
Getting Started with Haskell Data Analysis
Put your data analysis techniques to work and generate publication-ready visualizations
James Church
- 160 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Getting Started with Haskell Data Analysis
Put your data analysis techniques to work and generate publication-ready visualizations
James Church
About This Book
Put your Haskell skills to work and generate publication-ready visualizations in no time at all
Key Features
- Take your data analysis skills to the next level using the power of Haskell
- Understand regression analysis, perform multivariate regression, and untangle different cluster varieties
- Create publication-ready visualizations of data
Book Description
Every business and organization that collects data is capable of tapping into its own data to gain insights how to improve. Haskell is a purely functional and lazy programming language, well-suited to handling large data analysis problems. This book will take you through the more difficult problems of data analysis in a hands-on manner.
This book will help you get up-to-speed with the basics of data analysis and approaches in the Haskell language. You'll learn about statistical computing, file formats (CSV and SQLite3), descriptive statistics, charts, and progress to more advanced concepts such as understanding the importance of normal distribution. While mathematics is a big part of data analysis, we've tried to keep this course simple and approachable so that you can apply what you learn to the real world.
By the end of this book, you will have a thorough understanding of data analysis, and the different ways of analyzing data. You will have a mastery of all the tools and techniques in Haskell for effective data analysis.
What you will learn
- Learn to parse a CSV file and read data into the Haskell environment
- Create Haskell functions for common descriptive statistics functions
- Create an SQLite3 database using an existing CSV file
- Learn the versatility of SELECT queries for slicing data into smaller chunks
- Apply regular expressions in large-scale datasets using both CSV and SQLite3 files
- Create a Kernel Density Estimator visualization using normal distribution
Who this book is for
This book is intended for people who wish to expand their knowledge of statistics and data analysis via real-world examples. A basic understanding of the Haskell language is expected. If you are feeling brave, you can jump right into the functional programming style.
Frequently asked questions
Information
Descriptive Statistics
programming language. The goal of this book is to take you from being a beginner in math
and statistics, to the point that you feel comfortable working with large-scale datasets.
Now, the prerequisites for this book are that you know a little bit of the Haskell
programming language, and also a little bit of math and statistics. From there, we can start
you on your journey of becoming a data analyst.
- The CSV libraryâworking with CSV files
- Data ranges
- Data mean and standard deviation
- Data median
- Data mode
The CSV library â working with CSV files


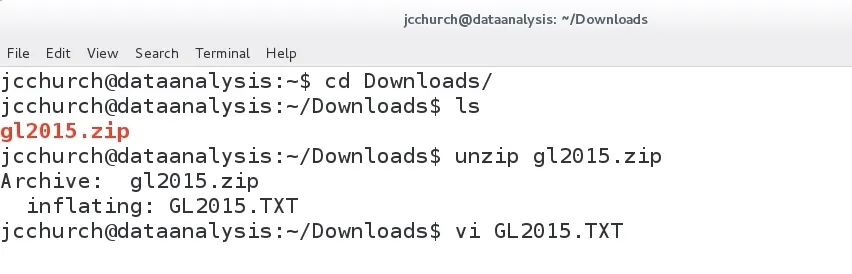



