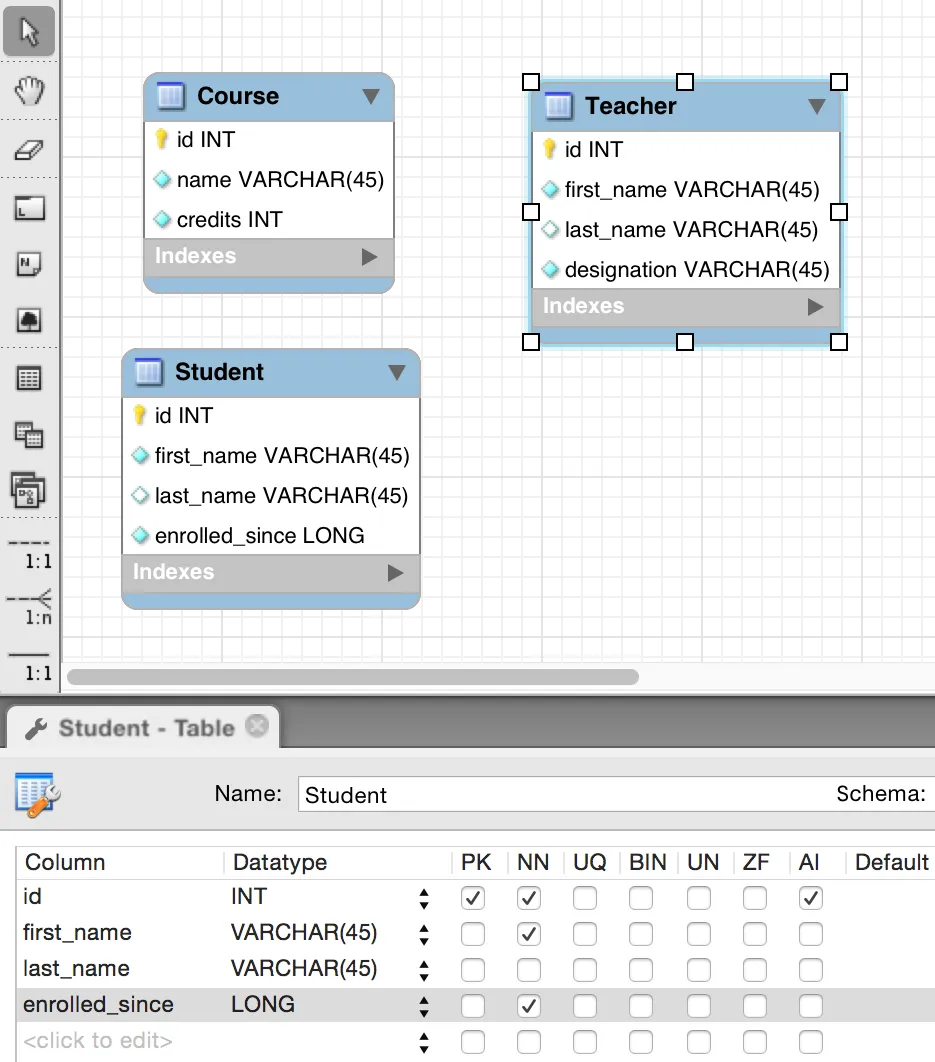
- First, we will create a non-identifying relationship between Course and Teacher.
- Click on the non-identifying one-to-many button in the toolbar (dotted lines and 1:n).
- Then, click on the Course table first and then on the Teacher table. It will create a relationship as shown in Figure 4.7. Note that a foreign key Teacher_id is created in the Course table. We don't want to make a Teacher_id field required in Course. A course can exist without a teacher in our application. Therefore, double-click on the link joining Course and Teacher tables.
- Then, click on the Foreign Key tab.
- On the Referenced Table side, uncheck the Mandatory checkbox:

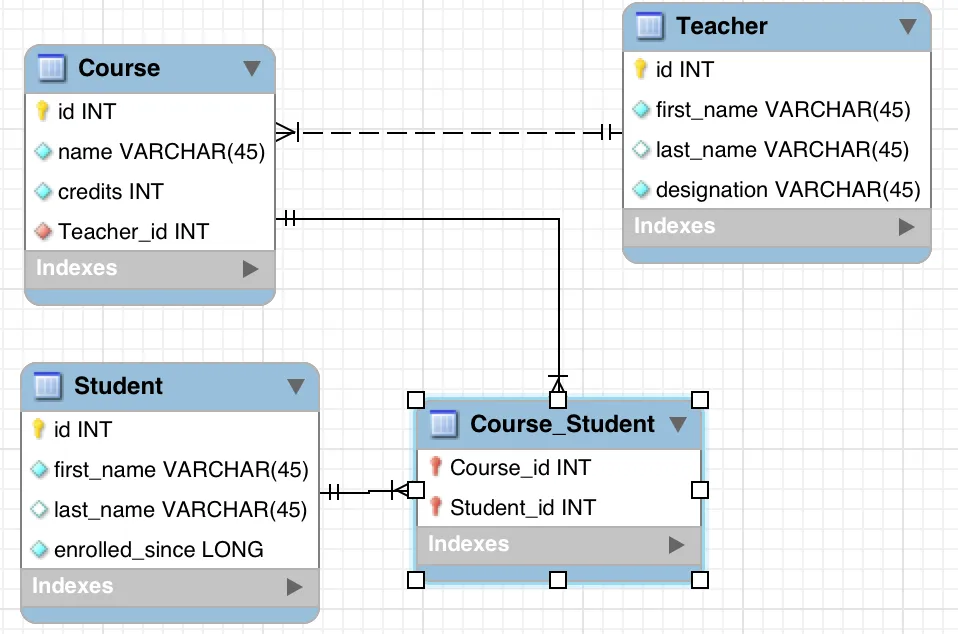
- Select the File | Export | Forward Engineer SQL Create Script... menu.
- On the SQL Export Options page, select checkboxes for two options:
- Generate DROP Statements Before Each CREATE Statement
- Generate DROP SCHEMA
- Specify the Output SQL Script File path if you want to save the script.
- On the last page of the Export wizard, you will see the script generated by MySQL Workbench. Copy this script by clicking the Copy to Clipboard button.
Script to create tables and relationships
-- MySQL Script generated by MySQL Workbench -- Sun Mar 8 18:17:07 2015 -- Model: New Model Version: 1.0 -- MySQL Workbench Forward Engineering SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0; SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0; SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='TRADITIONAL,ALLOW_INVALID_DATES'; -- ----------------------------------------------------- -- Schema course_management -- ----------------------------------------------------- DROP SCHEMA IF EXISTS `course_management` ; -- ----------------------------------------------------- -- Schema course_management -- ----------------------------------------------------- CREATE SCHEMA IF NOT EXISTS `course_management` DEFAULT CHARACTER SET utf8 COLLATE utf8_general_ci ; USE `course_management` ; -- ----------------------------------------------------- -- Table `course_management`.`Teacher` -- ----------------------------------------------------- DROP TABLE IF EXISTS `course_management`.`...