
OCP: Oracle Certified Professional Java SE 8 Programmer II Study Guide
Exam 1Z0-809
Jeanne Boyarsky, Scott Selikoff
- English
- ePUB (mobile friendly)
- Available on iOS & Android
OCP: Oracle Certified Professional Java SE 8 Programmer II Study Guide
Exam 1Z0-809
Jeanne Boyarsky, Scott Selikoff
About This Book
Complete, trusted preparation for the Java Programmer II exam
OCP: Oracle Certified Professional Java SE 8 Programmer II Study Guide is your comprehensive companion forpreparing for Exam1Z0-809as well as upgrade Exam 1Z0-810 and Exam 1Z0-813. With full coverage of 100% of exam objectives, this invaluable guide reinforces what you know, teaches you what you don't know, and gives you the hands-on practice you need to boost your skills. Written by expert Java developers, this book goes beyond mere exam prep with the insight, explanations and perspectives that come from years of experience. You'll review the basics of object-oriented programming, understand functional programming, apply your knowledge to database work, and much more. From the basic to the advanced, this guide walks you through everything you need to know to confidently take the OCP 1Z0-809 Exam andupgrade exams 1Z0-810 and1Z0-813.
Java 8 represents the biggest changes to the language to date, and the latest exam now requires that you demonstrate functional programming competence in order to pass. This guide has you covered, with clear explanations and expert advice.
- Understand abstract classes, interfaces, and class design
- Learn object-oriented design principles and patterns
- Delve into functional programming, advanced strings, and localization
- Master IO, NIO, and JDBC with expert-led database practice
If you're ready to take the next step in your IT career, OCP: Oracle Certified Professional Java SE 8 Programmer II Study Guide is your ideal companion on the road to certification.
Frequently asked questions
Information
Chapter 1
Advanced Class Design
- â Java Class Design
- Implement inheritance including visibility modifiers and composition
- Implement polymorphism
- Override hashCode, equals, and toString methods from Object class
- Develop code that uses the static keyword on initialize blocks, variables, methods, and classes
- â Advanced Java Class Design
- Develop code that uses abstract classes and methods
- Develop code that uses final keyword
- Create inner classes including static inner class, local class, nested class, and anonymous inner class
- Use enumerated types including methods, and constructors in an enum type
- Develop code that declares, implements, and/or extends interface and use the
@Override
annotation
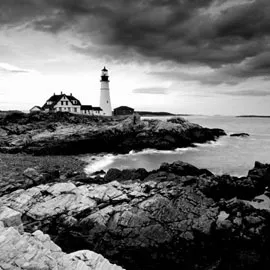
abstract
classes, static
, and final
. Most are implied. For example, the OCP objectives donât mention if
statements and loops. Clearly, you still need to know these. We will also point out differences in Java 8 to help those of you coming in from an older version of Java.instanceof
, implement equals
/hashCode
/toString
, create enumerations, and create nested classes.Reviewing OCA Concepts
Access Modifiers
public
, protected
, and private
and default access. Imagine the following method exists. For now, just remember the instance variables it tries to access:public static void main(String[] args) { BigCat cat = new BigCat(); System.out.println(cat.name); System.out.println(cat.hasFur); System.out.println(cat.hasPaws); System.out.println(cat.id);
main
method that instantiates a BigCat
and tries to print out all four variables. Which variables will be allowed in each case?package cat; public class BigCat { public String name = "cat"; protected boolean hasFur = true; boolean hasPaws = true; private int id; } package cat.species; public class Lynx extends BigCat { } package cat; public class CatAdmirer { } package mouse; public class Mouse { }
BigCat
, it doesnât in all of the classes.cat.name
compiles in all four classes because any code can access public
members. The line with cat.id
compiles only in BigCat
because only code in the same class can access private
members. The line with cat.hasPaws
compiles only in BigCat
and CatAdmirer
because only code in the same package can access code with default access.cat.hasFur
also compiles only in BigCat
and CatAdmirer
. protected
allows subclasses and c...