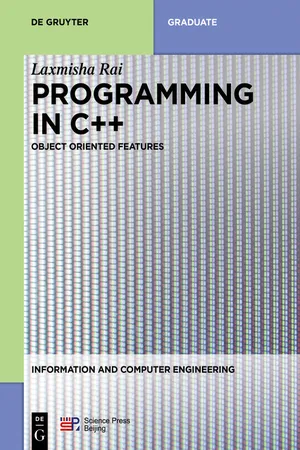
eBook - ePub
Programming in C++
Object Oriented Features
Laxmisha Rai, Laxmisha Rai
This is a test
Compartir libro
- 364 páginas
- English
- ePUB (apto para móviles)
- Disponible en iOS y Android
eBook - ePub
Programming in C++
Object Oriented Features
Laxmisha Rai, Laxmisha Rai
Detalles del libro
Vista previa del libro
Índice
Citas
Información del libro
The book presents an up-to-date overview of C++ programming with object-oriented programming concepts, with a wide coverage of classes, objects, inheritance, constructors, and polymorphism. Selection statements, looping, arrays, strings, function sorting and searching algorithms are discussed. With abundant practical examples, the book is an essential reference for researchers, students, and professionals in programming.
Preguntas frecuentes
¿Cómo cancelo mi suscripción?
¿Cómo descargo los libros?
Por el momento, todos nuestros libros ePub adaptables a dispositivos móviles se pueden descargar a través de la aplicación. La mayor parte de nuestros PDF también se puede descargar y ya estamos trabajando para que el resto también sea descargable. Obtén más información aquí.
¿En qué se diferencian los planes de precios?
Ambos planes te permiten acceder por completo a la biblioteca y a todas las funciones de Perlego. Las únicas diferencias son el precio y el período de suscripción: con el plan anual ahorrarás en torno a un 30 % en comparación con 12 meses de un plan mensual.
¿Qué es Perlego?
Somos un servicio de suscripción de libros de texto en línea que te permite acceder a toda una biblioteca en línea por menos de lo que cuesta un libro al mes. Con más de un millón de libros sobre más de 1000 categorías, ¡tenemos todo lo que necesitas! Obtén más información aquí.
¿Perlego ofrece la función de texto a voz?
Busca el símbolo de lectura en voz alta en tu próximo libro para ver si puedes escucharlo. La herramienta de lectura en voz alta lee el texto en voz alta por ti, resaltando el texto a medida que se lee. Puedes pausarla, acelerarla y ralentizarla. Obtén más información aquí.
¿Es Programming in C++ un PDF/ePUB en línea?
Sí, puedes acceder a Programming in C++ de Laxmisha Rai, Laxmisha Rai en formato PDF o ePUB, así como a otros libros populares de Informatik y Programmierung. Tenemos más de un millón de libros disponibles en nuestro catálogo para que explores.
Información
1 Introduction to Computers and Programming
Opportunities multiply as they are seized.
―Sun Tzu
―Sun Tzu
1.1 History of computers
Computers are used everywhere in the world these days. History of computers goes back to Abacus, a simple calculating device, invented in China around second century BC. We can see this device in China and other countries till date. Many scientists agree that Abacus is the first computer of its kind. Later in 1614, John Napier from Scotland invented the log method that reduces multiplication and division to addition and subtraction, respectively. In 1642, Blaise Pascal, another famous French mathematician, built a mechanical calculator that had the capacity for eight digits. Later, Joseph-Marie Jacquard of France invented an automatic loom controlled by punched cards.
In 1820s, Charles Babbage, the famous English mathematician, invented Difference Engine, but he stopped his work and developed Analytical Engine later. The Analytical Engine is a mechanical computer that can solve mathematical problems by using punched cards. This revolutionary invention of Babbage led to the development of more advanced computers at that time. For his great contribution, Charles Babbage is widely known as the Father of Computers. Many years later, Konrad Zuse, a German engineer, developed the first general-purpose programmable calculator in 1941. In 1945, University of Pennsylvania’s Moore School of Electrical Engineering completed the work of Electronic Numerical Integrator Analyzer and Computer (ENIAC). Later in 1947, Bell Telephone Laboratories developed the transistor. This facilitated a rapid development of computers with greater capability.
After the evolution of digital computing, the computer technology changed rapidly. Many improvements were made in internal organization of computers and computer programming languages. Later, many programming languages were developed to support various applications of computers.
1.2 Introduction to computer and computer science
Computer is a machine that is used to perform calculations. Computer can be programmed to perform many tasks. It can do repetitive tasks quickly and efficiently. It has a large capacity to store, retrieve, and manipulate data. The physical components of a computer are known as hardware. The main components of a computer are: Central Processing Unit (CPU), Input, Output, and Memory. The CPU acts as brain of the computer and executes a program (or software) that instructs the computer about what is to be done. Input and output (I/O) devices allow the computer to communicate with the user and the outside world. Input device is used to receive data and output device is used to display or produce the information. Memory is used to store the data and information.
Sometimes, computer science is viewed as the study of algorithms. This study encompasses four distinct areas:
- Machines for executing algorithms
- Languages for describing algorithms
- Foundations of algorithms
- Analysis of algorithms
An algorithm is a finite set of instructions that, if followed, accomplish a particular task. Moreover, studying algorithms is fundamental to understand computing principles. If you study computing for many years, you will study algorithms of frequently used processes. A number of books have been written about the algorithms for common activities, such as storing and ordering data. Although it is easy to find standard algorithms for those parts of programs that do common activities, one may develop own algorithms for unique problems. Two commonly used tools help to document program logic (the algorithm): flowcharts and pseudocode. Generally, flowcharts work well for small problems, but pseudocode is used for larger problems. Algorithms are written in pseudocode that resembles programming languages such as C or Pascal. Every algorithm must satisfy the following criteria:
- Input: zero or more externally supplied quantities should be there;
- Output: at least one quantity should be produced;
- Definiteness: each instruction must be clear and unambiguous;
- Finiteness: if we trace out the instructions of an algorithm, then for all cases the algorithm will terminate after a finite number of steps; and
- Effectiveness: every instruction must be sufficiently basic, so that it can, in principle, be carried out by a person using only pencil and paper. It is not necessary that each definite operation must also be feasible.
