
eBook - ePub
Beginning C# 3.0
An Introduction to Object Oriented Programming
Jack Purdum
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Beginning C# 3.0
An Introduction to Object Oriented Programming
Jack Purdum
Book details
Book preview
Table of contents
Citations
About This Book
Learn all the basics of C# 3.0 from Beginning C# 3.0: An Introduction to Object Oriented Programming, a book that presents introductory information in an intuitive format. If you have no prior programming experience but want a thorough, easy-to-understand introduction to C# and Object Oriented Programming, this book is an ideal guide. Using the tutorials and hands-on coding examples, you can discover tried and true tricks of the trade, understand design concepts, employ debugging aids, and design and write C# programs that are functional and that embody safe programming practices.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Beginning C# 3.0 an online PDF/ePUB?
Yes, you can access Beginning C# 3.0 by Jack Purdum in PDF and/or ePUB format, as well as other popular books in Informatik & Programmierung in C#. We have over one million books available in our catalogue for you to explore.
Information
Part I
Getting Started
Chapter 1: Getting Started
Chapter 2: Understanding Objects
Chapter 1
Getting Started
Welcome to the world of object-oriented programming and C#! The primary goal of this book is to use the C# programming language from Microsoft to teach you object-oriented programming, or OOP. This book assumes that you have no prior programming experience in any language and that you know nothing about OOP.
If you do have programming experience and some familiarity with OOP, thatâs fine. Having that experience makes things easier for you. However, I still encourage you to read the book from start to finish for a number of reasons. First, this book represents the distillation of 25 years of programming and teaching experience. I have a good idea of what works and what doesnât work when it comes to explaining complex topics so that theyâre easy to understand. Reading each chapter gives you the tools to understand the next chapter. Second, I may introduce topics in one chapter and then rely heavily on those topics in a much later chapter. In other words, the process used to learn OOP and C# is one that introduces new topics based upon ones that were introduced earlier. Obviously, itâs important to master the earlier content before tackling the later content. Finally, the programming examples I use also build on concepts presented in earlier program examples. It will be easier for you to understand the later program examples if youâve experimented with those programs introduced earlier in the book.
One more thing: You cannot learn programming by just reading about it. You have to dig in and start programming yourself. For that reason, there are exercises at the end of each chapter designed to help you hone your programming skills. The learning process is even more interesting if you try to create your own programs based upon some real problems youâd like to solve. Donât worry if things donât fall together instantly on the first try. You should plan to make a ton of âflat-foreheadâ mistakes . . . you know, the kind of mistake where, upon discovering it, you pound the heel of your hand into your forehead and say: âHow could I make such a stupid mistake!â Not to worry . . . weâve all been there. Such mistakes are just part of the process of becoming a programmer and you should expect to make your fair share. However, stick with it, read the book, and youâll be surprised at how fast things will come together. Indeed, I think youâll find programming to be a truly enjoyable pastime.
In this chapter, you will learn about
- Downloading Visual Studio .NETâs C# Express
- Installing C# Express
- Testing C# Express to ensure it was installed correctly
With that in mind, letâs get started.
A Short History of Object-Oriented Programming (OOP)
Many people believe that OOP is a product of the 1980s and the work done by Bjarne Stroustrup in moving the C language into the object-oriented world by creating the C++ language. Actually, SIMULA 1 (1962) and Simula 67 (1967) are the two earliest object-oriented languages. The work on the Simula languages was done by Ole-John Dahl and Kristen Nygaard at the Norwegian Computing Center in Oslo, Norway. While most of the advantages of OOP were available in the earlier Simula languages, it wasnât until C++ became entrenched in the 1990s that OOP began to flourish.
C was the parent language of C++ and it was often said that C was powerful enough to shoot yourself in the foot multiple times. C++, on the other hand, not only was powerful enough to shoot yourself in the foot, but you could blow your entire leg off without too much difficulty. Most programmers admit that C++ is a very powerful language and it is still in widespread use today. However, with that power comes a lot of complexity. Language developers wanted a simpler and perhaps less complex language for OOP development.
