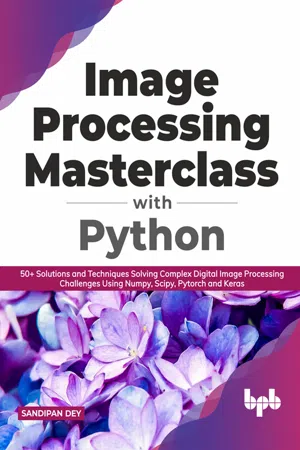
Image Processing Masterclass with Python
50+ Solutions and Techniques Solving Complex Digital Image Processing Challenges Using Numpy, Scipy, Pytorch and Keras
Sandipan Dey
- English
- ePUB (apto para móviles)
- Disponible en iOS y Android
Image Processing Masterclass with Python
50+ Solutions and Techniques Solving Complex Digital Image Processing Challenges Using Numpy, Scipy, Pytorch and Keras
Sandipan Dey
Información del libro
Over 50 problems solved with classical algorithms + ML / DL models
Key Features
- Problem-driven approach to practice image processing.
- Practical usage of popular Python libraries: Numpy, Scipy, scikit-image, PIL and SimpleITK.
- End-to-end demonstration of popular facial image processing challenges using MTCNN and Microsoft's Cognitive Vision APIs.
Description
This book starts with basic Image Processing and manipulation problems and demonstrates how to solve them with popular Python libraries and modules. It then concentrates on problems based on Geometric image transformations and problems to be solved with Image hashing. Next, the book focuses on solving problems based on Sampling, Convolution, Discrete Fourier transform, Frequency domain filtering and image restoration with deconvolution. It also aims at solving Image enhancement problems using different algorithms such as spatial filters and create a super resolution image using SRGAN.Finally, it explores popular facial image processing problems and solves them with Machine learning and Deep learning models using popular python ML / DL libraries.
What you will learn
- Develop strong grip on the fundamentals of Image Processing and Image Manipulation.
- Solve popular Image Processing problems using Machine Learning and Deep Learning models.
- Working knowledge on Python libraries including numpy, scipy and scikit-image.
- Use popular Python Machine Learning packages such as scikit-learn, Keras and pytorch.
- Live implementation of Facial Image Processing techniques such as Face Detection / Recognition / Parsing dlib and MTCNN.
Who this book is for
This book is designed specially for computer vision users, machine learning engineers, image processing experts who are looking for solving modern image processing/computer vision challenges.
Table of Contents
1. Chapter 1: Basic Image & Video Processing
2. Chapter 2: More Image Transformation and Manipulation
3. Chapter 3: Sampling, Convolution and Discrete Fourier Transform
4. Chapter 4: Discrete Cosine / Wavelet Transform and Deconvolution
5. Chapter 5: Image Enhancement
6. Chapter 6: More Image Enhancement
7. Chapter 7: Face Image Processing
About the Author
Sandipan Dey is a Data Scientist with a wide range of interests, covering topics such as Machine Learning, Deep Learning, Image Processing and Computer Vision. He has worked in numerous data science fields, such as recommender systems, predictive models for the events industry, sensor localization models, sentiment analysis, and device prognostics. He earned his master's degree in Computer Science from the University of Maryland, Baltimore County, and has published in a few IEEE data mining conferences and journals. He has also authored a couple of Image Processing books, published from an international publication house. He has earned certifications from 100+ MOOCs on data science and related courses. He is a regular blogger (at sandipanweb @wordpress, medium and data science central) and is a Machine Learning education enthusiast. LinkedIn Profile: https://www.linkedin.com/in/sandipan-dey-0370276
Preguntas frecuentes
Información
CHAPTER 1
Basic Image and Video Processing
Introduction
numpy
, scipy
, scikit-image
, opencv-python
, and matplotlib
) for basic image/video processing, manipulation, and transformation. We shall start by displaying the three channels of an RGB image with 3D visualizations. Next, we shall demonstrate how to capture a video from a camera and extract frames. Then, we shall show how to implement Instagram-like Gotham filter. Finally, we shall explore the following few problems on image manipulations and see how to solve them using python libraries:- Plot image montage, crop/resize images, and draw contours
- Convert PNG image with a palette to grayscale
- Rotate an image and convert RGB to YUV color space (using
scikit-image
,PIL
,python-opencv
, andscipy.ndimage
/misc
)
Structure
- Objectives
- Problems Display RGB image color channels in 3DVideo I/ORead/write video filesCapture video from camera and extract frames with OpenCV-PythonImplement Instagram-like Gotham filterExplore image manipulations (using
scikit-image
,PIL
,python-opencv
, andscipy ndimage
/misc
)Plot image montage withscikit-image
Crop/resize images with SciPyndimage
moduleDraw contours with OpenCV-PythonCounting objects in an imageConvert a PNG image with a palette to grayscale with PILDifferent ways to convert an RGB image to grayscaleRotating an image withscipy.ndimage
Image differences with PILConverting RGB to HSV and YUV color spaces withscikit-image
Resizing an image with OpenCV-PythonAdd a logo to an image withscikit-image
Change brightness/contrast of an image with linear transformation and gamma correction with OpenCV-PythonDetecting colors and changing colors of objects with OpenCV-PythonObject removal with seam carvingCreating fake miniature effect - Summary
- Questions
- Key terms
- References
Objectives
- Understand the image/video storage and data structures in python
- Do image/video file I/O in python using different libraries
- Write python code to do basic image/video manipulations
Problems
Display RGB image color channels in 3D
- First, start by importing all the required packages by using the following code. For reading an image, we need the
imread()
function from thescikit-image
library’sio
module. For array operations, ...