The term algorithm was originally derived from the phonetic pronunciation of the last name of Abu Ja’far Mohammed ibn Musa al-Khowarizmi, who was an Arabic mathematician and had invented a set of rules for performing the four basic arithmetic operations (addition, subtraction, multiplication, and division) on decimal numbers. An algorithm is the representation of a solution to a problem. One of the obstacles to overcome in using a computer to solve our problems is that of translating the idea of the algorithm to computer code (or program). Usually people cannot understand the actual machine code that the computer needs to run a program, so programs are written in a programming language such as C or Pascal, which is then converted into machine code for the computer to run. In the problem-solving phase of computer programming, programmers usually spend much of their time on designing algorithms. This means that students will have to be conscious of the strategies to solve problems in order to apply them to programming problems. These algorithms can be designed by the use of flowcharts or pseudocode.
A flowchart is a diagram made up of boxes, diamonds, and other shapes, connected by arrows, where each shape represents a step in the process, and the arrows show the order in which they occur. Flowcharting combines symbols and flowlines, to show figuratively the operation of an algorithm. From this common understanding, a number of interesting things may happen. For example, process-improvement ideas will often arise spontaneously during a flowcharting session. Moreover, after flowcharting, one may able to write a detailed procedure to solve a problem, which is a good way of documenting a process. Process improvement starts with an understanding of the process, and flowcharting is the first step toward process understanding. Flowcharting uses symbols that have been in use for a number of years to represent the type of operations and/or processes being performed. The standardized format provides a common method for people to visualize problems in the same manner. The use of standardized symbols makes the flowcharts easier to interpret; however, the sequence of activities that make up the process is more important. Note that the pseudocode also describes the essential steps to be taken, but without graphical enhancements. In the recent years, a general-purpose, developmental modeling language known as Unified Modeling Language (UML) was popularly used to visualize the design of a system.
A program is a set of quite a number of commands. It goes through many steps during its development. A typical program development may have the following steps:
- Requirement Analysis: In this step, a programmer tries to understand what the program is supposed to do?
- Program Design: After understanding the requirements, the programmer draws conclusions about how to do it. In this step, the programmer creates a strategy to achieve the goal. One of the techniques used during the design phase is drawing a flowchart of the program. In the flowchart, different shapes represent different kinds of steps, such as input and output, decisions, or calculations.
- Coding: In this step, the programmer writes the program in a chosen language.
- Testing: The programmer tests the program against the requirements of Step 1.
- Documentation: An important part of any program development is documentation. It is a good practice to have documents for every program we write. The documents explain other readers what we did, why we did it, and how we did it.
1.3 Introduction to software
The computer hardware includes physical devices such as keyboard, mouse, monitor, etc. The software includes a set of programs. Software allows us to perform various tasks by using computer. These tasks include word processing, database computations, programming, etc. Two common software types are system software and application software. System software is responsible for controlling, integrating, and managing the individual hardware components of a computer system. The operating system is the most important system software that generally interacts with the computer hardware. The application software is used to perform some specific applications such as drawing software, imaging software, etc.
Software is developed by using programming languages. There are many types of programming languages such as, interpreter or compiler-based languages. Software can be developed by using any of these types of languages depending on the application. In general, software is a collection of programs and routines that work together to perform a specific operation. A program is simply a set of instructions written in a particular language.

Over the years, the development of software is becoming increasingly complex. To guide the software development process, a number of software-based tools are developed. In general, there are five main steps during the development of software, which become major part of Software Development Life Cycle (SDLC). The main goals of SDLC are to reduce and minimize the risks involved in development. The software project planning, organizing, and maintenance are based on SDLC models. Some of the important and popular SDLC models are waterfall model, iterative model, spiral model, fountain model, V-model, and big bang model.
The simplest, most straightforward, and oldest SDLC model is the waterfall model. In this model, a number of activities are organized in a sequential manner. As shown in Fig. 1.1, each activity is followed after completion of the previous activity:
- Requirements gathering (or analysis)
- Design
- Coding (or implementation)
- Testing
- Delivery or maintenance
In software testing, verification and validation (V&V) is the process of checking that a software system meets the requirement specifications. The validation tests answer the question: Are we building the right software? On the other hand, the verification tests answer the following question: Are we building the software right?
Although waterfall model is simple, and easy to understand, it has a few disadvantages. For example:
- Delays are highly probable in this model, because there is a little scope for revision once a phase is completed.
- Moreover, any problems in the intermediate phases cannot be fixed until we reach the maintenance stage.
Logical improvement to the waterfall model resulted in the fountain model. In a fountain, water rises up to the middle and then falls back, either to the pool below or is re-entrained at an intermediate level. The same activities as those in the software development of the waterfall model are used in fountain model in the same sequence. However, there is an overlap and iteration of activities. The fountain model is a graphical representation to remind us that although some life cycle activities cannot start before others, there is a considerable overlap and merging of activities across the full life cycle.
Another flexible model in SDLC is the spiral model that emphasizes the need to revisit the earlier stages as many number of times as the project progresses. This allows multiple rounds of refinement. This takes the cues from iterative model and repetition of activities in...