
Learn C# Programming
A guide to building a solid foundation in C# language for writing efficient programs
Marius Bancila, Raffaele Rialdi, Ankit Sharma
- 636 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Learn C# Programming
A guide to building a solid foundation in C# language for writing efficient programs
Marius Bancila, Raffaele Rialdi, Ankit Sharma
About This Book
Get started with C# and strengthen your knowledge of core programming concepts such as procedural, object-oriented, generic, functional, and asynchronous programming along with the latest features of C# 8
Key Features
- Learn the fundamentals of C# with the help of easy-to-follow examples and explanations
- Leverage the latest features of C# 8, including nullable reference types, pattern matching enhancements, and asynchronous streams
- Explore object-oriented programming, functional programming, and multithreading concepts
Book Description
The C# programming language is often developers' primary choice for creating a wide range of applications for desktop, cloud, and mobile. In nearly two decades of its existence, C# has evolved from a general-purpose, object-oriented language to a multi-paradigm language with impressive features.
This book will take you through C# from the ground up in a step-by-step manner. You'll start with the building blocks of C#, which include basic data types, variables, strings, arrays, operators, control statements, and loops. Once comfortable with the basics, you'll then progress to learning object-oriented programming concepts such as classes and structures, objects, interfaces, and abstraction. Generics, functional programming, dynamic, and asynchronous programming are covered in detail. This book also takes you through regular expressions, reflection, memory management, pattern matching, exceptions, and many other advanced topics. As you advance, you'll explore the.NET Core 3 framework and learn how to use the dotnet command-line interface (CLI), consume NuGet packages, develop for Linux, and migrate apps built with.NET Framework. Finally, you'll understand how to run unit tests with the Microsoft unit testing frameworks available in Visual Studio.
By the end of this book, you'll be well-versed with the essentials of the C# language and be ready to start creating apps with it.
What you will learn
- Get to grips with all the new features of C# 8
- Discover how to use attributes and reflection to build extendable applications
- Utilize LINQ to uniformly query various sources of data
- Use files and streams and serialize data to JSON and XML
- Write asynchronous code with the async-await pattern
- Employ.NET Core tools to create, compile, and publish your applications
- Create unit tests with Visual Studio and the Microsoft unit testing frameworks
Who this book is for
If you have little experience in coding or C# and want to learn the essentials of C# programming to develop powerful programming techniques, this book is for you. It will also help aspiring programmers to write scripts or programs to accomplish specific tasks.
Frequently asked questions
Information
Chapter 1: Starting with the Building Blocks of C#
- Learning the history of C#
- Understanding the CLI
- Knowing the .NET family of frameworks
- Assemblies in .NET
- Understanding the basic structure of a C# program
The history of C#


Understanding the CLI
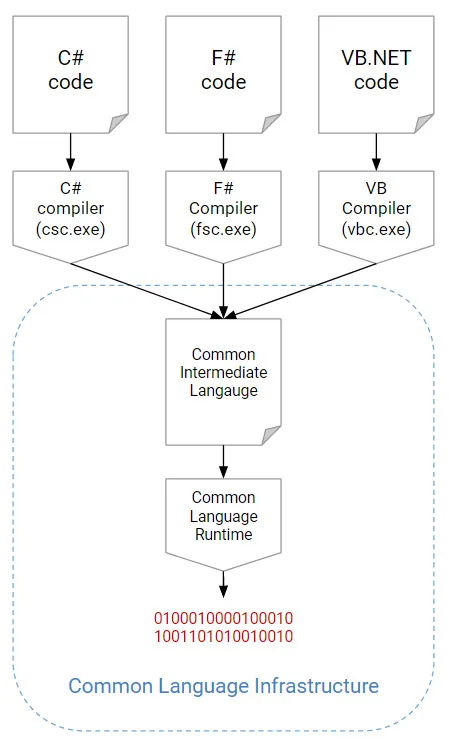
Common Type System (CTS)
- It enables cross-platform integration, type safety, and high-performance code execution.
- It provides an object-oriented model that supports the complete implementation of many programming languages.
- It provides rules for languages to ensure that objects and data types of objects written in different programming languages can interact with each other.
- It defines rules for type visibility and access to members.
- It defines rules for type inheritance, virtual methods, and object lifetime.
- Value types: These contain their data directly and have copy semantics, which means when an object of such a type is copied its data is copied.
- Reference types: These contain references to the memory address where the data is stored. When an object of a reference type is copied, the reference is copied and not the data it points to.
Common Language Specification (CLS)
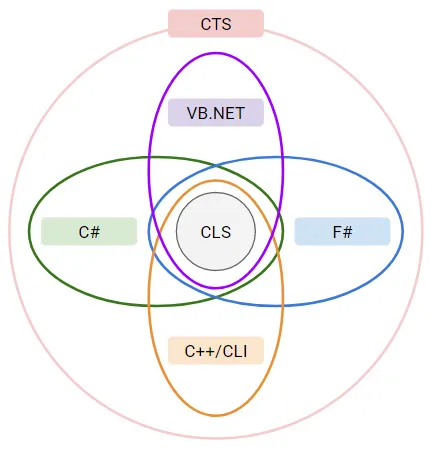