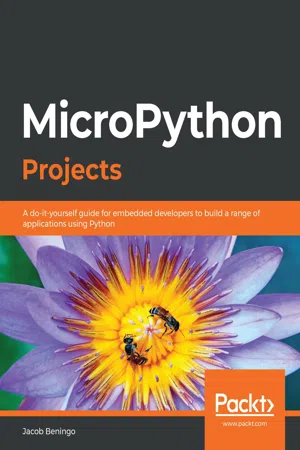
MicroPython Projects
A do-it-yourself guide for embedded developers to build a range of applications using Python
Jacob Beningo
- 294 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
MicroPython Projects
A do-it-yourself guide for embedded developers to build a range of applications using Python
Jacob Beningo
About This Book
Explore MicroPython through a series of hands-on projects and learn to design and build your own embedded systems using the MicroPython Pyboard, ESP32, the STM32 IoT Discovery kit, and the OpenMV camera module.
Key Features
- Delve into MicroPython Kernel and learn to make modifications that will enhance your embedded applications
- Design and implement drivers to interact with a variety of sensors and devices
- Build low-cost projects such as DIY automation and object detection with machine learning
Book Description
With the increasing complexity of embedded systems seen over the past few years, developers are looking for ways to manage them easily by solving problems without spending a lot of time on finding supported peripherals. MicroPython is an efficient and lean implementation of the Python 3 programming language, which is optimized to run on microcontrollers. MicroPython Projects will guide you in building and managing your embedded systems with ease.
This book is a comprehensive project-based guide that will help you build a wide range of projects and give you the confidence to design complex projects spanning new areas of technology such as electronic applications, automation devices, and IoT applications. While building seven engaging projects, you'll learn how to enable devices to communicate with each other, access and control devices over a TCP/IP socket, and store and retrieve data. The complexity will increase progressively as you work on different projects, covering areas such as driver design, sensor interfacing, and MicroPython kernel customization.
By the end of this MicroPython book, you'll be able to develop industry-standard embedded systems and keep up with the evolution of the Internet of Things.
What you will learn
- Develop embedded systems using MicroPython
- Build a custom debugging tool to visualize sensor data in real-time
- Detect objects using machine learning and MicroPython
- Discover how to minimize project costs and reduce development time
- Get to grips with gesture operations and parsing gesture data
- Learn how to customize and deploy the MicroPython kernel
- Explore the techniques for scheduling application tasks and activities
Who this book is for
If you are an embedded developer or hobbyist looking to build interesting projects using MicroPython, this book is for you. A basic understanding of electronics and Python is required while some MicroPython experience will be helpful.
Frequently asked questions
Information
Customizing the MicroPython Kernel Start Up Code
- An overview of the MicroPython kernel
- Navigating the startup code
- Modifying the default GPIO initialization
- Adding MicroPython modules to the kernel
- Testing the results
Technical requirements
- A Linux machine or virtual machine
- An STM32L4 IoT Discovery node
- A RobotDyn I2C 8-bit PCA8574 I/O expander module, or equivalent
- An Adafruit RGB pushbutton PN: 3423, or equivalent
- A breadboard
- 6" jumpers
- A generic two-position switch
- A 30-gauge wire-wrapping wire
- A Terminal application (PuTTY, RealTerm, Terminal, or one of many others)
- A text editor, such as Sublime Text
An overview of the MicroPython kernel
Downloading the MicroPython kernel
sudo apt-get install git
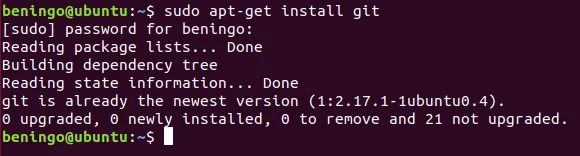
sudo clone https://github.com/micropython/micropython.git
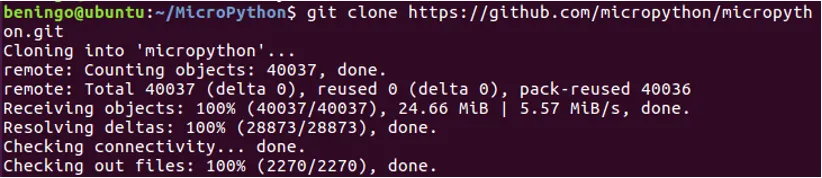
MicroPython kernel organization

Folder | Description |
docs | Contains documentation for different major ports. |
drivers | Contains external device drivers for items, such as displays, memory devices, radios, and SD cards. |
examples | Contains example Python scripts. |
extmod | Contains additional (non-core) modules that are implemented in C, such as crypto and filesystems. |
mpy-cross | The MicroPython cross-compiler, which generates bytecode from scripts. |
ports | Contains all the different architecture ports supported by MicroPython. |
py | The Python implementation, which includes the Python core, compiler, libraries, and runtime. |
tests | Contains the test framework for MicroPython. |
tools | Contains scripts that can be useful for developing the MicroPython kernel. |
Becoming familiar with the STM32L475SE_IOT01A port
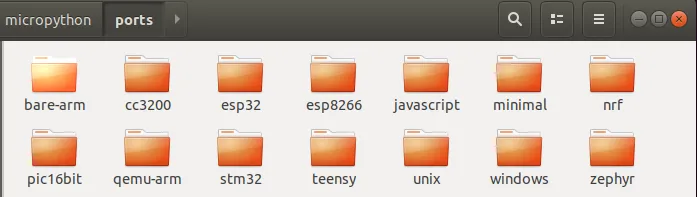
- Supported board folders, which are all the different boards supported by MicroPython
- STM32 derivative linker files, which define the memory maps for the different processors
- STM32 derivative pin maps, which describe what each pin does on the processor
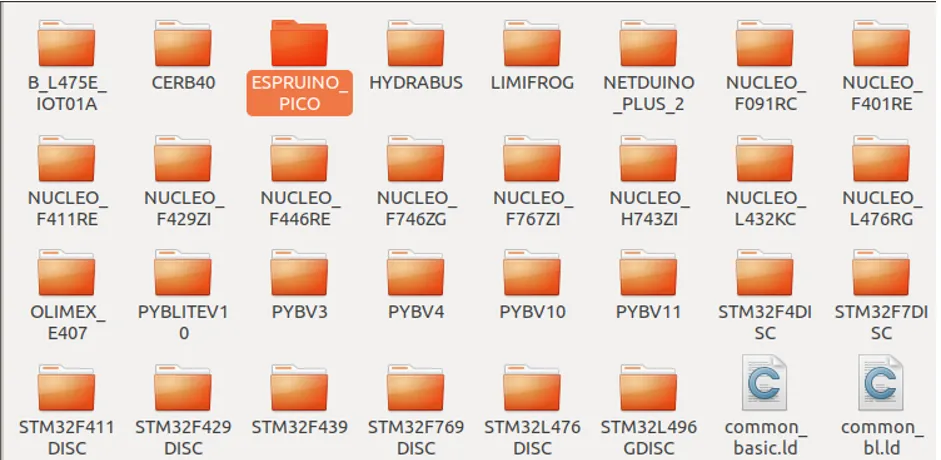