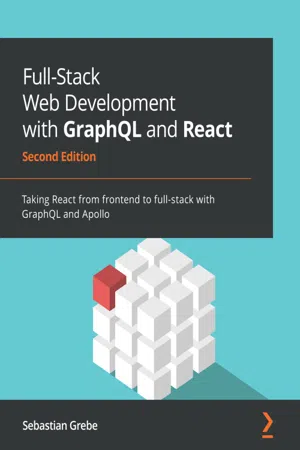
Full-Stack Web Development with GraphQL and React
Sebastian Grebe
- 472 páginas
- English
- ePUB (apto para móviles)
- Disponible en iOS y Android
Full-Stack Web Development with GraphQL and React
Sebastian Grebe
Información del libro
Unleash the power of GraphQL, React 17, Node, and Express to build a scalable and production-ready application from scratch to be deployed on AWSKey Features• Build full-stack applications with modern APIs using GraphQL and React Hooks• Integrate Apollo into React and build frontend components using GraphQL• Implement a self-updating notification pop-up with a unique GraphQL feature called SubscriptionsBook DescriptionReact and GraphQL, when combined, provide you with a very dynamic, efficient, and stable tech stack to build web-based applications. GraphQL is a modern solution for querying an API that represents an alternative to REST and is the next evolution in web development.This book guides you in creating a full-stack web application from scratch using modern web technologies such as Apollo, Express.js, Node.js, and React. First, you'll start by configuring and setting up your development environment. Next, the book demonstrates how to solve complex problems with GraphQL, such as abstracting multi-table database architectures and handling image uploads using Sequelize. You'll then build a complete Graphbook from scratch. While doing so, you'll cover the tricky parts of connecting React to the backend, and maintaining and synchronizing state. In addition to this, you'll also learn how to write Reusable React components and use React Hooks. Later chapters will guide you through querying data and authenticating users in order to enable user privacy. Finally, you'll explore how to deploy your application on AWS and ensure continuous deployment using Docker and CircleCI.By the end of this web development book, you'll have learned how to build and deploy scalable full-stack applications with ease using React and GraphQL.What you will learn• Build a GraphQL API by implementing models and schemas with Apollo and Sequelize• Set up an Apollo Client and build frontend components using React• Write Reusable React components and use React Hooks• Authenticate and query user data using GraphQL• Use Mocha to write test cases for your full-stack application• Deploy your application to AWS using Docker and CircleCIWho this book is forThis React GraphQL book is for web developers familiar with React and GraphQL who want to enhance their skills and build full-stack applications using industry standards like React, Apollo, Node.js, and SQL at scale while learning to solve complex problems with GraphQL.
Preguntas frecuentes
Información
Section 1: Building the Stack
- Chapter 1, Preparing Your Development Environment
- Chapter 2, Setting Up GraphQL with Express.js
- Chapter 3, Connecting to the Database
Chapter 1: Preparing Your Development Environment
- Architecture and technology
- Thinking critically about how to architect a stack
- Building the React and GraphQL stack
- Installing and configuring Node.js
- Setting up a React development environment with webpack, Babel, and other requirements
- Debugging React applications using Chrome DevTools and React Developer Tools