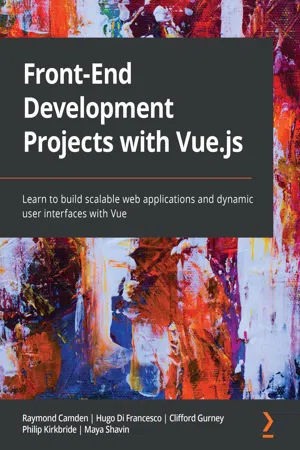
Front-End Development Projects with Vue.js
Learn to build scalable web applications and dynamic user interfaces with Vue
Raymond Camden, Hugo Di Francesco, Clifford Gurney, Philip Kirkbride, Maya Shavin
- 774 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Front-End Development Projects with Vue.js
Learn to build scalable web applications and dynamic user interfaces with Vue
Raymond Camden, Hugo Di Francesco, Clifford Gurney, Philip Kirkbride, Maya Shavin
About This Book
Get to grips with the core fundamentals of Vue.js 2 and learn to build reliable component-based applications with practical guidance from industry experts
Key Features
- Learn how to make the best use of the Vue.js 2 framework and build a full end-to-end project
- Build dynamic components and user interfaces that are fast and intuitive
- Write performant code that "just works" and is easily scalable and reusable
Book Description
Are you looking to use Vue 2 for web applications, but don't know where to begin? Front-End Development Projects with Vue.js will help build your development toolkit and get ready to tackle real-world web projects. You'll get to grips with the core concepts of this JavaScript framework with practical examples and activities.
Through the use-cases in this book, you'll discover how to handle data in Vue components, define communication interfaces between components, and handle static and dynamic routing to control application flow. You'll get to grips with Vue CLI and Vue DevTools, and learn how to handle transition and animation effects to create an engaging user experience. In chapters on testing and deploying to the web, you'll gain the skills to start working like an experienced Vue developer and build professional apps that can be used by other people.
You'll work on realistic projects that are presented as bitesize exercises and activities, allowing you to challenge yourself in an enjoyable and attainable way. These mini projects include a chat interface, a shopping cart and price calculator, a to-do app, and a profile card generator for storing contact details.
By the end of this book, you'll have the confidence to handle any web development project and tackle real-world front-end development problems.
What you will learn
- Set up a development environment and start your first Vue 2 project
- Modularize a Vue application using component hierarchies
- Use external JavaScript libraries to create animations
- Share state between components and use Vuex for state management
- Work with APIs using Vuex and Axios to fetch remote data
- Validate functionality with unit testing and end-to-end testing
- Get to grips with web app deployment
Who this book is for
This book is designed for Vue.js beginners. Whether this is your first JavaScript framework, or if you're already familiar with React or Angular, this book will get you on the right track. To understand the concepts explained in this book, you must be familiar with HTML, CSS, JavaScript, and Node package management.
Frequently asked questions
Information
1. Starting Your First Vue Project
Introduction
Angular versus Vue
React versus Vue
Advantages of Using Vue for Your Project
- Vue.js is another example of a pattern in development that is easy to learn but ha...