
eBook - ePub
What Every Engineer Should Know about Microcomputer Software
Keith A. Wehmeyer
This is a test
Share book
- 184 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
What Every Engineer Should Know about Microcomputer Software
Keith A. Wehmeyer
Book details
Book preview
Table of contents
Citations
About This Book
This book covers the entire scope of computer programming and Structured Program Design, from problem identification to maintaining existing programs. It is intended for two audiences: beginning programmers and experienced programmers seeking ways to improve the quality of their software.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is What Every Engineer Should Know about Microcomputer Software an online PDF/ePUB?
Yes, you can access What Every Engineer Should Know about Microcomputer Software by Keith A. Wehmeyer in PDF and/or ePUB format, as well as other popular books in Computer Science & Computer Engineering. We have over one million books available in our catalogue for you to explore.
Information
1
AN INTRODUCTION TO MICROCOMPUTER PROGRAM DESIGN
This chapter presents a complete overview of microcomputer program design, with key topics presented in more detail in subsequent chapters. In addition, a structured technique used in writing programs is presented as it pertains to structured program design. This technique will be discussed throughout the following chapters, as it is the basis for good program design. Finally, the titles and responsibilities of several important people in any programming organization are discussed. We begin now with a discussion of what microcomputer program design is and why it should be used.
STRUCTURED PROGRAM DESIGN
Structured program design (SPD) is a systematic procedure used to create, test, and verify computer software. As in any other design procedure, the programmer works through a sequence of pre-planned steps until the project is completed. This technique offers many advantages to those who use it fully, some of which are as follows:
- Efficiency. The programmer who proceeds under any set of defined guidelines should produce code quicker and with fewer errors. This reduces costs and can prevent many of the difficulties that will be discussed later in this book. As an example, Daly (1) conducted a study that compared software (any computer program) and hardware (the physical parts of a computer) projects of nearly equal complexity. His results showed that the software project took twice as long and cost four times as much to design and maintain as the hardware project. He attributed a large part of this increased time and cost to the fact that the hardware engineers used a more systematic approach toward design.
- Maintenance. It is widely accepted that about 75 - 80% of the programmerâs time is spent maintaining existing corporate software. Software maintenance differs from hardware maintenance in that software is ârepairedâ in order to correct errors or add functions, instead of fixing a system that no longer functions correctly. By using good design techniques, the programmer can free himself to spend more time on new design challenges. In addition, a program written using structured program techniques will be much easier to understand and modify.
- Cost Reduction. Hardware costs drop a factor of ten every decade, so an increasing amount of a projectâs total cost will depend on software generation. While the programmer may not be able to boost productivity at the same rate, any improvement will have an increasingly significant effect in controlling cost.
Unfortunately, many programmers do not use guidelines, and few are learning to use them. In a study by McClure (2), the results pointed to the fact that most programmers had not changed their approach to programming in the last five years and had no plans to do so in the next five. Thus, a great deal of improvement is possible by following only the simplest of guidelines.
PARALLELS WITH ENGINEERING DESIGN
Virtually every engineer is familiar with the engineering method of design. This basic approach stems from the scientific method, which is comprised of the following steps:
- Observe a phenomenon.
- Postulate a theory to explain the occurence of the phenomenon.
- Construct a test to prove the theory.
- Draw conclusions as to the validity of the theory based on the test results.
The engineering method parallels this with the following steps:
- Recognize and specify a need.
- Specify a product to fill the need.
- Design the product according to the above specification.
- Verify that the product design meets the specifications and fills the need.
Note that all four steps in the engineering method could be used to construct a program. Ideally, a specification should first be written based on a set of requirements. Next, a design process should be used to create and debug a program. Finally, the program should be checked against the specification for accuracy and completeness.
Other steps should be included in the software design process as well. Since most programs undergo frequent revisions and modifications, a convenient way of recording and documenting changes should be included. In addition, user documentation should be prepared, such as instruction or operation manuals. This documentation is very important, since it may be the only link between the programâs authors and users.
THE EIGHT STEPS OF STRUCTURED PROGRAM DESIGN
As previously stated, many of the steps used in other forms of engineering design are found in program design. Myers (3) summarizes the work of software generation into the following eight steps:
- Requirements Analysis and Definition. This is the point where the user and the authors begin the design process by deciding what they wish to do with a given configuration of hardware. Notice that the function of the program is to control the hardware in an agreed-upon fashion.
- Specification. At this point the desires of all parties are put into written form and are concretely defined. Time and cost limits are to be established and detailed as well. Since the specification will be referred to throughout the rest of the design process, the creation of a clear, well - defined specification is essential. This is usually the last point where a userâs input is considered until the program is operational.
- Design. Once the program specification is done, the programmer then begins to determine what resources will be required. Following this, construction of a project workbook begins. This workbook should contain the specification and all other materials used in the project. Next, the program logic is contructed using one of several design techniques into a flow chart of operation. This flow chart can be one that uses actual code, English phrases, or symbols to denote what the program will do and when.
