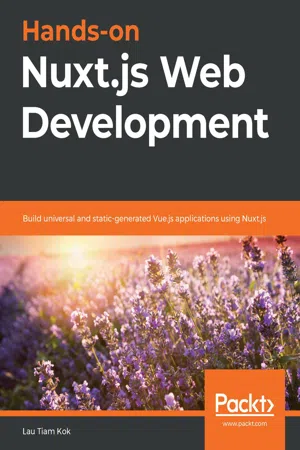
Hands-on Nuxt.js Web Development
Build universal and static-generated Vue.js applications using Nuxt.js
Lau Tiam Kok
- 698 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-on Nuxt.js Web Development
Build universal and static-generated Vue.js applications using Nuxt.js
Lau Tiam Kok
About This Book
Learn Nuxt.js for building server-side rendered, static-generated, and production-ready Vue.js web applications with the help of practical examples
Key Features
- Explore techniques for authentication, testing, and deployment to build your first complete Nuxt.js web app
- Write cleaner, maintainable, and scalable isomorphic JavaScript web applications
- Transform your Vue.js application into universal and static-generated web apps
Book Description
Nuxt.js is a progressive web framework built on top of Vue.js for server-side rendering (SSR). With Nuxt.js and Vue.js, building universal and static-generated applications from scratch is now easier than ever before.
This book starts with an introduction to Nuxt.js and its constituents as a universal SSR framework. You'll learn the fundamentals of Nuxt.js and find out how you can integrate it with the latest version of Vue.js. You'll then explore the Nuxt.js directory structure and set up your first Nuxt.js project using pages, views, routing, and Vue components. With the help of practical examples, you'll learn how to connect your Nuxt.js application with the backend API by exploring your Nuxt.js application's configuration, plugins, modules, middleware, and the Vuex store. The book shows you how you can turn your Nuxt.js application into a universal or static-generated application by working with REST and GraphQL APIs over HTTP requests. Finally, you'll get to grips with security techniques using authorization, package your Nuxt.js application for testing, and deploy it to production.
By the end of this web development book, you'll have developed a solid understanding of using Nuxt.js for your projects and be able to build secure, end-to-end tested, and scalable web applications with SSR, data handling, and SEO capabilities.
What you will learn
- Integrate Nuxt.js with the latest version of Vue.js
- Extend your Vue.js applications using Nuxt.js pages, components, routing, middleware, plugins, and modules
- Create a basic real-time web application using Nuxt.js, Node.js, Koa.js and RethinkDB
- Develop universal and static-generated web applications with Nuxt.js, headless CMS and GraphQL
- Build Node.js and PHP APIs from scratch with Koa.js, PSRs, GraphQL, MongoDB and MySQL
- Secure your Nuxt.js applications with the JWT authentication
- Discover best practices for testing and deploying your Nuxt.js applications
Who this book is for
The book is for any JavaScript or full-stack developer who wants to build server-side rendered Vue.js apps. A basic understanding of the Vue.js framework will assist with understanding key concepts covered in the book.
Frequently asked questions
Information
- Chapter 1, Introducing Nuxt
- Chapter 2, Getting Started with Nuxt
- Chapter 3, Adding UI Frameworks
- From Vue to Nuxt
- Why Use Nuxt?
- Types of applications
- Nuxt as a universal SSR app
- Nuxt as a static site generator
- Nuxt as a single-page app
From Vue to Nuxt
- Vue (https://vuejs.org/)
- Vue Router (https://router.vuejs.org/)
- Vuex (https://vuex.vuejs.org/)
- Vue Server Renderer (https://ssr.vuejs.org/)
- Vue Meta (https://vue-meta.nuxtjs.org/)
- Vue Loader (https://vue-loader.vuejs.org/)
- Babel Loader (https://webpack.js.org/loaders/babel-loader/)
Why use Nuxt?
Writing single-file components
Vue.component('todo-item', {...})
const TodoItem = {...}
// pages/index.vue
<template>
<p>{{ message }}</p>
</template>
<script>
export default {
data () {
return { message: 'Hello World' }
}
}
</script>
<style scoped>
p {
font-size: 2em;
text-align: center;
}
</style>
Writing ES2015+
// pages/about.vue
<script>
export default {
async asyncData ({ params, error }) {
//...
}
}
</script>
Writing CSS with a preprocessor
$ npm i less --save-dev
$ npm i less-loader --save-dev
Table of contents
- Title Page
- Copyright and Credits
- Dedication
- About Packt
- Contributors
- Preface
- Section 1: Your First Nuxt App
- Introducing Nuxt
- Getting Started with Nuxt
- Adding UI Frameworks
- Section 2: View, Routing, Components, Plugins, and Modules
- Adding Views, Routes, and Transitions
- Adding Vue Components
- Writing Plugins and Modules
- Adding Vue Forms
- Section 3: Server-Side Development and Data Management
- Adding a Server-Side Framework
- Adding a Server-Side Database
- Adding a Vuex Store
- Section 4: Middleware and Security
- Writing Route Middlewares and Server Middlewares
- Creating User Logins and API Authentication
- Section 5: Testing and Deployment
- Writing End-to-End Tests
- Using Linters, Formatters, and Deployment Commands
- Section 6: The Further Fields
- Creating an SPA with Nuxt
- Creating a Framework-Agnostic PHP API for Nuxt
- Creating a Real-Time App with Nuxt
- Creating a Nuxt App with a CMS and GraphQL
- Other Books You May Enjoy