
eBook - ePub
Professional Node.js
Building Javascript Based Scalable Software
Pedro Teixeira
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Professional Node.js
Building Javascript Based Scalable Software
Pedro Teixeira
Book details
Book preview
Table of contents
Citations
About This Book
Learn to build fast and scalable software in JavaScript with Node.js
Node.js is a powerful and popular new framework for writing scalable network programs using JavaScript. This no nonsense book begins with an overview of Node.js and then quickly dives into the code, core concepts, and APIs. In-depth coverage pares down the essentials to cover debugging, unit testing, and flow control so that you can start building and testing your own modules right away.
- Covers node and asynchronous programming main concepts
- Addresses the basics: modules, buffers, events, and timers
- Explores streams, file systems, networking, and automated unit testing
- Goes beyond the basics, and shares techniques and tools for debugging, unit testing, and flow control
If you already know JavaScript and are curious about the power of Node.js, then this is the ideal book for you.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Professional Node.js an online PDF/ePUB?
Yes, you can access Professional Node.js by Pedro Teixeira in PDF and/or ePUB format, as well as other popular books in Informatica & Programmazione in JavaScript. We have over one million books available in our catalogue for you to explore.
Information
Part I
Introduction and Setup
- CHAPTER 1: Installing Node
- CHAPTER 2: Introducing Node
Chapter 1
Installing Node
WHATâS IN THIS CHAPTER?
- Getting Node up and running
- Installing Node Package Manager (NPM)
- Using NPM to install, uninstall, and update packages
At the European JSConf in 2009, Ryan Dahl, a young programmer, presented a project he had been working on. This project was a platform that combined Googleâs V8 JavaScript engine, an event loop, and a low-level I/O API. This project was not like other server-side JavaScript platforms where all the I/O primitives were event-driven and there was no way around it. By leveraging the power and simplicity of JavaScript, this project turned the difficult task of writing event-driven server-side applications into an easy one. The project received a standing ovation and has since then been met with unprecedented growth, popularity, and adoption.
The project was named Node.js and is now known to developers simply as Node. Node provides a purely event-driven, non-blocking infrastructure for building highly concurrent software.
NOTE Node allows you to easily construct fast and scalable network services.
Ever since its introduction, Node has received attention from some of the biggest players in the industry. They have used Node to deploy networked services that are fast and scalable. Node is so attractive for several reasons.
One reason is JavaScript. JavaScript is the most widely used programming language on the planet. Most web programmers are used to writing JavaScript in the browser, and the server is a natural extension of that.
The other reason is Nodeâs simplicity. Nodeâs core functionalities are kept to a minimum and all the existing APIs are quite elegant, exposing the minimum amount of complexity to the programmers. When you want to build something more complex, you can easily pick, install, and use several of the available third-party modules.
Another reason Node is attractive is because of how easy it is to get started using it. You can download and install it very easily and then get it up and running in a matter of minutes.
The typical way to install Node on your development machine is by following the steps on the http://nodejs.org website. Node installs out of the box on Windows, Linux, Macintosh, and Solaris.
INSTALLING NODE ON WINDOWS
Node supports the Windows operating system since version 0.6.0. To install Node on Windows, point your browser to http://nodejs.org/#download and download the node-v*.msi Windows installer by clicking on the link. You should then be prompted with a security dialog box, as shown in Figure 1-1.
FIGURE 1-1
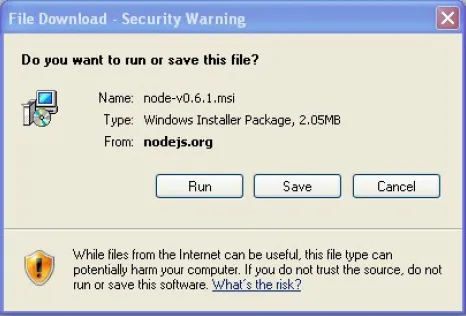
Click on the Run button, and you will be prompted with another security dialog box asking for confirmation. If you agree, the Node install wizard begins (see Figure 1-2).
FIGURE 1-2
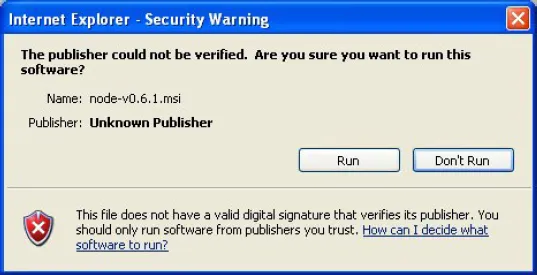
When you click Run, the Installation Wizard starts (see Figure 1-3).
FIGURE 1-3
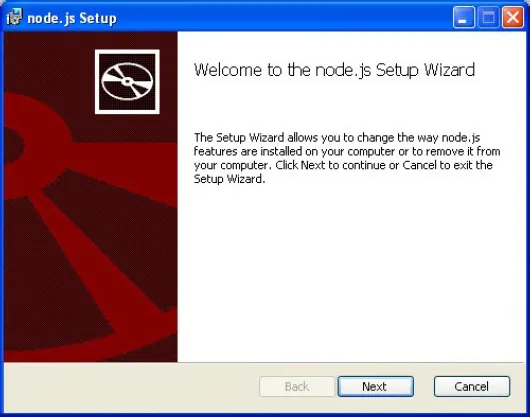
Click on the Next button and Node will start installing. A few moments later you will get the confirmation that Node was installed (see Figure 1-4).
