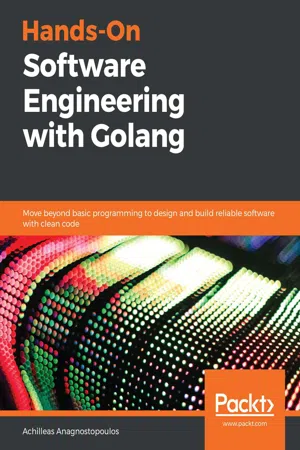
Hands-On Software Engineering with Golang
Move beyond basic programming to design and build reliable software with clean code
Achilleas Anagnostopoulos
- 640 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-On Software Engineering with Golang
Move beyond basic programming to design and build reliable software with clean code
Achilleas Anagnostopoulos
About This Book
Explore software engineering methodologies, techniques, and best practices in Go programming to build easy-to-maintain software that can effortlessly scale on demand
Key Features
- Apply best practices to produce lean, testable, and maintainable Go code to avoid accumulating technical debt
- Explore Go's built-in support for concurrency and message passing to build high-performance applications
- Scale your Go programs across machines and manage their life cycle using Kubernetes
Book Description
Over the last few years, Go has become one of the favorite languages for building scalable and distributed systems. Its opinionated design and built-in concurrency features make it easy for engineers to author code that efficiently utilizes all available CPU cores.
This Golang book distills industry best practices for writing lean Go code that is easy to test and maintain, and helps you to explore its practical implementation by creating a multi-tier application called Links 'R' Us from scratch. You'll be guided through all the steps involved in designing, implementing, testing, deploying, and scaling an application. Starting with a monolithic architecture, you'll iteratively transform the project into a service-oriented architecture (SOA) that supports the efficient out-of-core processing of large link graphs. You'll learn about various cutting-edge and advanced software engineering techniques such as building extensible data processing pipelines, designing APIs using gRPC, and running distributed graph processing algorithms at scale. Finally, you'll learn how to compile and package your Go services using Docker and automate their deployment to a Kubernetes cluster.
By the end of this book, you'll know how to think like a professional software developer or engineer and write lean and efficient Go code.
What you will learn
- Understand different stages of the software development life cycle and the role of a software engineer
- Create APIs using gRPC and leverage the middleware offered by the gRPC ecosystem
- Discover various approaches to managing package dependencies for your projects
- Build an end-to-end project from scratch and explore different strategies for scaling it
- Develop a graph processing system and extend it to run in a distributed manner
- Deploy Go services on Kubernetes and monitor their health using Prometheus
Who this book is for
This Golang programming book is for developers and software engineers looking to use Go to design and build scalable distributed systems effectively. Knowledge of Go programming and basic networking principles is required.
Frequently asked questions
Information
Section 1: Software Engineering and the Software Development Life Cycle
- Chapter 1, A Bird's-Eye View of Software Engineering
A Bird's-Eye View of Software Engineering
- A definition of software engineering
- The types of software engineering roles that you may encounter in contemporary organizations
- An overview of popular software development models and which one to select based on the project type and requirements
What is software engineering?
- What are the business use cases that the software needs to support?
- What components comprise the system and how do they interact with each other?
- Which technologies will be used to implement the various system components?
- How will the software be tested to ensure that its behavior matches the customer's expectations?
- How does load affect the system's performance and what is the plan for scaling the system?
Types of software engineering roles
The role of the software engineer (SWE)
- Junior engineer: A junior engineer is someone who has recently started their software development career and lacks the necessary experience to build and deploy production-grade software. Companies are usually keen on hiring junior engineers as it allows them to keep their hiring costs low. Furthermore, companies often pair promising junior engineers with senior engineers in an attempt to grow them into mid-level engineers and retain them for longer.
- Mid-level engineer: A typical mid-level engineer is someone who has at least three years of software development experience. Mid-level engineers are expected to have a solid grasp of the various aspects of the software development life cycle and are the ones who can exert a significant impact on the amount of code that's produced for a particular project. To this end, they not only contribute code, but also review and offer feedback to the code that's contributed by other team members.
- Senior engineer: This class of engineer is well-versed in a wide array of disparate technologies; their breadth of knowledge makes them ideal for assembling and managing software engineering teams, as well as serving as mentors and coaches for less senior engineers. From their years of experience, senior engineers acquire a deep understanding of a particular business domain. This trait allows them to serve as a liaison between their teams and the other, technical or non-technical, business stakeholders.
- Frontend engineers work exclusively on software that customers interact with. Examples of frontend work include the UI for a desktop application, a single-page web application for a software as a service (SaaS) offering, and a mobile application running on a phone or other smart device.
- Backend engineers specialize in building the parts of a system that implement the actual business logic and deal with data modeling, validation, storage, and retrieval.
- Full stack engineers are developers who have a good understanding of both frontend and backend technologies and no particular preference of doing frontend or backend work. This class of developers is more versatile as they can easily move between teams, depending on the project requirements.