
Design Patterns and Best Practices in Java
A comprehensive guide to building smart and reusable code in Java
Kamalmeet Singh, Adrian Ianculescu, LUCIAN-PAUL TORJE
- 280 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Design Patterns and Best Practices in Java
A comprehensive guide to building smart and reusable code in Java
Kamalmeet Singh, Adrian Ianculescu, LUCIAN-PAUL TORJE
About This Book
Create various design patterns to master the art of solving problems using Java
Key Features
- This book demonstrates the shift from OOP to functional programming and covers reactive and functional patterns in a clear and step-by-step manner
- All the design patterns come with a practical use case as part of the explanation, which will improve your productivity
- Tackle all kinds of performance-related issues and streamline your development
Book Description
Having a knowledge of design patterns enables you, as a developer, to improve your code base, promote code reuse, and make the architecture more robust. As languages evolve, new features take time to fully understand before they are adopted en masse. The mission of this book is to ease the adoption of the latest trends and provide good practices for programmers.
We focus on showing you the practical aspects of smarter coding in Java. We'll start off by going over object-oriented (OOP) and functional programming (FP) paradigms, moving on to describe the most frequently used design patterns in their classical format and explain how Java's functional programming features are changing them.
You will learn to enhance implementations by mixing OOP and FP, and finally get to know about the reactive programming model, where FP and OOP are used in conjunction with a view to writing better code. Gradually, the book will show you the latest trends in architecture, moving from MVC to microservices and serverless architecture. We will finish off by highlighting the new Java features and best practices. By the end of the book, you will be able to efficiently address common problems faced while developing applications and be comfortable working on scalable and maintainable projects of any size.
What you will learn
- Understand the OOP and FP paradigms
- Explore the traditional Java design patterns
- Get to know the new functional features of Java
- See how design patterns are changed and affected by the new features
- Discover what reactive programming is and why is it the natural augmentation of FP
- Work with reactive design patterns and find the best ways to solve common problems using them
- See the latest trends in architecture and the shift from MVC to serverless applications
- Use best practices when working with the new features
Who this book is for
This book is for those who are familiar with Java development and want to be in the driver's seat when it comes to modern development techniques. Basic OOP Java programming experience and elementary familiarity with Java is expected.
Frequently asked questions
Information
Functional Patterns
Introducing functional programming
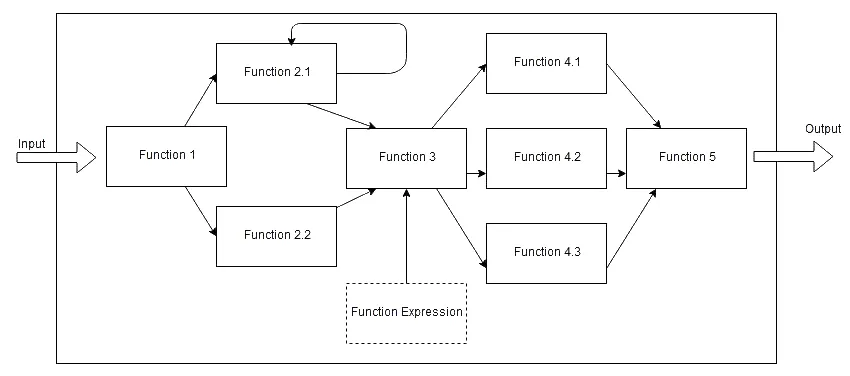
- Lambda expressions
- Pure functions
- Referential transparency
- First-class functions
- Higher-order functions
- Function composition
- Currying
- Closure
- Immutability
- Functors
- Applicatives
- Monads
Lambda expressions




Pure functions
- Writing to a file/console/network/screen
- Modifying an outside variable/state
- Calling a non-pure function
- Starting a process
Referential transparency
First-class functions
Higher-order functions
jshell> IntStream.of(70, 75, 80, 90).map(x -> (x - 32)*5/9).average();
$4 ==> OptionalDouble[25.5]