The next step in the development of OOP started in January of 1991 when James Gosling, Bill Joy, Patrick Naughton, Mike Sheradin, and several others met in Aspen, Colorado, to discuss ideas for the Stealth Project. The group wanted to develop intelligent electronic devices capable of being centrally controlled and programmed from a handheld device. They decided that OOP was the right direction to go with the development language, but felt that C++ was not up to the job. The result was the Oak programming language (named after an oak tree outside Goslingâs window), which eventually morphed into the Java programming language. (Oak had to be renamed because the team discovered that a language by that name already existed.)
Java quickly grew in popularity, spurred by the growth of the World Wide Web. In both cases this rapid growth was in part due to the fact that the âgutsâ necessary to run Java programs on the Web quickly became an integral part of various web browsers. With the improved Web functionality augmented by Java, the Web hit light speed.
To many programmers, C# is Microsoftâs answer to Java. Some would even say that C# is the result of Microsoftâs stubbornness in refusing to promote a language it did not develop. That sentiment is a bit too harsh. Microsoft had good reasons for developing C#, not the least of which was that it wanted what are known as type-safe programs that run in a managed environment. Youâre not ready to appreciate exactly what that means right now, but it will become clear as you learn C#.
Suffice it to say that C# provides you with a robust object-oriented programming language and an impressive set of tools to tackle almost any programming task. Whether you wish to develop desktop, distributed, web, or mobile applications, C# can handle the task.
As you become familiar with C#, you will appreciate its relatively few keywords, its crisp syntax, and its easy-to-use development environment. Youâll discover that pieces of programs you write in C# can be reused in other programs. Finally, you might appreciate the fact that there are many job opportunities for programmers who know C#. (In fact, in the writerâs locality as this text is being written, there are more job openings for C# programmers than in any other language.)
Installing C#
If you have already purchased and installed Visual Studio 2008 and C#, you can skip this section. If you havenât installed C#, this section tells you how to download and install the C# Express version of Visual Studio. C# Express is a modified version of C# that is available from Microsoft at no charge. While the Express version of C# is missing some features found in the commercial version of Visual Studio, you should be able to compile and run all the sample programs in this book using C# Express. Once you are convinced that you should do all your development work in C# (and you will be), you can purchase the full version of Visual Studio.
Downloading C# Express
At the time that this book is being written, you can go to: http://msdn2.microsoft.com/en-us/express/future/bb421473.aspx to download C# Express. The web page looks similar to what is shown in Figure 1-1. As you can see if you look closely at the figure, the book was written using Visual C# Express Edition Beta 2. (By the time you read this book, it is quite likely that the âBeta 2â part of the title will have changed.) Now click the IMG file link to download the file.
Figure 1-1
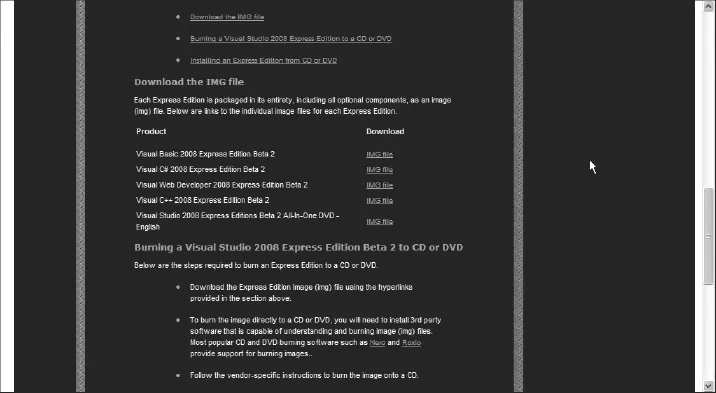
Depending upon the speed of your Internet connection, the file should be saved on your system within a few minutes.
Installing C# Express
After the download completes, click the executable file that was supplied (the file was named vcssetup.exe when I installed it, but it could change). You should see a screen similar to that shown in Figure 1-2, the C# Express Edition install...