
Cross-platform Desktop Application Development: Electron, Node, NW.js, and React
Dmitry Sheiko
- 300 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Cross-platform Desktop Application Development: Electron, Node, NW.js, and React
Dmitry Sheiko
About This Book
Build powerful cross-platform desktop applications with web technologies such as Node, NW.JS, Electron, and ReactAbout This Book• Build different cross-platform HTML5 desktop applications right from planning, designing, and deployment to enhancement, testing, and delivery• Forget the pain of cross-platform compatibility and build efficient apps that can be easily deployed on different platforms.• Build simple to advanced HTML5 desktop apps, by integrating them with other popular frameworks and libraries such as Electron, Node.JS, Nw.js, React, Redux, and TypeScript Who This Book Is ForThis book has been written for developers interested in creating desktop applications with HTML5. The first part requires essential web-master skills (HTML, CSS, and JavaScript). The second demands minimal experience with React. And finally for the third it would be helpful to have a basic knowledge of React, Redux, and TypeScript.What You Will Learn• Plan, design, and develop different cross-platform desktop apps• Application architecture with React and local state• Application architecture with React and Redux store• Code design with TypeScript interfaces and specialized types• CSS and component libraries such as Photonkit, Material UI, and React MDL• HTML5 APIs such as desktop notifications, WebSockets, WebRTC, and others• Desktop environment integration APIs of NW.js and Electron• Package and distribute for NW.JS and ElectronIn DetailBuilding and maintaining cross-platform desktop applications with native languages isn't a trivial task. Since it's hard to simulate on a foreign platform, packaging and distribution can be quite platform-specific and testing cross-platform apps is pretty complicated.In such scenarios, web technologies such as HTML5 and JavaScript can be your lifesaver. HTML5 desktop applications can be distributed across different platforms (Window, MacOS, and Linux) without any modifications to the code.The book starts with a walk-through on building a simple file explorer from scratch powered by NW.JS. So you will practice the most exciting features of bleeding edge CSS and JavaScript. In addition you will learn to use the desktop environment integration API, source code protection, packaging, and auto-updating with NW.JS.As the second application you will build a chat-system example implemented with Electron and React. While developing the chat app, you will get Photonkit. Next, you will create a screen capturer with NW.JS, React, and Redux.Finally, you will examine an RSS-reader built with TypeScript, React, Redux, and Electron. Generic UI components will be reused from the React MDL library. By the end of the book, you will have built four desktop apps. You will have covered everything from planning, designing, and development to the enhancement, testing, and delivery of these apps.Style and approachFilled with real world examples, this book teaches you to build cross-platform desktop apps right from scratch using a step-by-step approach.
Frequently asked questions
Information
Creating a File Explorer with NW.js-Planning, Designing, and Development
The application blueprint
- As a user, I can see the content of the current directory
- As a user, I can navigate through the filesystem
- As a user, I can open a file in the default associated program
- As a user, I can delete a file
- As a user, I can copy a file in the clipboard and paste it later in a new location
- As a user, I can open the folder containing the file with the system file manager
- As a user, I can close the application window
- As a user, I can minimize the application window
- As a user, I can maximize and restore the application window
- As a user, I can change the application language

Setting up an NW.js project
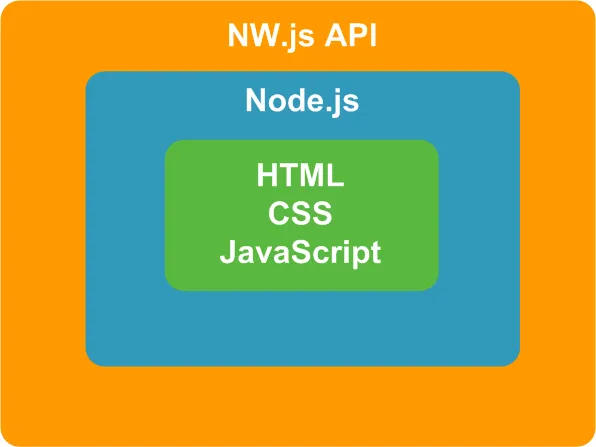
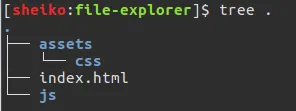
./index.html
<!DOCTYPE html>
<html>
<body>
<h1>File Explorer</h1>
</body>
</html>
Node Package Manager
sudo apt-get install npm
sudo npm install npm@latest -g
sudo npm install nw --global
sudo npm i nw -g