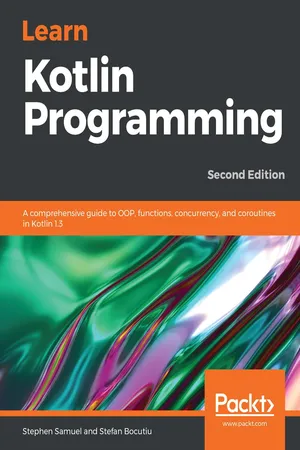
Learn Kotlin Programming
A comprehensive guide to OOP, functions, concurrency, and coroutines in Kotlin 1.3, 2nd Edition
Stephen Samuel, Stefan Bocutiu
- 514 pagine
- English
- ePUB (disponibile sull'app)
- Disponibile su iOS e Android
Learn Kotlin Programming
A comprehensive guide to OOP, functions, concurrency, and coroutines in Kotlin 1.3, 2nd Edition
Stephen Samuel, Stefan Bocutiu
Informazioni sul libro
Delve into the world of Kotlin and learn to build powerful Android and web applications
Key Features
- Learn the fundamentals of Kotlin to write high-quality code
- Test and debug your applications with the different unit testing frameworks in Kotlin
- Explore Kotlin's interesting features such as null safety, reflection, and annotations
Book Description
Kotlin is a general-purpose programming language used for developing cross-platform applications. Complete with a comprehensive introduction and projects covering the full set of Kotlin programming features, this book will take you through the fundamentals of Kotlin and get you up to speed in no time.
Learn Kotlin Programming covers the installation, tools, and how to write basic programs in Kotlin. You'll learn how to implement object-oriented programming in Kotlin and easily reuse your program or parts of it. The book explains DSL construction, serialization, null safety aspects, and type parameterization to help you build robust apps. You'll learn how to destructure expressions and write your own. You'll then get to grips with building scalable apps by exploring advanced topics such as testing, concurrency, microservices, coroutines, and Kotlin DSL builders. Furthermore, you'll be introduced to the kotlinx.serialization framework, which is used to persist objects in JSON, Protobuf, and other formats.
By the end of this book, you'll be well versed with all the new features in Kotlin and will be able to build robust applications skillfully.
What you will learn
- Explore the latest Kotlin features in order to write structured and readable object-oriented code
- Get to grips with using lambdas and higher-order functions
- Write unit tests and integrate Kotlin with Java code
- Create real-world apps in Kotlin in the microservices style
- Use Kotlin extensions with the Java collections library
- Uncover destructuring expressions and find out how to write your own
- Understand how Java-nullable code can be integrated with Kotlin features
Who this book is for
If you're a beginner or intermediate programmer who wants to learn Kotlin to build applications, this book is for you. You'll also find this book useful if you're a Java developer interested in switching to Kotlin.
Domande frequenti
Informazioni
Section 1: Fundamental Concepts in Kotlin
- Chapter 1, Getting Started with Kotlin
- Chapter 2, Kotlin Basics
- Chapter 3, Object-Oriented Programming in Kotlin
Getting Started with Kotlin
- Use the command line to compile and execute code written in Kotlin
- Use the REPL and write Kotlin scripts
- Create a Gradle project with Kotlin enabled
- Create a Maven project with Kotlin enabled
- Use IntelliJ to create a Kotlin project
- Use Eclipse IDE to create a Kotlin project
- Mix Kotlin and Java code in the same project
Technical requirements
Using the command line to compile and run Kotlin code
$ curl -s https://get.sdkman.io | bash $ bash $ sdk install kotlin 1.3.31
$ brew update
$ brew install [email protected]
fun main(args: Array<String>) { println("Hello, World!") }
kotlinc HelloWorld.kt -include-runtime -d HelloWorld.jar
$ java -jar HelloWorld.jar
$ kotlinc HelloWorld.kt
$ javap -c HelloWorldKt.class
Compiled from "HelloWorld.kt" public final class HelloWorldKt { public static final void main(java.lang.String[]); Code: 0: aload_0 1: ldc #9 // String args 3: invokestatic #15 // Method kotlin/jvm/internal/Intrinsics.checkParameterIsNotNull:(Ljava/lang/Ob ject;Ljava/lang/String;)V 6: ldc #17 // String Hello, World! 8: astore_1 9: nop 10: getstatic #23 // Field java/lang/System.out:Ljava/io/PrintStream; 13: aload_1 14: invokevirtual #29 // Method java/io/PrintStream.println:(Ljava/lang/Object;)V 17: return }
Kotlin runtime
$ kotlinc HelloWorld.kt -d HelloWorld.jar $ java -jar HelloWorld.jar Exception in thread "main" java.lang.NoClassDefFoundError: kotlin/jvm/internal/Intrinsics at HelloWorldKt.main(HelloWorld.kt) Caused by: java.lang.ClassNotFoundException: kotlin.jvm.internal.Intrinsics
$ java -cp $KOTLIN_HOME/lib/kotlin-runtime.jar:HelloWorld.jar HelloWorldKt
$ kotlinc -include-runtime HelloWorld.kt -d HelloW...