
Android System Programming
Roger Ye
- 470 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Android System Programming
Roger Ye
About This Book
Build, customize, and debug your own Android systemAbout This Book⢠Master Android system-level programming by integrating, customizing, and extending popular open source projects⢠Use Android emulators to explore the true potential of your hardware⢠Master key debugging techniques to create a hassle-free development environmentWho This Book Is ForThis book is for Android system programmers and developers who want to use Android and create indigenous projects with it. You should know the important points about the operating system and the C/C++ programming language.What You Will Learn⢠Set up the Android development environment and organize source code repositories⢠Get acquainted with the Android system architecture⢠Build the Android emulator from the AOSP source tree⢠Find out how to enable WiFi in the Android emulator⢠Debug the boot up process using a customized Ramdisk⢠Port your Android system to a new platform using VirtualBox⢠Find out what recovery is and see how to enable it in the AOSP build⢠Prepare and test OTA packagesIn DetailAndroid system programming involves both hardware and software knowledge to work on system level programming. The developers need to use various techniques to debug the different components in the target devices. With all the challenges, you usually have a deep learning curve to master relevant knowledge in this area. This book will not only give you the key knowledge you need to understand Android system programming, but will also prepare you as you get hands-on with projects and gain debugging skills that you can use in your future projects.You will start by exploring the basic setup of AOSP, and building and testing an emulator image. In the first project, you will learn how to customize and extend the Android emulator. Then you'll move on to the real challengeābuilding your own Android system on VirtualBox. You'll see how to debug the init process, resolve the bootloader issue, and enable various hardware interfaces. When you have a complete system, you will learn how to patch and upgrade it through recovery. Throughout the book, you will get to know useful tips on how to integrate and reuse existing open source projects such as LineageOS (CyanogenMod), Android-x86, Xposed, and GApps in your own system.Style and approachThis is an easy-to-follow guide full of hands-on examples and system-level programming tips.
Frequently asked questions
Information
Enabling Graphics
- Overview of Android graphics architecture
- Delving into graphics HAL
- Analyzing the Android emulator graphics HAL for comparison
As you will see, the content in this chapter is much longer than the rest. Reading and understanding the content in this chapter may be harder. What I suggest is that you can open a source code editor and load the relevant source code while you read this chapter. This will help you a lot to understand the source code and the points that I want to address in this chapter.
Introduction to the Android graphics architecture
- EGL driver
- OpenGL ES 1.x driver
- OpenGL ES 2.0 driver
- OpenGL ES 3.x driver (optional)
- Vulkan (optional)
- Gralloc HAL implementation
- Hardware Composer HAL implementation
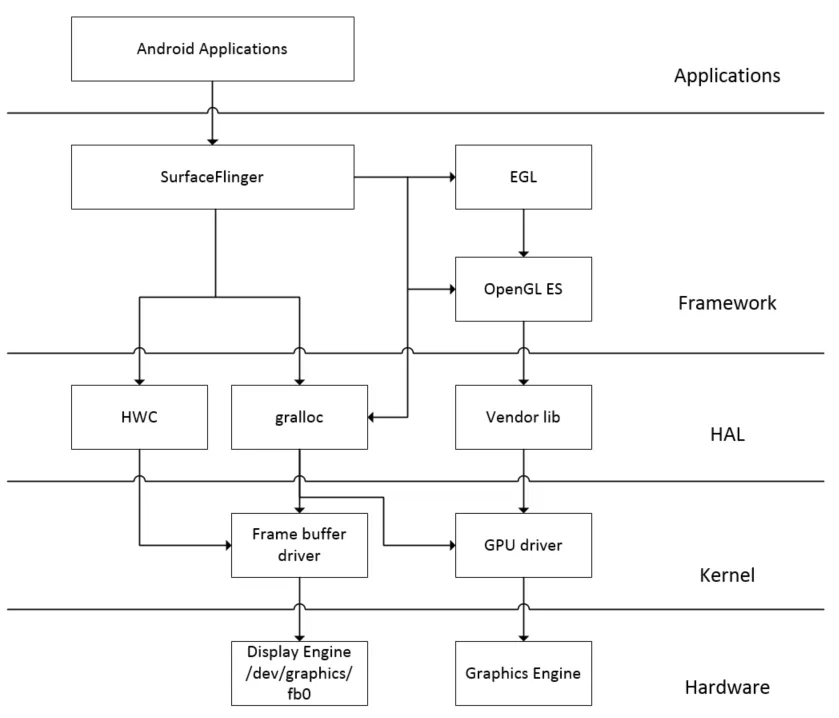
Delving into graphics HAL
Loading the Gralloc module
int hw_get_module(const char *id, const struct hw_module_t **module)
{
return hw_get_module_by_class(id, NULL, module);
}
int hw_get_module_by_class(const char *class_id, const char *inst,
const struct hw_module_t **module)
{
int i = 0;
char prop[PATH_MAX] = {0};
char path[PATH_MAX] = {0};
char name[PATH_MAX] = {0};
char prop_name[PATH_MAX] = {0};
if (inst)
snprintf(name, PATH_MAX, "%s.%s", class_id, inst);
else
strlcpy(name, class_id, PATH_MAX);
snprintf(prop_name, sizeof(prop_name), "ro.hardware.%s", name);
if (property_get(prop_name, prop, NULL) > 0) {
if (hw_module_exists(path, sizeof(path), name, prop) == 0) {
goto found;
}
}
for (i=0 ; i<HAL_VARIANT_KEYS_COUNT; i++) {
if (property_get(variant_keys[i], prop, NULL) == 0) {
continue;
}
if (hw_module_exists(path, sizeof(path), name, prop) == 0) {
goto found;
}
}
/* Nothing found, try the default */
if (hw_module_exists(path, sizeof(path), name, "default") == 0) {
goto found;
}
return -ENOENT;
found:
return load(class_id, path, module);
}
gralloc.<ro.hardware>.so
gralloc.<ro.product.board>.so
gralloc.<ro.board.platform>.so
gralloc.<ro.arch>.so
#define GRALLOC_HARDWARE_MODULE_ID "gralloc"