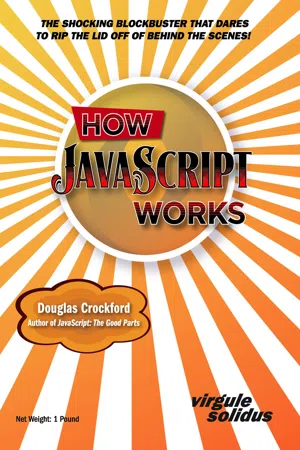
How JavaScript Works
Douglas Crockford
- English
- ePUB (mobile friendly)
- Available on iOS & Android
How JavaScript Works
Douglas Crockford
About This Book
A light-hearted romp thru the world’s most misunderstood programming language.
Douglas Crockford starts by looking at the fundamentals: names, numbers, booleans, characters, and bottom values. JavaScript’s number type is shown to be faulty and limiting, but then Crockford shows how to repair those problems. He then moves on to data structures and functions, exploring the underlying mechanisms and then uses higher order functions to achieve class-free object oriented programming.
The book also looks at eventual programming, testing, and purity, all the while looking at the requirements of The Next Language. Most of our languages are deeply rooted in the paradigm that produced FORTRAN. Crockford attacks those roots, liberating us to consider the next paradigm.
He also presents a strawman language and develops a complete transpiler to implement it. The book is deep, dense, full of code, and has moments when it is intentionally funny.
Frequently asked questions
Information


Front Matter
Did I hear you right, did I hear you sayinâ that youâre gonna make a copy of a game without payinâ? Come on, guys, I thought you knew better. Donât copy that floppy.
Douglas Crockford
ISBN-13 978-1-94-981501-6 Hardcover.
ISBN-13 978-1-94-981502-3 EPUB.
[email protected]
. 005.2762
Chapter List
Raindrops on roses and whiskers on kittens.
[ {"number": 0, "chapter": "Read Me First!"}, {"number": 1, "chapter": "How Names Work"}, {"number": 2, "chapter": "How Numbers Work"}, {"number": 3, "chapter": "How Big Integers Work"}, {"number": 4, "chapter": "How Big Floating Point Works"}, {"number": 5, "chapter": "How Big Rationals Work"}, {"number": 6, "chapter": "How Booleans Work"}, {"number": 7, "chapter": "How Arrays Work"}, {"number": 8, "chapter": "How Objects Work"}, {"number": 9, "chapter": "How Strings Work"}, {"number": 10, "chapter": "How Bottom Values Work"}, {"number": 11, "chapter": "How Statements Work"}, {"number": 12, "chapter": "How Functions Work"}, {"number": 13, "chapter": "How Generators Work"}, {"number": 14, "chapter": "How Exceptions Work"}, {"number": 15, "chapter": "How Programs Work"}, {"number": 16, "chapter": "How this Works"}, {"number": 17, "chapter": "How Class Free Works"}, {"number": 18, "chapter": "How Tail Calls Work"}, {"number": 19, "chapter": "How Purity Works"}, {"number": 20, "chapter": "How Eventual Programming Works"}, {"number": 21, "chapter": "How Date Works"}, {"number": 22, "chapter": "How JSON Works"}, {"number": 23, "chapter": "How Testing Works"}, {"number": 24, "chapter": "How Optimizing Works"}, {"number": 25, "chapter": "How Transpiling Works"}, {"number": 26, "chapter": "How Tokenizing Works"}, {"number": 27, "chapter": "How Parsing Works"}, {"number": 28, "chapter": "How Code Generation Works"}, {"number": 29, "chapter": "How Runtimes Work"}, {"number": 30, "chapter": "How Wat! Works"}, {"number": 31, "chapter": "How This Book Works"} ]
Chapter 0 Read Me First! âââââ
Few images invoke the mysteries and ultimate certainties of a sequence of random events as well as that of the proverbial monkey at a typewriter.
if
statement. If you need assistance with those sorts of details, ask JSLint. jslint.com
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference
If a feature is sometimes useful and sometimes dangerous and if there is a better option then always use the better option.