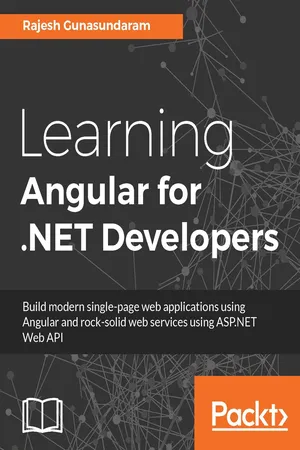
Learning Angular for .NET Developers
Rajesh Gunasundaram
- 248 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Learning Angular for .NET Developers
Rajesh Gunasundaram
About This Book
Build efficient web apps and deliver great results by integrating Angular and the.NET frameworkAbout This Book⢠Become a more productive developer and learn to use frameworks that implement good development practices⢠Achieve advanced autocompletion, navigation, and refactoring in Angular using Typescript⢠Follow a gradual introduction to the concepts with a lot of examples and explore the evolution of a production-ready applicationWho This Book Is ForIf you are a.NET developer who now wants to efficiently build single-page applications using the new features that Angular 4 has to offer, then this book is for you. Familiarity of HTML, CSS, and JavaScript is assumed to get the most from this book.What You Will Learn⢠Create a standalone Angular application to prototype user interfaces⢠Validate complex forms with Angular version 4 and use Bootstrap to style them⢠Build RESTful web services that work well with single-page applications⢠Use Gulp and Bower in Visual Studio to run tasks and manage JavaScript packages⢠Implement automatic validation for web service requests to reduce your boilerplate code⢠Use web services with Angular version 4 to offload and secure your application logic⢠Test your Angular version 4 and web service code to improve the quality of your software deliverablesIn DetailAre you are looking for a better, more efficient, and more powerful way of building front-end web applications? Well, look no further, you have come to the right place!This book comprehensively integrates Angular version 4 into your tool belt, then runs you through all the new options you now have on hand for your web apps without bogging you down. The frameworks, tools, and libraries mentioned here will make your work productive and minimize the friction usually associated with building server-side web applications.Starting off with building blocks of Angular version 4, we gradually move into integrating TypeScript and ES6. You will get confident in building single page applications and using Angular for prototyping components. You will then move on to building web services and full-stack web application using ASP.NET WebAPI. Finally, you will learn the development process focused on rapid delivery and testability for all application layers.Style and approachThis book covers everything there is to know about getting well-acquainted with Angular 4 and.NET without bogging you down. Everything is neatly laid out under clear headings for quick consultation, offering you the information required to understand a concept immediately, with short, relevant examples of each feature.
Frequently asked questions
Information
Angular Building Blocks - Part 1
- Modules
- Components
- Decorators and metadata
- Templates
- Bindings
- Directives
- Dependency injection
Modules (NgModules)
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
@NgModule({
imports: [ BrowserModule ],
providers: [ Logger ],
declarations: [ AppComponent ],
exports: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }

export class AppComponent { }
import {AppComponent} from './app.component';
import {Component} from '@angular/core';
Components
Class FirstComponent {
}

import { Component } from '@angular/core';
@Component({
selector: 'first-component',
template: `<h1>{{getGreetingPhrase()}} {{name}}</h1>`,
})
export class FirstComponent {
name: string;
constructor() {
this.name = 'Rajesh Gunasundaram';
}
getGreetingPhrase() {
return 'Hello Author,';
}
}