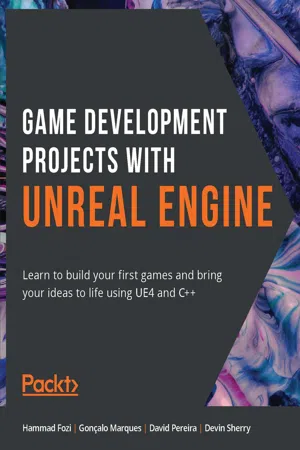
Game Development Projects with Unreal Engine
Learn to build your first games and bring your ideas to life using UE4 and C++
Hammad Fozi, Gonçalo Marques, David Pereira, Devin Sherry
- 822 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Game Development Projects with Unreal Engine
Learn to build your first games and bring your ideas to life using UE4 and C++
Hammad Fozi, Gonçalo Marques, David Pereira, Devin Sherry
About This Book
Learn the tools and techniques of game design using a project-based approach with Unreal Engine 4 and C++
Key Features
- Kickstart your career or dive into a new hobby by exploring game design with UE4 and C++
- Learn the techniques needed to prototype and develop your own ideas
- Reinforce your skills with project-based learning by building a series of games from scratch
Book Description
Game development can be both a creatively fulfilling hobby and a full-time career path. It's also an exciting way to improve your C++ skills and apply them in engaging and challenging projects.
Game Development Projects with Unreal Engine starts with the basic skills you'll need to get started as a game developer. The fundamentals of game design will be explained clearly and demonstrated practically with realistic exercises. You'll then apply what you've learned with challenging activities.
The book starts with an introduction to the Unreal Editor and key concepts such as actors, blueprints, animations, inheritance, and player input. You'll then move on to the first of three projects: building a dodgeball game. In this project, you'll explore line traces, collisions, projectiles, user interface, and sound effects, combining these concepts to showcase your new skills.
You'll then move on to the second project; a side-scroller game, where you'll implement concepts including animation blending, enemy AI, spawning objects, and collectibles. The final project is an FPS game, where you will cover the key concepts behind creating a multiplayer environment.
By the end of this Unreal Engine 4 game development book, you'll have the confidence and knowledge to get started on your own creative UE4 projects and bring your ideas to life.
What you will learn
- Create a fully-functional third-person character and enemies
- Build navigation with keyboard, mouse, gamepad, and touch controls
- Program logic and game mechanics with collision and particle effects
- Explore AI for games with Blackboards and Behavior Trees
- Build character animations with Animation Blueprints and Montages
- Test your game for mobile devices using mobile preview
- Add polish to your game with visual and sound effects
- Master the fundamentals of game UI design using a heads-up display
Who this book is for
This book is suitable for anyone who wants to get started using UE4 for game development. It will also be useful for anyone who has used Unreal Engine before and wants to consolidate, improve and apply their skills. To grasp the concepts explained in this book better, you must have prior knowledge of the basics of C++ and understand variables, functions, classes, polymorphism, and pointers. For full compatibility with the IDE used in this book, a Windows system is recommended.
Frequently asked questions
Information
1. Unreal Engine Introduction
Introduction
Exercise 1.01: Creating an Unreal Engine 4 Project
- After installing Unreal Engine version 4.24, launch the editor by clicking the Launch button of the version icon.
- After you've done so, you'll be greeted with the engine's projects window, which will show you the existing projects that you can open and work on and also give you the option to create a new project. Because we have no projects yet, the Recent Projects section will be empty. To create a new project, you'll first have to choose Project Category, which in our case will be Games.
- After you've selected that option, click the Next button. After that, you'll see the project templates window. This window will show all the available project templates in the Unreal Engine. When creating a new project, instead of having that project start off empty, you have the option to add some assets and code out of the box, which you can then modify to your liking. There are several project templates available for different types of games, but we'll want to go with the Third Person project template in this case.
- Select that template and click the Next button, which should take you to the Project Settings window.In this window, you'll be able to choose a few options related to your project:
- Blueprint or C++: Choose whether you want to be able to add C++ classes. The default option may be Blueprint, but in our case, we'll want to select the C++ option.
- Quality: Choose whether you want your project to have high-quality graphics or high performance. You can set this option to Maximum Quality.
- Raytracing: Choose whether you want Raytracing enabled or disabled. Raytracing is a novel graphics rendering technique which allows you to render objects by simulating the path of light (using light rays) over a digital environment. Although this technique is rather costly in terms of performance, it also provides much more realistic graphics, especially when it comes to lighting. You can set it to disabled.
- Target Platforms: Choose the main platforms you'll want this project to run on. Set this option to Desktop/Console.
- Starter Content: Choose whether you want this project to come with an additional set of basic assets. Set this option to With Starter Content.
- Location and Name: At the bottom of the window, you'll be able to choose the location where your project will be stored on your computer and its name.
- After you've made sure that all the options are set to their intended values, click the Create Project button. This will cause your project to be created according to the parameters you set and may take a few minutes until it's ready.