FIGURE 1-4
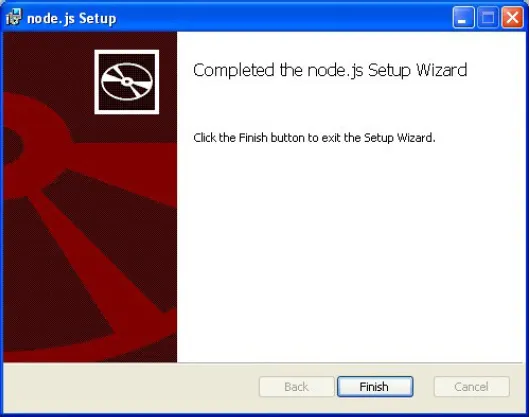
INSTALLING ON MAC OS X
If you use Mac OS X you can install Node using an Install Wizard. To start, head to http://nodejs.org/#download and download the node-v*.pkg Macintosh installer by clicking on the link. Once the download is finished, click on the downloaded file to run it. You will then get the first wizard dialog box, as seen in Figure 1-5.
FIGURE 1-5
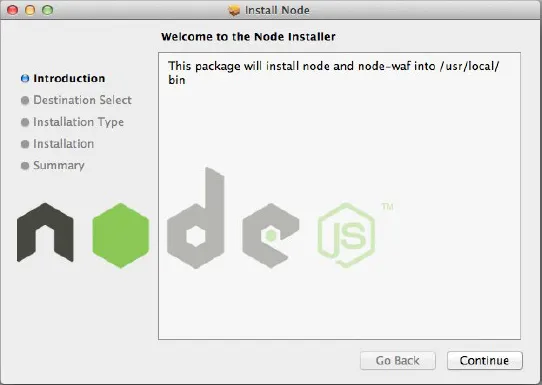
Choose to continue and install. The wizard will then ask you for the system user password, after which the installation will start. A few seconds later youâll get the confirmation window stating that Node is installed on your system (see Figure 1-6).
FIGURE 1-6
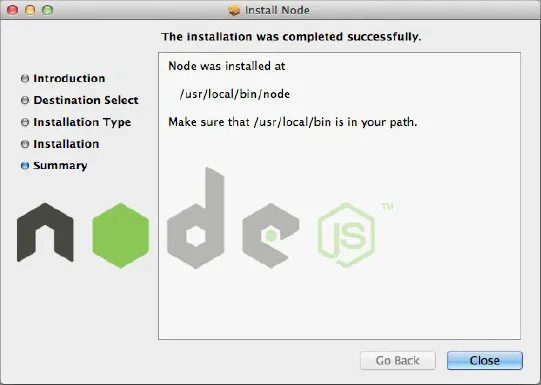
INSTALLING NODE USING THE SOURCE CODE
If you have a UNIX system, you can install Node by compiling the source code. First you need to select which version of Node you will be installing, then you will download the source code and build, install, and run Node.
NOTE Node depends on several third-party code libraries, but fortunately most of them are already distributed along with the program. If you are building from source code, you should need only two things:
- python (version 2.4 or higher) â The build tools distributed with Node run on python.
- libssl-dev â If you plan to use SSL/TLS encryption in your networking, youâll need this. libssl is the library used in the openssl tool. On Linux and UNIX systems it can usually be installed with your favorite package manager. The libssl library comes pre-installed on Mac OS X.
Choosing the Node Version
Two different versions of Node are usually available for download on the nodejs.org website: the latest stable and the latest unstable.
In Node, the minor version numbering denotes the stability of the version. Stable versions have an even minor version (0.2, 0.4, 0.6), and unstable versions have an odd minor version (0.1, 0.3, 0.5, 0.7).
Not only might an unstable version be functionally unstable, but the API might also be mutating. The stable versions should not change the public API. For each stable branch, a new patch should include only bug fixes, whereas APIs sometimes change in the unstable branch.
Unless you want to test a new feature that is only available in the latest unstable release, you should always choose the latest stable version. The unstable versions are a battleground for the Node Core Team to test new developments in the platform.
More and more projects and companies successfully use Node in production (some of the most relevant are on the nodejs.org home page), but you might have to put some effort into keeping up with the API changes on each new minor stable release. Thatâs the price of using a new technology.
Downloading the Node Source Code
After you choose a version to download, copy the source code tarball URL from the http://nodejs.org website and download it. If youâre running in a UNIX system, you probably have wget installed, which means that you can download it by using a shell prompt and typing the following:
$ wget http://nodejs.org/dist/v0.6.1/node-v0.6.12.tar.gz
If you donât have wget installed, you may also use the curl utility:
$ curl -O http://nodejs.org/dist/v0.6.1/node-v0.6.12.tar.gz
If you donât have either of these tools installed, you have to find another way to download the tarball file into your local directory â such as by using a browser or transferring it via the local network.
(The examples in this book use the latest stable version at the time of writing, which is 0.6.12.)
Building Node
Now that you have the source code in your computer, you can build the Node executable. First you need to unpack the source files like this:
$ tar xfz node-v0.6.12.tar.gz
Then step into the source directory:
$ cd node-v0.6.12
Configure it:
$ ./configure
You should get a successful output like this:
'config...