- Programming. Once the programâs logic has been charted, it is up to the programmer to convert the flow chart into actual program code. Included in this conversion should be documentation showing the âhow and whyâ of program operation. In essence, the flow chart statements are placed next to the code that performs the corresponding functions. Following this, a structured âwalk-throughâ review of the program is suggested as an error-trapping mechanism before the program is entered into a machine. The scope and function of the structured walk-through is discussed later in this book. Finally, the testing and debugging process continues inside the machine until the program functions as its author believes it should. It is in this phase that most proponents of structured program design techniques feel the greatest amounts of time, money, and energy are saved.
- Verification and Testing. Once the program is operating in the machine, it must be checked against the specification for accuracy and correctness. Programs not meeting the specification will repeat the design process once again from the beginning and will not pass this step until the program fulfills the specified need. Once this is so, the program must then be tested thoroughly for reliability under conditions that any user is expected to have. Finally, the program must be tested under adverse conditions to make sure the program protects both itself and its data from errors and erroneous input by the user. Such âbullet-proofâ testing cannot be exhaustive in large systems, such as a multi-user operating system. In fact, it may not be possible to simulate all possible errors or modes of operation within a reasonable amount of time. In this case, the programmer must choose the most probable errors.
- Performance Appraisal. At this point the program is considered functionally acceptable and further information must be gathered as to its characteristics. Here the program is evaluated on its quality and efficient use of resources such as outside equipment, time, and memory. The software is also evaluated as to its flexibility, clarity, and its ability to be used in other machines or situations (known as portability and adaptability). Finally, the program is checked to see if changes and modifications can be added easily without major revisions. If an area is found unacceptable, the programmer should take steps to optimize those areas so the software will function properly. Another review should then be done against the specification in these areas, as they should be within the scope of a userâs requirements. This step differs from the preceding ones since the programmer shifts emphasis from design to optimization and compression.
- Operation and Maintenance. As previously stated, a programmer spends close to 75% of his time in the function of maintenance. In this step, the operations and instruction manuals are prepared and minor revisions are made to aid the operator in using the software. Errors that have managed to get through the various stages of testing are corrected here. Since users are not always programmers (they may be businesspeople, secretaries, or engineers), care must be taken to make sure they can learn how to use the program in the shortest possible time period and with the least amount of assistance from outside sources.
- Configuration Management. Finally, a method of documenting changes and informing users of this act is needed. Revisions which are desired by users are processed through change notices, repetition of the above design steps, and documentation of the problem and its solution. This leads to the release of new or modified software. This software must somehow be distinguished from previous versions and should include any new applicable instructions. The new software must also be stored so future access is guaranteed for subsequent changes.
Not all programmers need to use every step described above; some may use more, some less. Some may even differ in how the project is divided. The technique presented above is only a small subset of the many methods available to design programs in a structured fashion. The important thing is that a standard technique should be used that will tell the programmer what needs to be done and when. Such techniques help in the initial design effort and will guarantee less confusion when changes are to be made at a later date.
THE CONCEPT OF MODULARITY
Computer programs often become so large that a programmer could not keep track of all the functions at one time. When this situation occurs, the programmer is forced to break the functions up into small groups and work on each group separately. This âdivide and conquerâ technique is called modular decomposition.
Modular decomposition has the advantage of the programmer being able to concentrate his efforts on one individual problem without worrying about others. In addition, several programmers can each tackle one problem simultaneously and then combine their efforts into a finished program. To use this technique, the programmer simply divides the program into groups (commonly called modules), and assigns someone to program and test each module. When complete, the modules are chained together into the finished program.
Modules are usually grouped by a common function or process. Examples of this would be a module which handles all I/O (input/output) using the keyboard and display screen, a module which adds two numbers to it and returns the result, and a module that diagnoses errors and informs the users of any problems. Note from Figure 1.1 that modules are characterized by a single entry point where data is supplied, a process which acts upon the data given to yield a result, and a single exit point which returns the result to the calling program. In addition, these modules can then be broken down further into smaller modules if necessary. An example of this is a program which utilizes one module to handle math functions and is comprised of smaller modules which divide, multiply, subtract, etc. These modules can in turn be broken down into smaller modules which will eventually lead to a module small enough to be transformed into code easily.
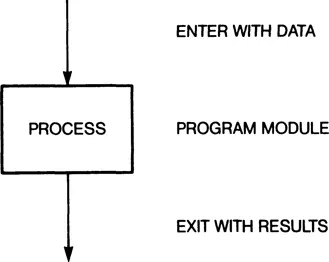
FIGURE 1.1
REASONS FOR MODULAR DECOMPOSITION
As stated before, modular programming allows the use of multiple programmers who can each focus their attention on one specific problem and solve it. When finished, the modules can be grouped together to form the finished program. However, other advantages can be obtained by programming this way.
Since all modules are independent, they can be re-used in other programs that require the same function. Thus, a module need only be written once and then copied for use in other programs. By keeping a catalog of all modules, the programmer can re-use his own and otherâs work instead of re-creating identical software. This will reduce the time and expense of generating future programs.
Since modules are written separately due to their independence, they can be tested separately for the same reason. Eac...