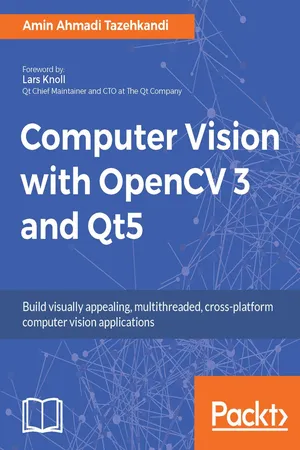
Computer Vision with OpenCV 3 and Qt5
Amin Ahmadi Tazehkandi, Vinícius G. Mendonça, Karl Phillip Buhr
- 448 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Computer Vision with OpenCV 3 and Qt5
Amin Ahmadi Tazehkandi, Vinícius G. Mendonça, Karl Phillip Buhr
About This Book
Blend the power of Qt with OpenCV to build cross-platform computer vision applications
Key Features
- ? Start creating robust applications with the power of OpenCV and Qt combined
- ? Learn from scratch how to develop cross-platform computer vision applications
- ? Accentuate your OpenCV applications by developing them with Qt
Book Description
Developers have been using OpenCV library to develop computer vision applications for a long time. However, they now need a more effective tool to get the job done and in a much better and modern way. Qt is one of the major frameworks available for this task at the moment.
This book will teach you to develop applications with the combination of OpenCV 3 and Qt5, and how to create cross-platform computer vision applications. We'll begin by introducing Qt, its IDE, and its SDK. Next you'll learn how to use the OpenCV API to integrate both tools, and see how to configure Qt to use OpenCV. You'll go on to build a full-fledged computer vision application throughout the book.
Later, you'll create a stunning UI application using the Qt widgets technology, where you'll display the images after they are processed in an efficient way. At the end of the book, you'll learn how to convert OpenCV Mat to Qt QImage. You'll also see how to efficiently process images to filter them, transform them, detect or track objects as well as analyze video. You'll become better at developing OpenCV applications.
What you will learn
- ? Get an introduction to Qt IDE and SDK
- ? Be introduced to OpenCV and see how to communicate between OpenCV and Qt
- ? Understand how to create UI using Qt Widgets
- ? Learn to develop cross-platform applications using OpenCV 3 and Qt 5
- ? Explore the multithreaded application development features of Qt5
- ? Improve OpenCV 3 application development using Qt5
- ? Build, test, and deploy Qt and OpenCV apps, either dynamically or statically
- ? See Computer Vision technologies such as filtering and transformation of images, detecting and matching objects, template matching, object tracking, video and motion analysis, and much more
- ? Be introduced to QML and Qt Quick for iOS and Android application development
Who this book is for
This book is for readers interested in building computer vision applications. Intermediate knowledge of C++ programming is expected. Even though no knowledge of Qt5 and OpenCV 3 is assumed, if you're familiar with these frameworks, you'll benefit.
Frequently asked questions
Information
Creating a Comprehensive Qt+OpenCV Project
- Structure of a Qt project and Qt build process
- Design patterns in Qt and OpenCV
- Styling in Qt applications
- Languages in Qt applications
- How to use Qt Linguist tool
- How to Create and Use plugins in Qt
Behind the scenes
Hello_Qt_OpenCV.pro Hello_Qt_OpenCV.pro.user main.cpp mainwindow.cpp mainwindow.h mainwindow.ui
The qmake tool
QT += core gui
TARGET = Hello_Qt_OpenCV TEMPLATE = app
SOURCES += \ main.cpp \ mainwindow.cpp HEADERS += \ mainwindow.h FORMS += \ mainwindow.ui
win32: { include("c:/dev/opencv/opencv.pri") } unix: !macx{ CONFIG += link_pkgconfig PKGCONFIG += opencv } unix: macx{ INCLUDEPATH += "/usr/local/include" LIBS += -L"/usr/local/lib" \ -lopencv_world }
#include "mainwindow.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow w; w.show(); return a.exec(); }
QApplication a(argc, argv); MainWindow w; w.show(); return a.exec();