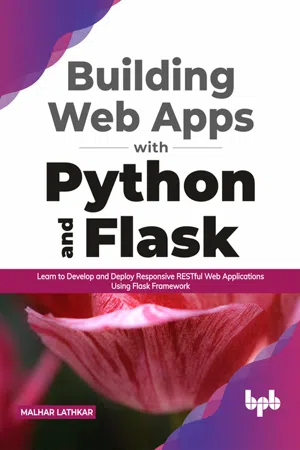
Building Web Apps with Python and Flask
Learn to Develop and Deploy Responsive RESTful Web Applications Using Flask Framework
Malhar Lathkar
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Building Web Apps with Python and Flask
Learn to Develop and Deploy Responsive RESTful Web Applications Using Flask Framework
Malhar Lathkar
About This Book
A practical guide for the rapid web application development with Flask
Key Features
- Expert-led coverage of core capabilities of Flask, key extensions and its implementation.
- Explore the Werkzeug toolkit and Jinja Template engine and see how Flask interacts with JavaScript and CSS.
- Detailed modules on building and deploying RESTful applications using Flask.
Description
This book teaches the reader the complete workflow of developing web applications using Python and its most outperforming microframework, Flask.The book begins with getting you up to speed in developing a strong understanding of the web application development process and how Python is used in developing the applications. You will learn how to write your own first Flask-based web application in Python. You will learn about web gateway interfaces, including CGI and WSGI along with various tools like the Jinja 2 engine, Werkzeug toolkit, and Click toolkit. You will learn and practice the core features of Flask such as URL routing, rendering, handling static assets of a web application, how to handle cookies and sessions, and other HTTP objects. Once you have developed a strong knowledge of Flask, you will now dive deeper into advanced topics that includes Flask extensions for working with relational and NOSQL databases, Flask_WTF, and Flask-Bootstrap. You will explore design patterns, various blueprints on how to build modular and scalable applications, and finally how to deploy the RESTful APIs successfully on your own.
What you will learn
- Get to know everything about the core capabilities of Flask.
- Understand the basic building blocks of Flask.
- Get familiar with advanced features of Flask, including blueprints, Flask extensions, and database connectivity.
- Get ready to design your own Flask-based web applications and RESTful APIs.
- Learn to build modular and scalable applications and how to deploy them successfully.
Who this book is for
This book is ideal for Python enthusiasts, open source contributors, and web app developers who intend to add Python web technologies in their skillsets and startup companies. The understanding of the core Python language with intermediate level expertise is required and experience of working with SQL, HTML, CSS, and JavaScript is an added advantage.
Table of Contents
1. Python for CGI
2. WSGI
3. Flask Fundamentals
4. URL Routing
5. Rendering Templates
6. Static Files
7. HTTP Objects
8. Using Databases
9. More Flask Extensions
10. Blueprints and Contexts
11. Web API with Flask
12. Deploying Flask Applications
13. Appendix
About the Author
Malhar Lathkar is an independent software professional, corporate trainer, freelance technical writer, and Subject Matter Expert with an experience of more than three decades. He has trained hundreds of students/professionals in Python, Data Science, Java and Android, PHP and web development, etc.He also has the experience of delivering talks and conducting workshops on various IT topics.He writes regularly in a local newspaper on sports and technology-related current topics. LinkedIn Profile: https://www.linkedin.com/in/malharlathkar
Frequently asked questions
Information
CHAPTER 1
Python for CGI
Introduction
Structure
- Advent of WWW
- What is CGI?
- Configuration of Apache server
- The basics of HTTP protocol
- Request methods
- The cgi module
- Cookies
- Alternatives to CGI
Objectives
- Understand the basics of CGI
- Use Python as CGI script
Advent of WWW
wwwroot
. The server maps client's request to the corresponding web page in the document root and renders its HTML content to the client browser.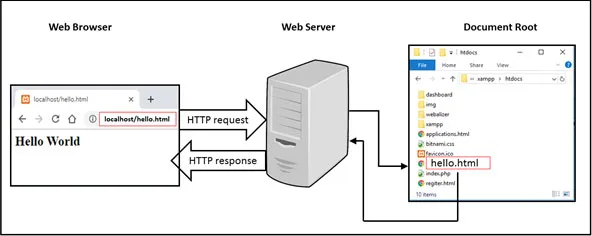
What is CGI?
.py
extension) are used as CGI scripts.cgi-bin
, in which these scripts are stored. On receiving a request, the web server looks for and executes the corresponding script. Obviously, runtime environment of the language in which the said script is written must be available for the server.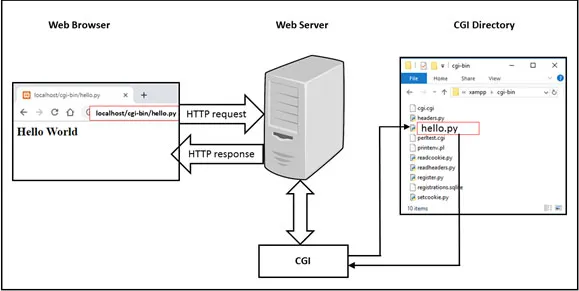
Configuration of Apache Server
C:\XAMPP
folder.htdocs
folder and a cgi-bin
folder (apart from others) will be seen. The htdocs
folder is configured as document root of the server
, and cgi-bin
is the folder to store CGI script.cgi
requests. For that, you need to open Apache's httpd.conf
configuration file (found at C:\xampp\apache\conf\httpd.conf
) using any text editor (for example, Notepad) and look for a string AddHandler
in it.AddHandler cgi-script
directive tells the server which types of files are to be used as cgi
scripts. Ensure that the .py
extension is added in the list of extensions as follows:AddHandler cgi-script .cgi .pl .py
.cgi
, .pl
, and .py
extensions as CGI script. Now you are ready to launch the Apache server. To test the instal...