
Angular Projects
Build modern web apps by exploring Angular 12 with 10 different projects and cutting-edge technologies, 2nd Edition
Aristeidis Bampakos
- 344 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Angular Projects
Build modern web apps by exploring Angular 12 with 10 different projects and cutting-edge technologies, 2nd Edition
Aristeidis Bampakos
About This Book
PUBLISHER'S NOTE: An updated 2023 edition, compatible with Angular 16, is now available.
Key Features
- Explore Angular's capabilities for building applications across different platforms
- Combine popular web technologies with Angular such as monorepo, Jamstack, and PWA
- Build your own libraries and schematics using Angular CDK and Angular CLI
Book Description
Packed with practical advice and detailed recipes, this updated second edition of Angular Projects will teach you everything you need to know to build efficient and optimized web applications using Angular. Among the things you'll learn in this book are the essential features of the framework, which you'll master by creating ten different real-world web applications. Each application will demonstrate how to integrate Angular with a different library and tool. As you advance, you'll familiarize yourself with implementing popular technologies, such as Angular Router, Scully, Electron, Angular service worker, Nx monorepo tools, NgRx, and more while building an issue tracking system. You'll also work on a PWA weather application, a mobile photo geotagging application, a component UI library, and many other exciting projects.In the later chapters, you'll get to grips with customizing Angular CLI commands using schematics.By the end of this book, you will have the skills you need to be able to build Angular apps using a variety of different technologies according to your or your client's needs.
What you will learn
- Set up Angular applications using Angular CLI and Nx Console
- Create a personal blog with Jamstack and SPA techniques
- Build desktop applications with Angular and Electron
- Enhance user experience (UX) in offline mode with PWA techniques
- Make web pages SEO-friendly with server-side rendering
- Create a monorepo application using Nx tools and NgRx for state management
- Focus on mobile application development using Ionic
- Develop custom schematics by extending Angular CLI
Who this book is for
This book is for developers with beginner-level Angular experience who want to become proficient in using essential tools and dealing with the various use cases they may encounter in Angular. Beginner-level knowledge of web application development and basic experience working with ES6 or TypeScript is essential before you dive in.
]]>
Frequently asked questions
Information
Chapter 1: Creating Your First Web Application in Angular
- Introduction to Angular
- Introduction to the Angular CLI
- Exploring the rich ecosystem of Angular tooling in VSCode
- How to create our first Angular application
- How to use Nx Console for automating Angular CLI commands
Essential background theory and context
- A list of blog posts
- An issue reporting form
- A weather display widget
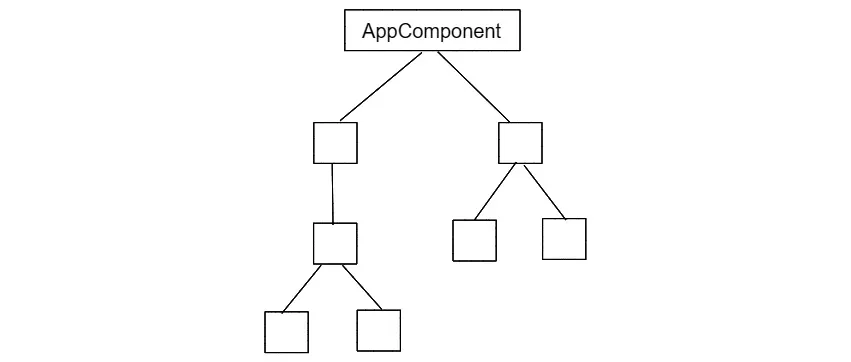
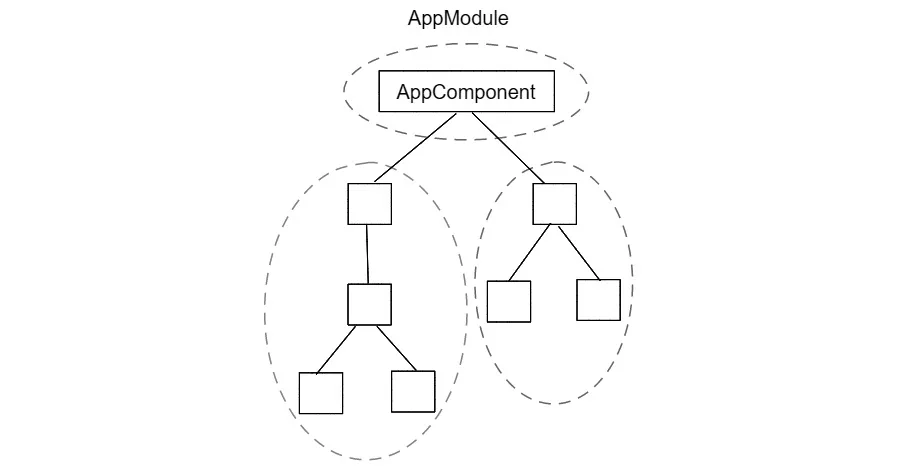
- Access data from a backend API using the HTTP protocol.
- Interact with the local storage of the browser.
- Error logging.
- Data transformations.
Introduction to the Angular CLI
- Node.js: A JavaScript runtime that is built on the v8 engine of Chrome. You can download any Long-Term Support (LTS) version from https://nodejs.org/en.
- npm: A package manager for the Node.js runtime.