
Advanced Algorithms and Data Structures
Marcello La Rocca
- 768 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Advanced Algorithms and Data Structures
Marcello La Rocca
About This Book
"An accessible introduction to the fundamental algorithms used to run the world." - Richard Vaughan, Purple Monkey Collective Advanced Algorithms and Data Structures introduces a collection of algorithms for complex programming challenges in data analysis, machine learning, and graph computing. Summary
As a software engineer, you'll encounter countless programming challenges that initially seem confusing, difficult, or even impossible. Don't despair! Many of these "new" problems already have well-established solutions. Advanced Algorithms and Data Structures teaches you powerful approaches to a wide range of tricky coding challenges that you can adapt and apply to your own applications. Providing a balanced blend of classic, advanced, and new algorithms, this practical guide upgrades your programming toolbox with new perspectives and hands-on techniques.Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications. About the technology
Can you improve the speed and efficiency of your applications without investing in new hardware? Well, yes, you can: Innovations in algorithms and data structures have led to huge advances in application performance. Pick up this book to discover a collection of advanced algorithms that will make you a more effective developer. About the book
Advanced Algorithms and Data Structures introduces a collection of algorithms for complex programming challenges in data analysis, machine learning, and graph computing. You'll discover cutting-edge approaches to a variety of tricky scenarios. You'll even learn to design your own data structures for projects that require a custom solution. What's inside
Build on basic data structures you already know
Profile your algorithms to speed up application
Store and query strings efficiently
Distribute clustering algorithms with MapReduce
Solve logistics problems using graphs and optimization algorithms About the reader
For intermediate programmers. About the author
Marcello La Rocca is a research scientist and a full-stack engineer. His focus is on optimization algorithms, genetic algorithms, machine learning, and quantum computing. Table of Contents 1 Introducing data structures
PART 1 IMPROVING OVER BASIC DATA STRUCTURES
2 Improving priority queues: d-way heaps
3 Treaps: Using randomization to balance binary search trees
4 Bloom filters: Reducing the memory for tracking content
5 Disjoint sets: Sub-linear time processing
6 Trie, radix trie: Efficient string search
7 Use case: LRU cache
PART 2 MULTIDEMENSIONAL QUERIES
8 Nearest neighbors search
9 K-d trees: Multidimensional data indexing
10 Similarity Search Trees: Approximate nearest neighbors search for image retrieval
11 Applications of nearest neighbor search
12 Clustering
13 Parallel clustering: MapReduce and canopy clustering
PART 3 PLANAR GRAPHS AND MINIMUM CROSSING NUMBER
14 An introduction to graphs: Finding paths of minimum distance
15 Graph embeddings and planarity: Drawing graphs with minimal edge intersections
16 Gradient descent: Optimization problems (not just) on graphs
17 Simulated annealing: Optimization beyond local minima
18 Genetic algorithms: Biologically inspired, fast-converging optimization
Frequently asked questions
Information
1 Introducing data structures
- Explaining why you should learn about data structures and algorithms
- Understanding the difference between algorithms and data structures
- Abstracting a problem
- Moving from problems to solutions
1.1 Data structures
1.1.1 Defining a data structure
array = [âCâ, âAâ, âRâ]
is stored: an array of characters storing the characters C
, A
, and R
, such that, for instance, array[1]
corresponds to the value âAâ
.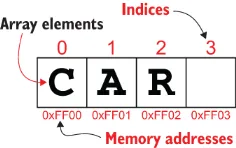
- An abstract data type (ADT) specifies the operations that can be performed on some data and the computational complexity of those operations. No details are provided on how data is stored or how physical memory is used.
- A data structure (DS) is a concrete implementation of the specification provided by an ADT.