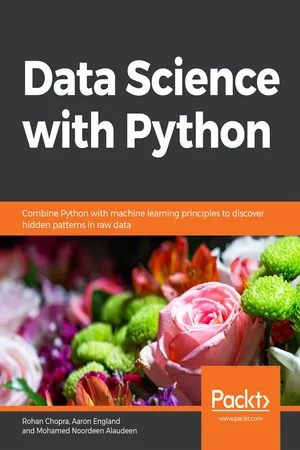
Data Science with Python
Combine Python with machine learning principles to discover hidden patterns in raw data
Rohan Chopra, Aaron England, Mohamed Noordeen Alaudeen
- 426 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Data Science with Python
Combine Python with machine learning principles to discover hidden patterns in raw data
Rohan Chopra, Aaron England, Mohamed Noordeen Alaudeen
About This Book
Leverage the power of the Python data science libraries and advanced machine learning techniques to analyse large unstructured datasets and predict the occurrence of a particular future event.
Key Features
- Explore the depths of data science, from data collection through to visualization
- Learn pandas, scikit-learn, and Matplotlib in detail
- Study various data science algorithms using real-world datasets
Book Description
Data Science with Python begins by introducing you to data science and teaches you to install the packages you need to create a data science coding environment. You will learn three major techniques in machine learning: unsupervised learning, supervised learning, and reinforcement learning. You will also explore basic classification and regression techniques, such as support vector machines, decision trees, and logistic regression.
As you make your way through chapters, you will study the basic functions, data structures, and syntax of the Python language that are used to handle large datasets with ease. You will learn about NumPy and pandas libraries for matrix calculations and data manipulation, study how to use Matplotlib to create highly customizable visualizations, and apply the boosting algorithm XGBoost to make predictions. In the concluding chapters, you will explore convolutional neural networks (CNNs), deep learning algorithms used to predict what is in an image. You will also understand how to feed human sentences to a neural network, make the model process contextual information, and create human language processing systems to predict the outcome.
By the end of this book, you will be able to understand and implement any new data science algorithm and have the confidence to experiment with tools or libraries other than those covered in the book.
What you will learn
- Pre-process data to make it ready to use for machine learning
- Create data visualizations with Matplotlib
- Use scikit-learn to perform dimension reduction using principal component analysis (PCA)
- Solve classification and regression problems
- Get predictions using the XGBoost library
- Process images and create machine learning models to decode them
- Process human language for prediction and classification
- Use TensorBoard to monitor training metrics in real time
- Find the best hyperparameters for your model with AutoML
Who this book is for
Data Science with Python is designed for data analysts, data scientists, database engineers, and business analysts who want to move towards using Python and machine learning techniques to analyze data and predict outcomes. Basic knowledge of Python and data analytics will prove beneficial to understand the various concepts explained through this book.
Frequently asked questions
Information
Chapter 1
Introduction to Data Science and Data Pre-Processing
Learning Objectives
- Use various Python machine learning libraries
- Handle missing data and deal with outliers
- Perform data integration to bring together data from different sources
- Perform data transformation to convert data into a machine-readable form
- Scale data to avoid problems with values of different magnitudes
- Split data into train and test datasets
- Describe the different types of machine learning
- Describe the different performance measures of a machine learning model
Introduction
Python Libraries
Note
Roadmap for Building Machine Learning Models
- Data Pre-processingThis is the first step in building a machine learning model. Data pre-processing refers to the transformation of data before feeding it into the model. It deals with the techniques that are used to convert unusable raw data into clean reliable data.Since data collection is often not performed in a controlled manner, raw data often contains outliers (for example, age = 120), nonsensical data combinations (for example, model: bicycle, type: 4-wheeler), missing values, scale problems, and so on. Because of this, raw data cannot be fed into a machine learning model because it might compromise the quality of the results. As such, this is the most important step in the process of data science.
- Model LearningAfter pre-processing the data and splitting it into train/test sets (more on this later), we move on to modeling. Models are nothing but sets of well-defined methods called algorithms that use pre-processed data to learn patterns, which can later be used to make predictions. There are different types of learning algorithms, including supervised, semi-supervised, unsupervised, and reinforcement learning. These will be discussed later.
- Model EvaluationIn this stage, the models are evaluated with the help of specific performance metrics. With these metrics, we can go on to tune the hyperparameters of a model in order to improve it. This process is called hyperparameter optimization. We will repeat this step until we are satisfied with the performance.
- PredictionOnce we are happy with the results from the evaluation step, we will then move on to predictions. Predictions are made by the trained model when it is exposed to a new dataset. In a business setting, these predictions can be shared with decision makers to make effective business choices.
- Model Deployment The whole...