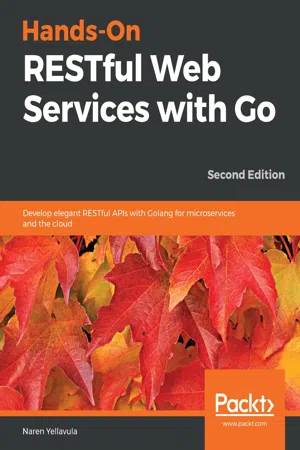
Hands-On RESTful Web Services with Go
Develop elegant RESTful APIs with Golang for microservices and the cloud, 2nd Edition
Naren Yellavula
- 404 páginas
- English
- ePUB (apto para móviles)
- Disponible en iOS y Android
Hands-On RESTful Web Services with Go
Develop elegant RESTful APIs with Golang for microservices and the cloud, 2nd Edition
Naren Yellavula
Información del libro
Design production-ready, testable, and maintainable RESTful web services for the modern web that scale easily
Key Features
- Employ a combination of custom and open source solutions for application program interface (API) development
- Discover asynchronous API and API security patterns and learn how to deploy your web services to the cloud
- Apply design patterns and techniques to build reactive and scalable web services
Book Description
Building RESTful web services can be tough as there are countless standards and ways to develop API. In modern architectures such as microservices, RESTful APIs are common in communication, making idiomatic and scalable API development crucial. This book covers basic through to advanced API development concepts and supporting tools.
You'll start with an introduction to REST API development before moving on to building the essential blocks for working with Go. You'll explore routers, middleware, and available open source web development solutions in Go to create robust APIs, and understand the application and database layers to build RESTful web services. You'll learn various data formats like protocol buffers and JSON, and understand how to serve them over HTTP and gRPC. After covering advanced topics such as asynchronous API design and GraphQL for building scalable web services, you'll discover how microservices can benefit from REST. You'll also explore packaging artifacts in the form of containers and understand how to set up an ideal deployment ecosystem for web services. Finally, you'll cover the provisioning of infrastructure using infrastructure as code (IaC) and secure your REST API.
By the end of the book, you'll have intermediate knowledge of web service development and be able to apply the skills you've learned in a practical way.
What you will learn
- Explore the fundamentals of API development and web services
- Understand the various building blocks of API development in Go
- Use superior open source solutions for representational state transfer (REST) API development
- Scale a service using microservices and asynchronous design patterns
- Deliver containerized artifacts to the Amazon Web Services (AWS) Cloud
- Get to grips with API security and its implementation
Who this book is for
This book is for all the Go developers who are comfortable with the language and seeking to learn REST API development. Even senior engineers can enjoy this book, as it discusses many cutting-edge concepts, such as building microservices, developing API with GraphQL, using protocol buffers, asynchronous API design, and Infrastructure as a Code. Developers who are already familiar with REST concepts and stepping into the Go world from other platforms, such as Python and Ruby, can also benefit a lot.
Preguntas frecuentes
Información
Asynchronous API Design
- Understanding sync/async API requests
- Fan-in/fan-out of services
- Delaying API jobs with queuing
- Long-running task design
- Caching strategies for APIs
- Event-driven API
Technical requirements
- OS: Linux (Ubuntu 18.04)/Windows 10/Mac OS X >=10.13
- Go stable version compiler >= 1.13.5
- Dep: A dependency management tool for Go >= 0.5.3
- Docker version >= 18.09.2
Understanding sync/async API requests


Fan-in/fan-out of services


Delaying API jobs with queuing
- Compress images and email the final result
- Automatic back pressuring (limiting the load on the server to predictable amounts)