
Beginning React
Simplify your frontend development workflow and enhance the user experience of your applications with React
Andrea Chiarelli
- 96 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Beginning React
Simplify your frontend development workflow and enhance the user experience of your applications with React
Andrea Chiarelli
About This Book
Take your web applications to a whole new level with efficient, component-based UIs that deliver cutting-edge interactivity and performance.
Key Features
- Elaborately explains basics before introducing advanced topics
- Explains creating and managing the state of components across applications
- Implement over 15 practical activities and exercises across 11 topics to reinforce your learning
Book Description
Projects like Angular and React are rapidly changing how development teams build and deploy web applications to production. In this book, you'll learn the basics you need to get up and running with React and tackle real-world projects and challenges. It includes helpful guidance on how to consider key user requirements within the development process, and also shows you how to work with advanced concepts such as state management, data-binding, routing, and the popular component markup that is JSX. As you complete the included examples, you'll find yourself well-equipped to move onto a real-world personal or professional frontend project.
What you will learn
- Understand how React works within a wider application stack
- Analyze how you can break down a standard interface into specific components
- Successfully create your own increasingly complex React components with HTML or JSX
- Correctly handle multiple user events and their impact on overall application state
- Understand the component lifecycle to optimize the UX of your application
- Configure routing to allow effortless, intuitive navigation through your components
Who this book is for
If you are a frontend developer who wants to create truly reactive user interfaces in JavaScript, then this is the book for you. For React, you'll need a solid foundation in the essentials of the JavaScript language, including new OOP features that were introduced in ES2015. An understanding of HTML and CSS is assumed, and a basic knowledge of Node.js will be useful in the context of managing a development workflow, but is not essential.
Frequently asked questions
Information
Managing User Interactivity
- Handle events generated by user interaction
- Change a component's state on event triggering
- Use a component's lifecycle events for a better user experience
- Configure routing to allow navigation through components
Managing User Interaction
[
{"code":"P01",
"name": "Traditional Merlot",
"description": "A bottle of middle weight wine, lower in tannins
(smoother), with a more red-fruited flavor profile.",
"price": 4.5, "selected": false},
{"code":"P02",
"name": "Classic Chianti",
"description": "A medium-bodied wine characterized by a marvelous
freshness with a lingering, fruity finish",
"price": 5.3, "selected": false},
{"code":"P03",
"name": "Chardonnay",
"description": "A dry full-bodied white wine with spicy,
bourbon-y notes in an elegant bottle",
"price": 4.0, "selected": false},
{"code":"P04",
"name": "Brunello di Montalcino",
"description": "A bottle of red wine with exceptionally bold fruit
flavors, high tannin, and high acidity",
"price": 7.5, "selected": false}
]
import React from 'react';
class Product extends React.Component {
showPrice() {
alert(this.props.item.price);
}
render() {
return <li onClick={() => this.showPrice()}>
<h3>{this.props.item.name}</h3>
<p>{this.props.item.description}</p>
</li>;
}
}
export default Product;
- Open a console window
- Move to the my-shop-01 folder
- Run npm install
- Run npm start
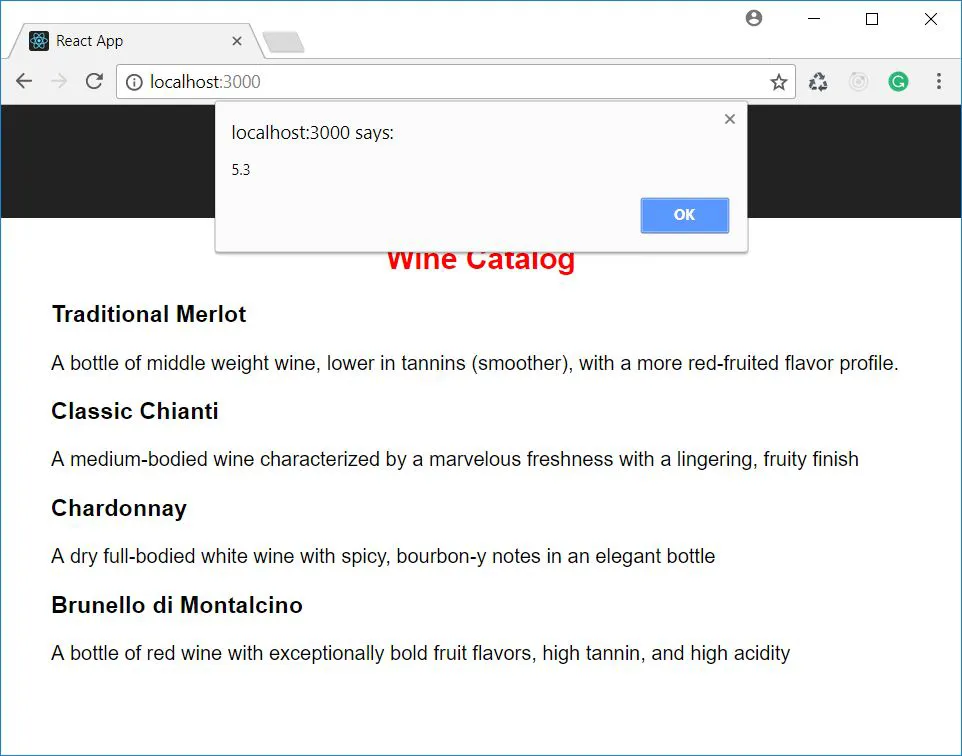
HTML Events versus React Events
<li onclick="...">...</li>
<li onClick=...>...</li>
<li onclick="showPrice()">...</li>
<li onClick={showPrice}>...</li>
<li onClick={() => this.showPrice()}>
<a href="#" onClick={(e) => { e.preventDefault();
console.log("Clicked");}}>Click</a>
Event Handlers and the this Keyword
- Use an arrow function and invoke the method inside its body, as shown in the following example:
<li onClick={() => this.showPrice()}>
- Use the bind() method to bind the method to the current class context, as shown in the following example:
<li onClick={this.showPrice.bind(this)}>
- You can use bind() in the class constructor instead of using it inline when assigning the method to the event attribute. The following is an example of this approach:
constructor() {
this.showPrice = this.showPrice.bind(this);
}
...
<li onClick={this.showPrice}>
Changing the State
[
{"code":"P01",
"name": "Traditional Merlot",
"description": "A bottle of middle weight wine, lower in tannins
(smoother), with a more red-fruited flavor profile.",
"price": 4.5, "selected": false},
{"code":"P02",
"name": "Classic Chianti",
"description": "A medium-bodied wine characterized by a marvelous
freshness with a lingering, fruity finish",
"price": 5.3, "selected": false},
{"code":"P03",
"name": "Chardonnay",
"description": "A dry full-bodied white wine...