
WordPress Plugin Development Cookbook - Second Edition
Yannick Lefebvre
- 386 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
WordPress Plugin Development Cookbook - Second Edition
Yannick Lefebvre
About This Book
Learn to create plugins for WordPress 4.x to deliver custom projects or share with the community through detailed step-by-step recipes and code examplesAbout This Book⢠Learn how to change and extend WordPress to perform virtually any task⢠Explore the plugin API through approachable examples and detailed explanations⢠Mold WordPress to your project's needs or transform it to benefit the entire communityWho This Book Is ForIf you are a WordPress user, developer, or a site integrator with basic knowledge of PHP and an interest to create new plugins to address your personal needs, client needs, or share with the community, then this book is for you.What You Will Learn⢠Discover how to register user callbacks with WordPress, forming the basis of plugin creation⢠Explore the creation of administration pages and adding new content management sections through custom post types and custom database tables⢠Improve your plugins by customizing the post and page editors, categories and user profiles, and creating visitor-facing forms⢠Make your pages dynamic using Javascript, AJAX and adding new widgets to the platform⢠Learn how to add support for plugin translation and distribute your work to the WordPress communityIn DetailWordPress is a popular, powerful, and open Content Management System. Learning how to extend its capabilities allows you to unleash its full potential, whether you're an administrator trying to find the right extension, a developer with a great idea to enhance the platform for the community, or a website developer working to fulfill a client's needs. This book shows readers how to navigate WordPress' vast set of API functions to create high-quality plugins with easy-to-configure administration interfaces.With new recipes and materials updated for the latest versions of WordPress 4.x, this second edition teaches you how to create plugins of varying complexity ranging from a few lines of code to complex extensions that provide intricate new capabilities.You'll start by using the basic mechanisms provided in WordPress to create plugins and execute custom user code. You will then see how to design administration panels, enhance the post editor with custom fields, store custom data, and modify site behavior based on the value of custom fields. You'll safely incorporate dynamic elements on web pages using scripting languages, and build new widgets that users will be able to add to WordPress sidebars and widget areas.By the end of this book, you will be able to create WordPress plugins to perform any task you can imagine.Style and approachThis cookbook will take you through the creation of your first simple plugin to adding entirely new sections and widgets in the administration interface, so you can learn how to change and extend WordPress to perform virtually any task. Each topic is illustrated through realistic examples showing how to solve common problems, followed by detailed explanations of all concepts used
Frequently asked questions
Information
User Settings and Administration Pages
- Creating default user settings on plugin initialization
- Storing user settings using arrays
- Removing plugin data on deletion
- Creating an administration page menu item in the settings menu
- Creating a multi-level administration menu
- Adding menu items leading to external pages
- Hiding items that users should not access from the default menu
- Rendering the admin page content using HTML
- Processing and storing plugin configuration data
- Displaying a confirmation message when options are saved
- Adding custom help pages
- Rendering the admin page contents using the Settings API
- Accessing user settings from action and filter hooks
- Formatting admin pages using meta boxes
- Splitting admin code from the main plugin file to optimize site performance
- Storing style sheet data in user settings
- Managing multiple sets of user settings from a single admin page
- Creating a network level plugin with admin pages
Introduction
Creating default user settings on plugin initialization
How to do it...
- Navigate to the WordPress plugin directory of your development installation.
- Create a new directory called ch3-individual-options.
- Navigate to this directory and create a new text file called ch3-individual-options.php.
- Open the new file in a code editor and add an appropriate header at the top of the plugin file, naming the plugin Chapter 3 - Individual Options.
- Add the following line of code to register a function that will be executed when the plugin is activated, after its initial installation or following an upgrade:
register_activation_hook( __FILE__, 'ch3io_set_default_options' );
- Add the following code section to provide an implementation for the ch3io_set_default_options function:
function ch3io_set_default_options() {
if ( false === get_option( 'ch3io_ga_account_name' ) ) {
add_option( 'ch3io_ga_account_name', 'UA-0000000-0' );
}
}
- Save and close the plugin file. Execute the activation function that was just added by clicking on the Activate option of this chapter 3 - Individual Options plugin.
- In your web server's MySQL database administration tool, select your WordPress database (wordpressdev if you followed the recipe in Chapter 1, Preparing a Local Development Environment), then select the wpdev_options table. Click on the SQL tab, replace the default query with the following statement, and click on Go:
select * from wpdev_options where option_name = 'ch3io_ga_account_name'
- Your query should return a single row with the default value assigned to the new option:
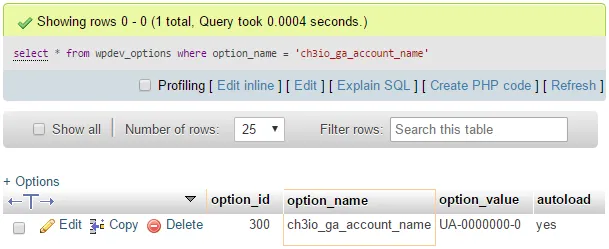