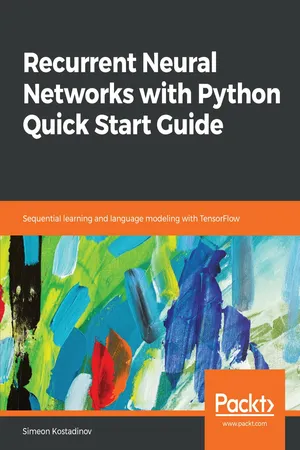
Recurrent Neural Networks with Python Quick Start Guide
Sequential learning and language modeling with TensorFlow
Simeon Kostadinov
- 122 páginas
- English
- ePUB (apto para móviles)
- Disponible en iOS y Android
Recurrent Neural Networks with Python Quick Start Guide
Sequential learning and language modeling with TensorFlow
Simeon Kostadinov
Información del libro
Learn how to develop intelligent applications with sequential learning and apply modern methods for language modeling with neural network architectures for deep learning with Python's most popular TensorFlow framework.
Key Features
- Train and deploy Recurrent Neural Networks using the popular TensorFlow library
- Apply long short-term memory units
- Expand your skills in complex neural network and deep learning topics
Book Description
Developers struggle to find an easy-to-follow learning resource for implementing Recurrent Neural Network (RNN) models. RNNs are the state-of-the-art model in deep learning for dealing with sequential data. From language translation to generating captions for an image, RNNs are used to continuously improve results. This book will teach you the fundamentals of RNNs, with example applications in Python and the TensorFlow library. The examples are accompanied by the right combination of theoretical knowledge and real-world implementations of concepts to build a solid foundation of neural network modeling.
Your journey starts with the simplest RNN model, where you can grasp the fundamentals. The book then builds on this by proposing more advanced and complex algorithms. We use them to explain how a typical state-of-the-art RNN model works. From generating text to building a language translator, we show how some of today's most powerful AI applications work under the hood.
After reading the book, you will be confident with the fundamentals of RNNs, and be ready to pursue further study, along with developing skills in this exciting field.
What you will learn
- Use TensorFlow to build RNN models
- Use the correct RNN architecture for a particular machine learning task
- Collect and clear the training data for your models
- Use the correct Python libraries for any task during the building phase of your model
- Optimize your model for higher accuracy
- Identify the differences between multiple models and how you can substitute them
- Learn the core deep learning fundamentals applicable to any machine learning model
Who this book is for
This book is for Machine Learning engineers and data scientists who want to learn about Recurrent Neural Network models with practical use-cases. Exposure to Python programming is required. Previous experience with TensorFlow will be helpful, but not mandatory.
Preguntas frecuentes
Información
Creating a Spanish-to-English Translator
- Understanding the translation model: This section is entirely focused on the theory behind this system.
- What an LSTM network is: We'll be understanding what sits behind this advanced version of recurrent neural networks.
- Understanding sequence-to-sequence network with attention: You will grasp the theory behind this powerful model, get to know what it actually does, and why it is so widely used for different problems.
- Building the Spanish-to-English translator: This section is entirely focused on implementing the knowledge acquired up to this point in a working program. It includes the following:
- Training the model
- Predicting English translations
- Evaluating the accuracy of the model
Understanding the translation model

- Encode the Spanish sentence, using the encoder RNN, into the intermediate state
- Using that state and the decoder RNN, generate the output sentence in English
What is an LSTM network?





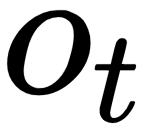
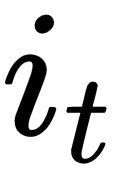

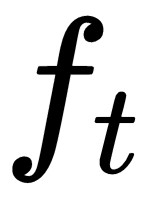

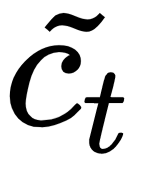
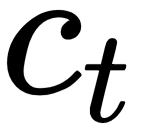



