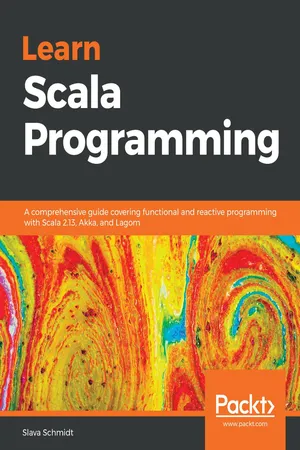
Learn Scala Programming
A comprehensive guide covering functional and reactive programming with Scala 2.13, Akka, and Lagom
Slava Schmidt
- 498 pagine
- English
- ePUB (disponibile sull'app)
- Disponibile su iOS e Android
Learn Scala Programming
A comprehensive guide covering functional and reactive programming with Scala 2.13, Akka, and Lagom
Slava Schmidt
Informazioni sul libro
A step-by-step guide in building high-performance scalable applications with the latest features of Scala.
Key Features
- Develop a strong foundation in functional programming and Scala's Standard Library (STL)
- Get a detailed coverage of Lightbend Lagom—the latest microservices framework from Lightbend
- Understand the Akka framework and learn event-based Programming with Scala
Book Description
The second version of Scala has undergone multiple changes to support features and library implementations. Scala 2.13, with its main focus on modularizing the standard library and simplifying collections, brings with it a host of updates.
Learn Scala Programming addresses both technical and architectural changes to the redesigned standard library and collections, along with covering in-depth type systems and first-level support for functions. You will discover how to leverage implicits as a primary mechanism for building type classes and look at different ways to test Scala code. You will also learn about abstract building blocks used in functional programming, giving you sufficient understanding to pick and use any existing functional programming library out there. In the concluding chapters, you will explore reactive programming by covering the Akka framework and reactive streams.
By the end of this book, you will have built microservices and learned to implement them with the Scala and Lagom framework.
What you will learn
- Acquaint yourself with the new standard library of Scala 2.13
- Get to grips with the Grok functional paradigms
- Get familiar with type system to express domain constraints
- Understand the actor model and different Akka libraries
- Grasp the concept of building microservices using Lagom framework
- Deep dive into property-based testing and its practical applications
Who this book is for
This book is for beginner to intermediate level Scala developers who would like to advance and gain knowledge of the intricacies of the Scala language, expand their functional programming tools, and explore actor-based concurrency models.
Domande frequenti
Informazioni
Project 1 - Building Microservices with Scala
- Essentials of microservices
- Purely functional HTTP APIs with http4s
- Purely functional database access with doobie
- API integration testing with Http1Client
- Reactive HTTP API with Akka-HTTP
- Event-sourced persistent state with Akka Persistence
Technical requirements
- SBT 1.2+
- Java 1.8+
Essentials of microservices
- Codebase and technological stack: The code of the service should not be shared with other services.
- Deployment: The service is deployed independently of other services both in terms of time and underlying infrastructure.
- State: The service has its own persistent store and the only way for other services to access the data is by calling the owning service.
- Failure-handling: Microservices are expected to be resilient. In the case of failures of downstream services, the one in question is expected to isolate failure.
- Continuous delivery even for very complex applications
- The complexity of each service is low because it is limited to a single business capability
- Independent deployment implies independent scalability for services with different loads
- Code-independence enables polyglot environments and makes the adoption of new technologies easier
- Teams can be scaled down in size, which reduces communication overhead and speeds up decision-making
- Unavailability of habitual transactions
- Debugging, testing, and tracing calls involving multiple microservices
- The complexity shifts from the individual service into the space between them
- Service location and protocol discovery require lots of effort