
Getting Started with Web Components
Build modular and reusable components using HTML, CSS and JavaScript
Prateek Jadhwani
- 158 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Getting Started with Web Components
Build modular and reusable components using HTML, CSS and JavaScript
Prateek Jadhwani
About This Book
Explore modern Web Component design and integrate them with a variety of web frameworks to build encapsulated reusable UI components for your web apps
Key Features
- Learn Web Components with more than 50 web component examples for both beginners and advanced users
- Create responsive and highly customizable web pages using HTML, CSS, and JavaScript
- Extend the potential of Web Components by integrating them with standard web frameworks
Book Description
Web Components are a set of APIs that help you build reusable UI modules that can operate in any modern browser using just Vanilla JavaScript. The power of Web Components lies in their ability to build frontend web applications with or without web frameworks.
With this practical guide, you will understand how Web Components can help you build reusable UI components for your modern web apps. The book starts by explaining the fundamentals of Web Components' design and strategies for using them in your existing frontend web projects. You will also learn how to use JavaScript libraries such as Polymer.js and Stencil.js for building practical components. As you progress, you will build a single-page application using only Web Components to fully realize their potential. This practical guide demonstrates how to work with Shadow DOM and custom elements to build the standard components of a web application. Toward the end of the book, you will learn how to integrate Web Components with standard web frameworks to help you manage large-scale web applications.
By the end of this book, you will have learned about the capabilities of Web Components in building custom elements and have the necessary skills for building a reusable UI for your web applications.
What you will learn
- Understand Web Component design, specifications, and life cycle
- Create single-page applications using Web Components
- Enable reusability and customization for your UI components
- Implement Web Components in your web apps using Polymer and Stencil libraries
- Build powerful frontend components from scratch and deploy them on the web
- Design patterns and best practices to integrate Web Components into your existing web application
Who this book is for
This book is for developers who have heard about web components, but don't really know where to start. This book is also for intermediate and advanced developers who know what web components are, but are still afraid to use them in production. This book is also for frontend engineers who are simply looking into web components in order to increase their knowledge and skills.
Frequently asked questions
Information
Building a Single Page App using Web Components
- Understanding project requirements
- Figuring out reusable Web Components
- Configuring starter project and APIs
- App components
- Other components
- Implementing routing
- Enabling analytics
Understanding project requirements
Figuring out reusable Web Components

- Header component: A header that can be used on all pages. It needs to be sticky on the top, and clicking on the links should change the URL.
- GIF cover component: A component that takes a URL as an attribute and shows it. It can also have a height limit.
- Search bar component: A component that is responsible for getting input from a user and searching for a string with the help of APIs. And when the search is complete, it returns the results with the help of a custom event.
- Search container: A component that will have a Search bar component inside it, and will show GIF cover components based on the result obtained by the Search bar.

- Header component: Same as previously
- GIF cover: The same component that we used on the last page to show GIFs
- Show Trending component: The container component that will make the call to the API to get trending GIFs and create a collection of GIF Cover components
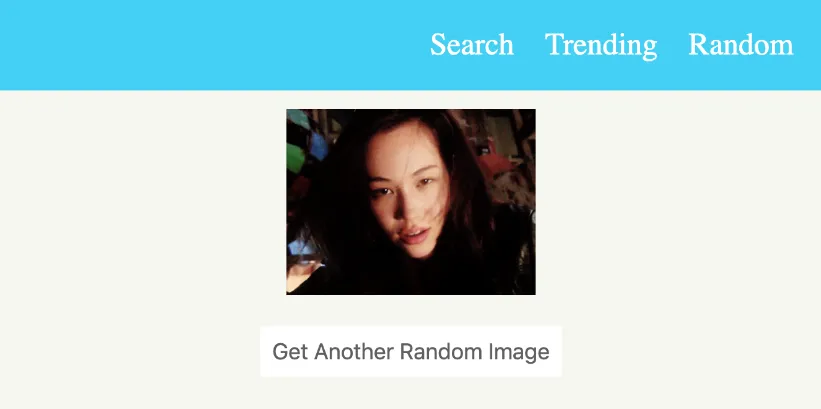
- Header component: Same as previously.
- GIF cover: Same as the last one, but we won't be seeing a lot of them.
- Show Random component: A component that is responsible for making the API call to get a random GIF. It also needs to have a button that needs to trigger the API again when it is clicked.
Configuring the Starter Project and APIs
Pre-requisites
Setting up the project
cd Chapter\ 06/Starter\ Project/
npm install