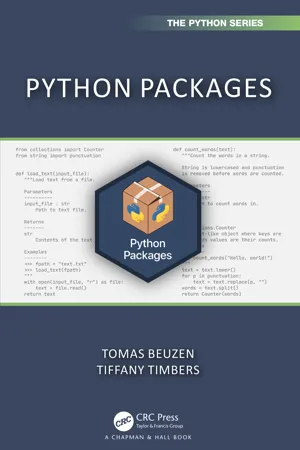
eBook - ePub
Python Packages
Tomas Beuzen, Tiffany Timbers
This is a test
Share book
- 200 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Python Packages
Tomas Beuzen, Tiffany Timbers
Book details
Book preview
Table of contents
Citations
About This Book
Python Packages introduces Python packaging at an introductory and practical level that's suitable for those with no previous packaging experience. Despite this, the text builds up to advanced topics such as automated testing, creating documentation, versioning and updating a package, and implementing continuous integration and deployment. Covering the entire Python packaging life cycle, this essential guide takes readers from package creation all the way to effective maintenance and updating.
Python Packages focuses on the use of current and best-practice packaging tools and services like poetry, cookiecutter, pytest, sphinx, GitHub, and GitHub Actions.
Features:
- The book's source code is available online as a GitHub repository where it is collaborated on, automatically tested, and built in real time as changes are made; demonstrating the use of good reproducible and clear project workflows.
- Covers not just the process of creating a package, but also how to document it, test it, publish it to the Python Package Index (PyPI), and how to properly version and update it.
- All concepts in the book are demonstrated using examples. Readers can follow along, creating their own Python packages using the reproducible code provided in the text.
- Focuses on a modern approach to Python packaging with emphasis on automating and streamlining the packaging process using new and emerging tools such as poetry and GitHub Actions.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Python Packages an online PDF/ePUB?
Yes, you can access Python Packages by Tomas Beuzen, Tiffany Timbers in PDF and/or ePUB format, as well as other popular books in Informatik & Programmierung in Python. We have over one million books available in our catalogue for you to explore.
Information
1Introduction
DOI: 10.1201/9781003189251-1
Python packages are a core element of the Python programming language and are how you write reusable and shareable code in Python. This book assumes that readers are familiar with how to install a package using a package installer like pip or conda, and how to import and use it with the help of the import statement in Python.
For example, the command below uses pip to install numpy(Harris et al., 2020), the core scientific computing package for Python:

Once the package is installed, it can be used in a Python interpreter. For example, to round pi to three decimal places:
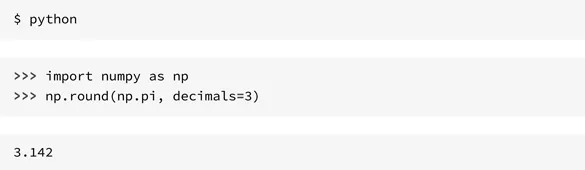
At a minimum, a package bundles together code (such as functions, classes, variables, or scripts) so that it can be easily reused across different projects. However, packages are typically also supported by extra content such as documentation and tests, which become exponentially more important if you wish to share your package with others.
As of January 2022, there are over 350,000 packages available on the Python Package Index (PyPI)1, the official online software repository for Python. Packages are a key reason why Python is such a powerful and widely used programming language. The chances are that someone has already solved a problem that you're working on, and you can benefit from their work by downloading and installing their package. Put simply, packages are how you make it as easy as possible to use, maintain, share, and collaborate on Python code with others, whether they be your friends, work colleagues, the world, or your future self!
Even if you never intend to share your code with others, making packages will ultimately save you time. Packages make it significantly easier for you to reuse and maintain your code within a project and across different projects. After programming for some time, most people will eventually reach a point where they want to reuse code from one project in another. For beginners, in particular, this is something often accomplished by copying-and-pasting existing code into the new project. Despite being inefficient, this practice also makes it difficult to improve and maintain your code across projects. Creating a simple Python package will solve these problems.
Regardless of your motivation, the goal of this book is to show you how to easily develop Python packages. The focus is overwhelmingly practical â we will leverage modern methods and tools to develop and maintain packages efficiently, reproducibly, and with as much automation as possible, so you can focus on writing and sharing code. Along the way, we'll also enlighten some interesting and relevant lower-level details of Python packaging and the Python programming language.
1.1âWhy you should create packages
There are many reasons why you should develop Python packages!
- To effectively share your code with others.
- They save you time. Even if you don't intend to share your package with others, they help you easily reuse and maintain your code across multiple projects.
- They force you to organize and document your code, such that it can be easily understood and used at a later time.
- They isolate dependencies for your code and improve its reproducibility.
- They are a good way to practice writing good code.
- Packages can be used to effectively bundle up reproducible data analysis and programming projects.
- Finally, developing and distributing packages supports the Python ecosystem and other Python users who can benefit from your work.
2System setup
DOI: 10.1201/9781003189251-2
If you intend to follow along with the code presented in this book, we recommend you follow these setup instructions so that you will run into fewer technical issues.
2.1 The command-line interface
A command-line interface (CLI) is a text-based interface used to interact with your computer. We'll be using a CLI for various tasks throughout this book. We'll assume Mac and Linux users are using the âTerminalâ and Windows users are using the âAnaconda Promptâ (which we'll install in the next section) as a CLI.
2.2 Installing software
Section 2.2.1 and Section 2.2.2 describe how to install the software you'll need to develop a Python package and follow along with the text and examples in this book. However, we also support an alternative setup with Docker that has everything you need already installed to get started. The Docker approach is recommended for anyone that runs into issues installing or using any of the software below on their specific operating system, or anyone who would simply prefer to use Docker â if that's you, skip to Section 2.3 for now, and we'll describe the Docker setup later in Section 2.6.
2.2.1 Installing Python
We recommend installing the latest version of Python via the Miniconda distribution by following the instructions in the M...