
Learning Concurrency in Kotlin
Build highly efficient and robust applications
Miguel Angel Castiblanco Torres
- 266 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Learning Concurrency in Kotlin
Build highly efficient and robust applications
Miguel Angel Castiblanco Torres
About This Book
Take advantage of Kotlin's concurrency primitives to write efficient multithreaded applications
Key Features
- Learn Kotlin's unique approach to multithreading
- Work through practical examples that will help you write concurrent non-blocking code
- Improve the overall execution speed in multiprocessor and multicore systems
Book Description
The primary requirements of modern-day applications are scalability, speed, and making the most use of hardware. Kotlin meets these requirements with its immense support for concurrency. Many concurrent primitives of Kotlin, such as channels and suspending functions, are designed to be non-blocking and efficient. This allows for new approaches to concurrency and creates unique challenges for the design and implementation of concurrent code. Learning Concurrency in Kotlin addresses those challenges with real-life examples and exercises that take advantage of Kotlin's primitives. Beginning with an introduction to Kotlin's coroutines, you will learn how to write concurrent code and understand the fundamental concepts needed to be able to write multithreaded software in Kotlin. You'll explore how to communicate between and synchronize your threads and coroutines to write asynchronous applications that are collaborative. You'll also learn how to handle errors and exceptions, as well as how to leverage multi-core processing. In addition to this, you'll delve into how coroutines work internally, allowing you to see the bigger picture. Throughout the book you'll build an Android application â an RSS reader â designed and implemented according to the different topics covered in the book
What you will learn
- Understand Kotlin's approach to concurrency
- Implement sequential and asynchronous suspending functions
- Create suspending data sources that are resumed on demand
- Explore the best practices for error handling
- Use channels to communicate between coroutines
- Uncover how coroutines work under the hood
Who this book is for
If you're a Kotlin or Android developer interested in learning how to program concurrently to enhance the performance of your applications, this is the book for you.
Frequently asked questions
Information
Suspending Functions and the Coroutine Context
- What a suspending function is
- How to use suspending functions
- When to use async functions instead of suspending functions
- What the coroutine context is
- Different types of contexts such as dispatcher, exception handlers, and non-cancellables
- Combining and separating contexts to define the behavior of coroutines
Improving the UI of the RSS Reader
Giving each feed a name
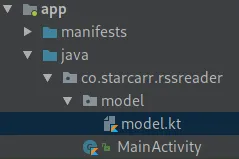
package co.starcarr.rssreader.model
data class Feed(
val name: String,
val url: String
)
private val feeds = listOf(
Feed("npr", "https://www.npr.org/rss/rss.php?id=1001"),
Feed("cnn", "http://rss.cnn.com/rss/cnn_topstories.rss"),
Feed("fox", "http://feeds.foxnews.com/foxnews/latest?format=xml"),
Feed("inv", "htt:myNewsFeed")
)
private fun asyncFetchHeadlines(feed: Feed,
dispatcher: CoroutineDispatcher) = async(dispatcher) {
val builder = factory.newDocumentBuilder()
val xml = builder.parse(feed.url)
...
}
Fetching more information about the articles from the feed
package co.starcarr.rssreader.model
data class Feed(
val name: String,
val url: String
)
data class Article(
val feed: String,
val title: String,
val summary: String
)