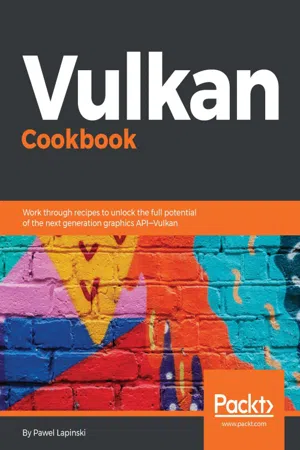
Vulkan Cookbook
Pawel Lapinski
- 700 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Vulkan Cookbook
Pawel Lapinski
About This Book
Work through recipes to unlock the full potential of the next generation graphics APIâVulkanAbout This Book⢠This book explores a wide range of modern graphics programming techniques and GPU compute methods to make the best use of the Vulkan API⢠Learn techniques that can be applied to a wide range of platforms desktop, smartphones, and embedded devices⢠Get an idea on the graphics engine with multi-platform support and learn exciting imaging processing and post-processing techniquesWho This Book Is ForThis book is ideal for developers who know C/C++ languages, have some basic familiarity with graphics programming, and now want to take advantage of the new Vulkan API in the process of building next generation computer graphics. Some basic familiarity of Vulkan would be useful to follow the recipes. OpenGL developers who want to take advantage of the Vulkan API will also find this book useful.What You Will Learn⢠Work with Swapchain to present images on screen⢠Create, submit, and synchronize operations processed by the hardware⢠Create buffers and images, manage their memory, and upload data to them from CPU⢠Explore descriptor sets and set up an interface between application and shaders⢠Organize drawing operations into a set of render passes and subpasses⢠Prepare graphics pipelines to draw 3D scenes and compute pipelines to perform mathematical calculationsâ˘Implement geometry projection and tessellation, texturing, lighting, and post-processing techniquesâ˘Write shaders in GLSL and convert them into SPIR-V assembliesâ˘Find out about and implement a collection of popular, advanced rendering techniques found in games and benchmarksIn DetailVulkan is the next generation graphics API released by the Khronos group. It is expected to be the successor to OpenGL and OpenGL ES, which it shares some similarities with such as its cross-platform capabilities, programmed pipeline stages, or nomenclature. Vulkan is a low-level API that gives developers much more control over the hardware, but also adds new responsibilities such as explicit memory and resources management. With it, though, Vulkan is expected to be much faster.This book is your guide to understanding Vulkan through a series of recipes. We start off by teaching you how to create instances in Vulkan and choose the device on which operations will be performed. You will then explore more complex topics such as command buffers, resources and memory management, pipelines, GLSL shaders, render passes, and more. Gradually, the book moves on to teach you advanced rendering techniques, how to draw 3D scenes, and how to improve the performance of your applications.By the end of the book, you will be familiar with the latest advanced techniques implemented with the Vulkan API, which can be used on a wide range of platforms.Style and approachThis recipe-based guide will empower you to implement modern graphic programming techniques and help gain a solid understanding of the new Vulkan API.
Frequently asked questions
Information
Graphics and Compute Pipelines
- Creating a shader module
- Specifying pipeline shader stages
- Specifying a pipeline vertex binding description, attribute description, and input state
- Specifying a pipeline input assembly state
- Specifying a pipeline tessellation state
- Specifying a pipeline viewport and scissor test state
- Specifying a pipeline rasterization state
- Specifying a pipeline multisample state
- Specifying a pipeline depth and stencil state
- Specifying a pipeline blend state
- Specifying pipeline dynamic states
- Creating a pipeline layout
- Specifying graphics pipeline creation parameters
- Creating a pipeline cache object
- Retrieving data from a pipeline cache
- Merging multiple pipeline cache objects
- Creating a graphics pipeline
- Creating a compute pipeline
- Binding a pipeline object
- Creating a pipeline layout with a combined image sampler, a buffer, and push constant ranges
- Creating a graphics pipeline with vertex and fragment shaders, depth test enabled, and with dynamic viewport and scissor tests
- Creating multiple graphics pipelines on multiple threads
- Destroying a pipeline
- Destroying a pipeline cache
- Destroying a pipeline layout
- Destroying a shader module
Introduction
Creating a shader module
How to do it...
- Take the handle of a logical device stored in a variable of type VkDevice named logical_device.
- Load a binary SPIR-V assembly of a selected shader and store it in a variable of type std::vector<unsigned char> named source_code.
- Create a variable of type VkShaderModuleCreateInfo named shader_module_create_info. Use the following values to initialize its members:
- VK_STRUCTURE_TYPE_SHADER_MODULE_CREATE_INFO value for sType.
- nullptr value for pNext
- 0 value for flags
- The number of elements in the source_code vector (size in bytes) for codeSize
- A pointer to the first element of the source_code variable for pCode
- Create a variable of type VkShaderModule named shader_module in which the handle of a created shader module will be stored.
- Make the vkCreateShaderModule( logical_device, &shader_module_create_info, nullptr, &shader_module ) function call for which provide the logical_device variable, a pointer to the shader_module_create_info, a nullptr value, and a pointer to the shader_module variable.
- Make sure the vkCreateShaderModule() function call returned a VK_SUCCESS value which indicates that the shader module was properly created.
How it works...
VkShaderModuleCreateInfo shader_module_create_info = { VK_STRUCTURE_TYPE_SHADER_MODULE_CREATE_INFO, nullptr, 0, source_code.size(), reinterpret_cast<uint32_t const *>(source_code.data()) };
VkResult result = vkCreateShaderModule( logical_device, &shader_module_create_info, nullptr, &shader_module ); if( VK_SUCCESS != result ) { std::cout << "Could not create a shader module." << std::endl; return false; } return true;
See also
- Specifying pipeline shader stages
- Creating a graphics pipeline
- Creating a compute pipeline
- Destroying a shader module
Specifying pipeline shader stages
Getting ready
structShaderStageParameters { VkShaderStageFlagBits ShaderStage; VkShaderModule ShaderModule; char const * EntryPointName; VkSpecializationInfo const * SpecializationInfo; };
How to do it...
- Create a shader module or modules containing source code for each shader stage that will be active in a given pipeline (refer to the Creating a shader module recipe).
- Create a std::vector variable named shader_stage_create_infos with elements of type VkPipelineShaderStageCreateInfo.
- For each shader stage that should be enabled in a given pipeline, add an element to the shader_stage_create_infos vector and use the following values to initialize its members:
- VK_STRUCTURE_TYPE_PIPELINE_SHADER_STAGE_CREATE_INFO value for sType
- nullptr value for pNext
- 0 value for flags
- The selected shader stage for stage
- The shader module with a source code of a given shader stage for module
- The name of the fu...