
Data Structure and Algorithms Using C++
A Practical Implementation
Sachi Nandan Mohanty, Pabitra Kumar Tripathy
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Data Structure and Algorithms Using C++
A Practical Implementation
Sachi Nandan Mohanty, Pabitra Kumar Tripathy
About This Book
Everyone knows that programming plays a vital role as a solution to automate and execute a task in a proper manner. Irrespective of mathematical problems, the skills of programming are necessary to solve any type of problems that may be correlated to solve real life problems efficiently and effectively. This book is intended to flow from the basic concepts of C++ to technicalities of the programming language, its approach and debugging. The chapters of the book flow with the formulation of the problem, it's designing, finding the step-by-step solution procedure along with its compilation, debugging and execution with the output. Keeping in mind the learner's sentiments and requirements, the exemplary programs are narrated with a simple approach so that it can lead to creation of good programs that not only executes properly to give the output, but also enables the learners to incorporate programming skills in them. The style of writing a program using a programming language is also emphasized by introducing the inclusion of comments wherever necessary to encourage writing more readable and well commented programs. As practice makes perfect, each chapter is also enriched with practice exercise questions so as to build the confidence of writing the programs for learners. The book is a complete and all-inclusive handbook of C++ that covers all that a learner as a beginner would expect, as well as complete enough to go ahead with advanced programming. This book will provide a fundamental idea about the concepts of data structures and associated algorithms. By going through the book, the reader will be able to understand about the different types of algorithms and at which situation and what type of algorithms will be applicable.
Frequently asked questions
Information
1
Introduction to Data Structure
1.1 Definition and Use of Data Structure
- Organization of data
- Accessing methods
- Degree of associativity
- Processing alternatives for information
- The data structures must be rich enough in structure to reflect the relationship existing between the data, and
- The structure should be simple so that we can process data effectively whenever required.
1.2 Types of Data Structure
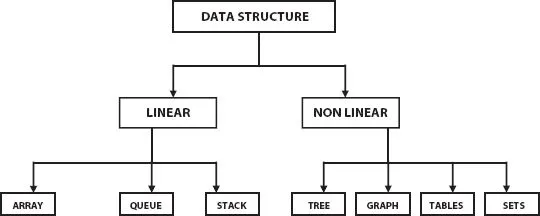
- Traversing
- Insertion
- Deletion
- Merging
- Sorting
- Searching
1.3 Algorithm
- Finding a method for solving a problem. Every step of an algorithm should be defined in a precise and in a clear manner. Pseudo code is also used to describe an algorithm.
- The next step is to validate the algorithm. This step includes all the steps in our algorithm and should be done manually by giving the required input, perform the required steps including in our algorithm and should get the required amount of output in a finite amount of time.
- Finally implement the algorithm in terms of programming language.
- ❖ Floor and Ceiling Functions
- Floor function returns the greatest integer that does not exceed the number.
- Ceiling function returns the least integer that is not less than the number.
- ❖ Remainder Function To find the remainder “mod” function is being used as
- ❖ To find the Integer and Absolute value of a number INT(5.34) = 5 This statement returns the integer part of the numberINT(- 6.45) = 6 This statement returns the absolute as well as the integer portion of the number
- ❖ Summation Symbol To add a series of number as a1+ a2 + a3 +............+ an the symbol Σ is used
- ❖ Factorial of a Number The product of the positive integers from 1 to n is known as the factorial of n and it is denoted as n!.
While writing the algorithm the comments are provided with in [ ].The assignment should use the symbol “: =” instead of “=”For Input use Read : variable nameFor output use write : message/variable name
If condition, then: Statements [end of if structure]
If condition, then: Statements Else : Statements [end of if structure]
If condition1, then: Statements Else If condition2, then: Statements Else If condition3, then: Statements ………………………………………… ………………………………………… ………………………………………… Else If conditionN, then: Statements Else: Statements [end of if structure]
Repeat for var = start_value to end_value by step_value ...