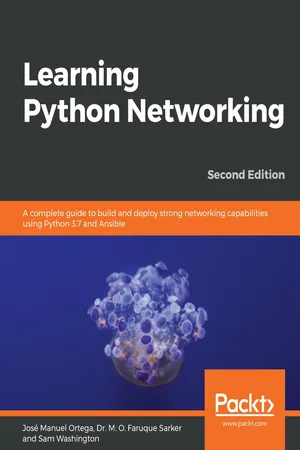
Learning Python Networking
A complete guide to build and deploy strong networking capabilities using Python 3.7 and Ansible , 2nd Edition
José Manuel Ortega, Dr. M. O. Faruque Sarker, Sam Washington
- 490 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Learning Python Networking
A complete guide to build and deploy strong networking capabilities using Python 3.7 and Ansible , 2nd Edition
José Manuel Ortega, Dr. M. O. Faruque Sarker, Sam Washington
About This Book
Achieve improved network programmability and automation by leveraging powerful network programming concepts, algorithms, and tools
Key Features
- Deal with remote network servers using SSH, FTP, SNMP and LDAP protocols.
- Design multi threaded and event-driven architectures for asynchronous servers programming.
- Leverage your Python programming skills to build powerful network applications
Book Description
Network programming has always been a demanding task. With full-featured and well-documented libraries all the way up the stack, Python makes network programming the enjoyable experience it should be.
Starting with a walk through of today's major networking protocols, through this book, you'll learn how to employ Python for network programming, how to request and retrieve web resources, and how to extract data in major formats over the web. You will utilize Python for emailing using different protocols, and you'll interact with remote systems and IP and DNS networking. You will cover the connection of networking devices and configuration using Python 3.7, along with cloud-based network management tasks using Python.
As the book progresses, socket programming will be covered, followed by how to design servers, and the pros and cons of multithreaded and event-driven architectures. You'll develop practical clientside applications, including web API clients, email clients, SSH, and FTP. These applications will also be implemented through existing web application frameworks.
What you will learn
- Execute Python modules on networking tools
- Automate tasks regarding the analysis and extraction of information from a network
- Get to grips with asynchronous programming modules available in Python
- Get to grips with IP address manipulation modules using Python programming
- Understand the main frameworks available in Python that are focused on web application
- Manipulate IP addresses and perform CIDR calculations
Who this book is for
If you're a Python developer or a system administrator with Python experience and you're looking to take your first steps in network programming, then this book is for you. If you're a network engineer or a network professional aiming to be more productive and efficient in networking programmability and automation then this book would serve as a useful resource. Basic knowledge of Python is assumed.
Frequently asked questions
Information
Section 1: Introduction to Network and HTTP Programming
- Chapter 1, Network Programming with Python
- Chapter 2, Programming for the Web with HTTP
Network Programming with Python
- An introduction to TCP/IP networking
- Protocol concepts and the problems that protocols solve
- Addressing
- Creating RESTful web applications and working with flask and HTTP requests
- Interacting flask with the SQLAlchemy database
Technical requirements
An introduction to TCP/IP networking
Introduction to TCP/IP
The protocol stack, layer by layer
- Application layer: This layer manages the high-level protocols, including representation, coding, and dialogue control issues. It handles everything related to applications, and the data is packed appropriately for the next layer. It is a user process that cooperates with other processes on the same host or a different one. Examples of protocols at this layer are TELNET, File Transfer Protocol (FTP), and Simple Mail Transfer Protocol (SMTP).
- Transport layer: This layer handles quality of service, reliability, flow control, and error correction. One of its protocols is the TCP, which provides reliable network communications that are oriented to the connection, unlike UDP, which is not connection oriented. It also provides data transfer. Example protocols include TCP (connection oriented) and UDP (non-connection oriented).
- Network layer: The purpose of the internet layer is to send packets from the source of any network and make them reach their destination, regardless of the route they take to get there.
- Network access layer: This is also called a host-to-host network layer. It includes the LAN and WAN protocols, and the details in the physical and data link layers of the OSI model. Also known as the link layer or data link layer, the network interface layer is the interface to the current network hardware.
