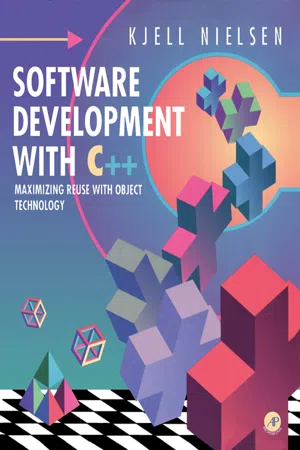
Software Development with C++
Maximizing Reuse with Object Technology
Kjell Nielsen
- 474 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Software Development with C++
Maximizing Reuse with Object Technology
Kjell Nielsen
About This Book
Software Development with C++: Maximizing Reuse with Object Technology is about software development and object-oriented technology (OT), with applications implemented in C++. The basis for any software development project of complex systems is the process, rather than an individual method, which simply supports the overall process. This book is not intended as a general, all-encompassing treatise on OT. The intent is to provide practical information that is directly applicable to a development project. Explicit guidelines are offered for the infusion of OT into the various development phases. The book is divided into five major parts. Part I describes why we need a development process, the phases and steps of the software process, and how we use individual methods to support this process. Part II lays the foundation for the concepts included in OT. Part III describes how OT is used in the various phases of the software development process, including the domain analysis, system requirements analysis, system design, software requirements analysis, software design, and implementation. Part IV deals exclusively with design issues for an anticipated C++ implementation. Part V is devoted to object-oriented programming with C++. This book is intended for practicing software developers, software managers, and computer science and software engineering students. Sufficient guidelines are included to aid project leaders in establishing an overall development process for small, medium, and large system applications.
Frequently asked questions
Information
Introduction to The Software Development Process
Introduction
Publisher Summary
1.1 WHY DO WE NEED A DEVELOPMENT PROCESS?
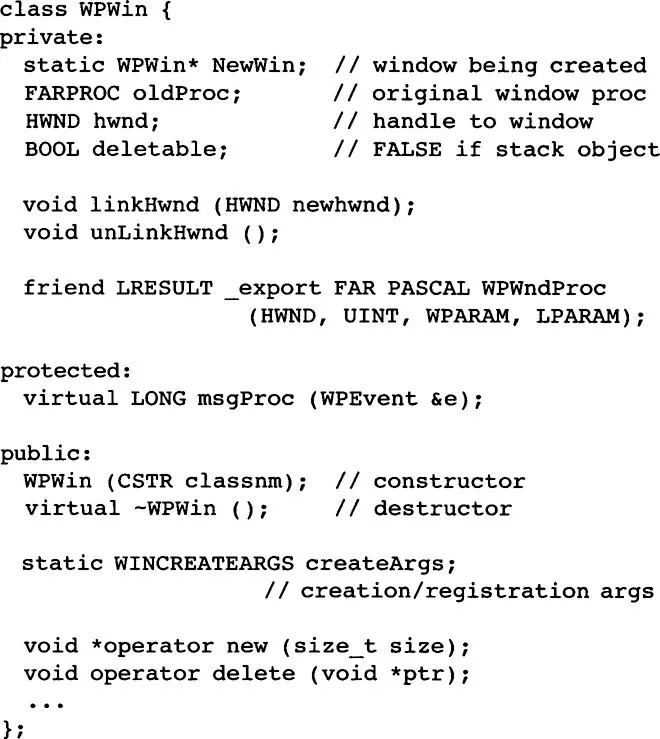